In this article, I will add questions about JavaScript Classes. Let's begin:
-
This one is about converting constructor function to class. It is a beneficial coding exercise to understand JavaScript class knowledge.
// Rewrite the following consturctor function as a Class function Animal(name) { this.name = name; } Animal.prototype.duck = function () { console.log(this.name, 'duck'); }; const animal = new Animal('daffy'); animal.duck();
The answer will be below:
// The answer is below class Animal { constructor(name) { this.name = name; } duck() { console.log(this.name, 'duck'); } } const animal = new Animal('daffy'); // new keyword is mandatory animal.duck();
And one more related question after converting to class.
// 3. What will be the following console output? console.log(typeof Animal);
The answer is:
function
You can test it in below:
-
This one is about differences in declaration of class and functions.
// 1. What will be the output? const car = new Car('Clio'); function Car(model) { this.model = model; } Car.prototype.getModel = function () { return this.model; }; console.log('car model:', car.getModel()); // 2. What will be the output? const bike = new Bike('Bianchi'); class Bike { constructor(model) { this.model = model; } getModel() { return this.model; } } console.log('bike model:', bike.getModel());
The console output will be below:
car model: Clio ReferenceError: Bike is not defined
function definitions are hoisted so we can create an instance before declaration. However, class definitions are not initialized until their definition is evaluated so it gives
Bike is not defined
error. You can read This stackoverflow answer for more detailed information.You can test it in below:
-
Next question is about inheritance in JavaScript classes.
// 1. What will be the console outputs? class Parent { constructor(name) { this.name = name; } getName() { return this.name; } } class Child extends Parent { constructor(name) { super(name); } getMessage() { return `My name is ${super.getName()}`; } } const child = new Child('Halil'); console.log(child.getMessage()); console.log(child.getName());
The outputs will be as below:
My name is Halil Halil
So, although there is no
getName()
method inChild
class it is inherited fromParent
class.You can test it below:
-
Write a generator function which takes an array and returns its every member in each call.
The answer can be as below:
function* sendNumber(list) { for (let item of list) { yield item; } } const iterator = sendNumber([1, 2, 3]); // What will be written? console.log(iterator.next()); // What will be written? console.log(iterator.next()); // What will be written? console.log(iterator.next()); // What will be written? console.log(iterator.next());
And the console outputs will be:
{ value: 1, done: false } { value: 2, done: false } { value: 3, done: false } { value: undefined, done: true }
You can test it in below:
You can read the previous articles of this series from the links below:
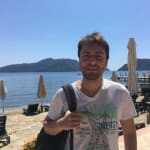
JavaScript Interview Coding Questions — 1
Halil Can Ozcelik ・ Jan 4 '20 ・ 2 min read
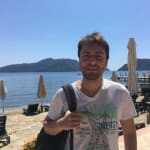
JavaScript Interview Coding Questions — 2
Halil Can Ozcelik ・ Jan 6 '20 ・ 2 min read
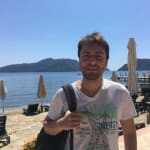
Top comments (0)