I am trying to explain some possible coding questions in software developer interviews. I will mention recursion and array mutation in this third article. These two topics are important in functional programming paradigm. Also, the last example is about prototypal inheritance which is crucial to understand inheritance in JavaScript.
-
Write a recursive function to calculate the total of numbers between 1 to n?
n
will be the parameter of our function. So we should call this calculator function until reaching to1
which is our end point. So, one of the possible effective solutions will be below code:
function calculateTotal(number, total = 1) { return number > 1 ? calculateTotal(number - 1, total + number) : total; } console.log(calculateTotal(10));
You can examine the code in below:
-
Write a recursive factorial calculator function.
We can easily adapt same logic to factorial calculation as below:
function factorial(number, product = 1) { return number > 0 ? factorial(number - 1, product * number) : product; } console.log(factorial(5));
!! The recursive functions above will cause stack overflow error for large inputs. In order to prevent it, Trampoline Pattern can be used as below:
// recursive optimization to prevent stack overflow error function trampoline(fn) { return (...args) => { let result = fn(...args); while (typeof result === 'function') { result = result(); } return result; }; } // Write a recursive function to calculate the total of numbers between 1 to n? function calculateTotal(number, total = 1) { return number > 1 ? () => calculateTotal(number - 1, total + number) : total; } const totalCalculator = trampoline(calculateTotal); console.log(totalCalculator(100000)); // Write a recursive factorial calculator function function factorial(number, product = 1) { return number > 0 ? () => factorial(number - 1, product * number) : product; } const factorialCalculator = trampoline(factorial); console.log(factorialCalculator(100));
You can examine the code in below:
-
This one is about mutator methods in JavaScript arrays. Immutability of variables is an important topic in functional programming.
var arr = [1, 2, 3, 7, 4]; // Which of the followings will mutate variables? // Find a functional alternative for mutator ones. arr.push(5); => mutator arr.shift(); => mutator arr.concat(6, 7); => non-mutator arr.map(a => a * a); => non-mutator arr.sort(); => mutator
And these can be alternative solutions for mutator ones.
var arr = [1, 2, 3, 7, 4]; // Which of the followings will mutate variables? // Find a functional alternative for mutator ones. arr.push(5); => arr.concat(5); arr.shift(); => arr.slice(1); arr.concat(6, 7); => non-mutator arr.map(a => a * a); => non-mutator arr.sort(); => arr.concat().sort()
You can examine the code in below:
-
This one is to examine your understanding about Prototypal Inheritance.
function Person() {} // 1st definition for 'sayHi' method Person.prototype.sayHi = function () { console.log('Hi!'); }; var person = new Person(); // What will be the printed message? person.sayHi(); // 2nd definition for 'sayHi' method Person.prototype.sayHi = function () { console.log('Hello!'); }; // What will be the printed message? person.sayHi(); // What will be returned? person.hasOwnProperty('sayHi');
The output will be below:
Hi! Hello! false
person
object doesn't have ownsayHi()
method becausePerson
function doesn't have any method. When we instantiate an object withnew
keyword, it inherits allprototype
methods of the function as its__proto__
property. So, in first execution ofsayHi()
the defined one is loggingHi!
so it is executed. But after second definition ofsayHi()
the newer one will be called. Because,person.sayHi()
points to the same function due to prototypal inheritance. Finally,person.hasOwnProperty('sayHi')
returnsfalse
because this is not a property ofperson
object, it is inherited by prototype chain.You can examine the code in below:
My some other articles:
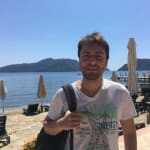
JavaScript Interview Coding Questions — 1
Halil Can Ozcelik ・ Jan 4 '20 ・ 2 min read
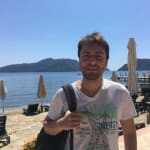
Top comments (2)
Question 1 can be very inefficient, and you'll exceed the call stack size if you enter a big enough number.
The trampoline pattern is a more efficient way to do recursion.
blog.logrocket.com/using-trampolin...
Great! I am adding it to article. Thanks.