Given time in 24-hour format, convert it to words.
For example:
13:00 = one o'clock
13:09 = nine minutes past one
13:15 = quarter past one
13:29 = twenty nine minutes past one
13:30 = half past one
13:31 = twenty nine minutes to two
13:45 = quarter to two
00:48 = twelve minutes to one
00:08 = eight minutes past midnight
12:00 = twelve o'clock
00:00 = midnight
Note: If minutes == 0, use 'o'clock'. If minutes <= 30, use 'past', and for minutes > 30, use 'to'.
Good luck!
Tests:
07:01
01:59
12:01
00:01
11:31
23:31
This challenge comes from KenKamau on CodeWars. Thank you to CodeWars, who has licensed redistribution of this challenge under the 2-Clause BSD License!
Want to propose a challenge idea for a future post? Email yo+challenge@dev.to with your suggestions!
Top comments (1)
A little big but does the job
Output
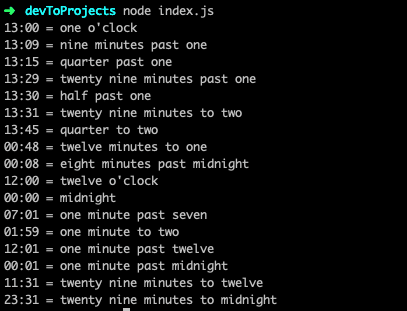