Let’s continue to coding assessment questions.
-
This one is about testing hoisting knowledge in JavaScript.
// What will be the console outputs? Why? callMe1(); callMe2(); function callMe1() { console.log('I am here'); } var callMe2 = function () { console.log('I am here too'); };
You will get an error for
callMe2()
. Console log will be below:
I am here TypeError: callMe2 is not a function
In JavaScript, basically function and variable definitions are moved to top (which is named as hoisting). The
callMe2
variable is defined but it is not a function! This is because, hoisting only moves up to declarations not initializations. So, ourcallMe2
variable is declared but the function is not assigned to it.You can test it in below:
-
This one is for testing the knowledge about
const
,let
andvar
.
// 1. What will be output? var v; let l; const c; console.log({ v, l, c });
Again you will get an error.
const c; ^ SyntaxError: Missing initializer in const declaration
You must assign a value while declaring a
const
variable!You can test it in below:
-
Another question about variable declarations.
// 2. What will be outputs? Try one by one! console.log('a:', a); var a = 1; console.log('b:', b); let b = 2; console.log('c:', c); const c = 3;
If you try them one by one you will see the following console logs:
a: undefined ReferenceError: b is not defined ReferenceError: c is not defined
This is because variables which are declared with
let
orconst
are not initialized until their definition is evaluated! So it will throw ReferenceError. You can read Temporal Dead Zone part for more detailed information.You can test it in below:
My some other articles:
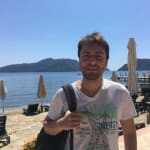
JavaScript Interview Coding Questions — 1
Halil Can Ozcelik ・ Jan 4 '20 ・ 2 min read
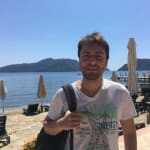
JavaScript Interview Coding Questions - 3
Halil Can Ozcelik ・ Jan 7 '20 ・ 3 min read
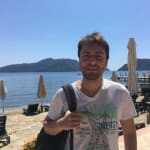
Top comments (2)
interesting.
thanks :3