Let's create a VCard with JS
Code
HTML
<fieldset>
<div>
<label for="cardInfoEl">cardInfo</label>
<input type="text" id="cardInfoEl" />
</div>
<div>
<label for="emailEl">email</label>
<input type="text" id="emailEl" />
</div>
<div>
<label for="nameEl">name</label>
<input type="text" id="nameEl" />
</div>
<div>
<label for="orgEl">org</label>
<input type="text" id="orgEl" />
</div>
<div>
<label for="titleEl">title</label>
<input type="text" id="titleEl" />
</div>
<div>
<label for="telEl">tel</label>
<input type="text" id="telEl" />
</div>
<div>
<label for="addressEl">address</label>
<input type="text" id="addressEl" />
</div>
<div>
<label for="fileEl">Clique aqui para carregar imagem</label>
<input type="file" id="fileEl" hidden /><br />
<img width="100" alt="PrΓ©via da imagem..." id="previewEl" />
</div>
<button id="downloadEl">Save</button>
</fieldset>
The form includes fields for entering information such as name, email address, organization, title, phone number and address, as well as an image that can be uploaded using a "Click here to upload image" button.
All input elements are labeled and have a unique "id" that can be used to reference them in other code (eg in JavaScript). The "Save" button at the end of the form allows the information to be saved.
JS
'use strict';
function downloadToFile(content, filename, contentType) {
const a = document.createElement('a');
const file = new Blob([content], { type: contentType });
a.href = URL.createObjectURL(file);
a.download = filename;
a.click();
URL.revokeObjectURL(a.href);
}
function previewFile(event) {
let reader = new FileReader();
let file = event.target.files[0];
reader.readAsDataURL(file);
reader.onloadend = () => (previewEl.src = reader.result);
}
const makeVCardVersion = () => `VERSION:3.0`;
const makeVCardInfo = (info) => `N:${info}`;
const makeVCardName = (name) => `FN:${name}`;
const makeVCardOrg = (org) => `ORG:${org}`;
const makeVCardTitle = (title) => `TITLE:${title}`;
const makeVCardPhoto = (img) => `PHOTO;TYPE=JPEG;ENCODING=b:[${img}]`;
const makeVCardTel = (phone) => `TEL;TYPE=WORK,VOICE:${phone}`;
const makeVCardAdr = (address) => `ADR;TYPE=WORK,PREF:;;${address}`;
const makeVCardEmail = (email) => `EMAIL:${email}`;
const makeVCardTimeStamp = () => `REV:${new Date().toISOString()}`;
function makeVCard() {
let vcard = `BEGIN:VCARD
${makeVCardVersion()}
${makeVCardInfo(cardInfoEl.value)}
${makeVCardName(nameEl.value)}
${makeVCardOrg(orgEl.value)}
${makeVCardTitle(titleEl.value)}
${makeVCardPhoto(previewEl.src)}
${makeVCardTel(telEl.value)}
${makeVCardAdr(addressEl.value)}
${makeVCardEmail(emailEl.value)}
${makeVCardTimeStamp()}
END:VCARD`;
downloadToFile(vcard, 'vcard.vcf', 'text/vcard');
}
downloadEl.addEventListener('click', makeVCard);
fileEl.addEventListener('change', previewFile);
This code is a JavaScript script for generating and downloading a vCard file. A vCard is a file format standard for electronic business cards and is commonly used for exchanging contact information.
The script begins with a function, downloadToFile, which is used to download the generated vCard file. The function takes three arguments: content, filename, and contentType. The content argument is the vCard information to be downloaded, filename is the name of the file to be downloaded, and contentType is the type of content, which in this case is text/vcard.
Next, there is a function, previewFile, that is used to preview the selected image file. The function listens for a change event on the file input element, reads the selected file as a data URL, and sets the src attribute of the previewEl image element to the result of the file reader.
There are then a series of makeVCard functions, each of which generates a specific line of the vCard file. These functions take arguments and return strings in a vCard format. For example, makeVCardName takes a name argument and returns a string in the format FN:name.
Finally, the makeVCard function is defined, which combines all of the vCard information and generates the vCard file. The function sets the value of the vCard file by calling each of the makeVCard functions with the appropriate arguments, and then passes the resulting vCard content to the downloadToFile function to be downloaded.
The script ends with two event listeners, one for the download button and one for the file input element. The download button event listener calls the makeVCard function when the button is clicked, and the file input element event listener calls the previewFile function when a file is selected
Demo
See below for the complete working project.
π‘ NOTE: If you can't see it click here and see the final result
Thanks for reading!
If you have any questions, complaints or tips, you can leave them here in the comments. I will be happy to answer!
ππ See you later! ππ
Support Me
Youtube - WalterNascimentoBarroso
Github - WalterNascimentoBarroso
Codepen - WalterNascimentoBarroso
Top comments (1)
Hello, is there a javascript library that we need to add to the head section to this vcard? The card seems to be corrupt on Mozilla and Chrome esp on mobile phone and wont install.
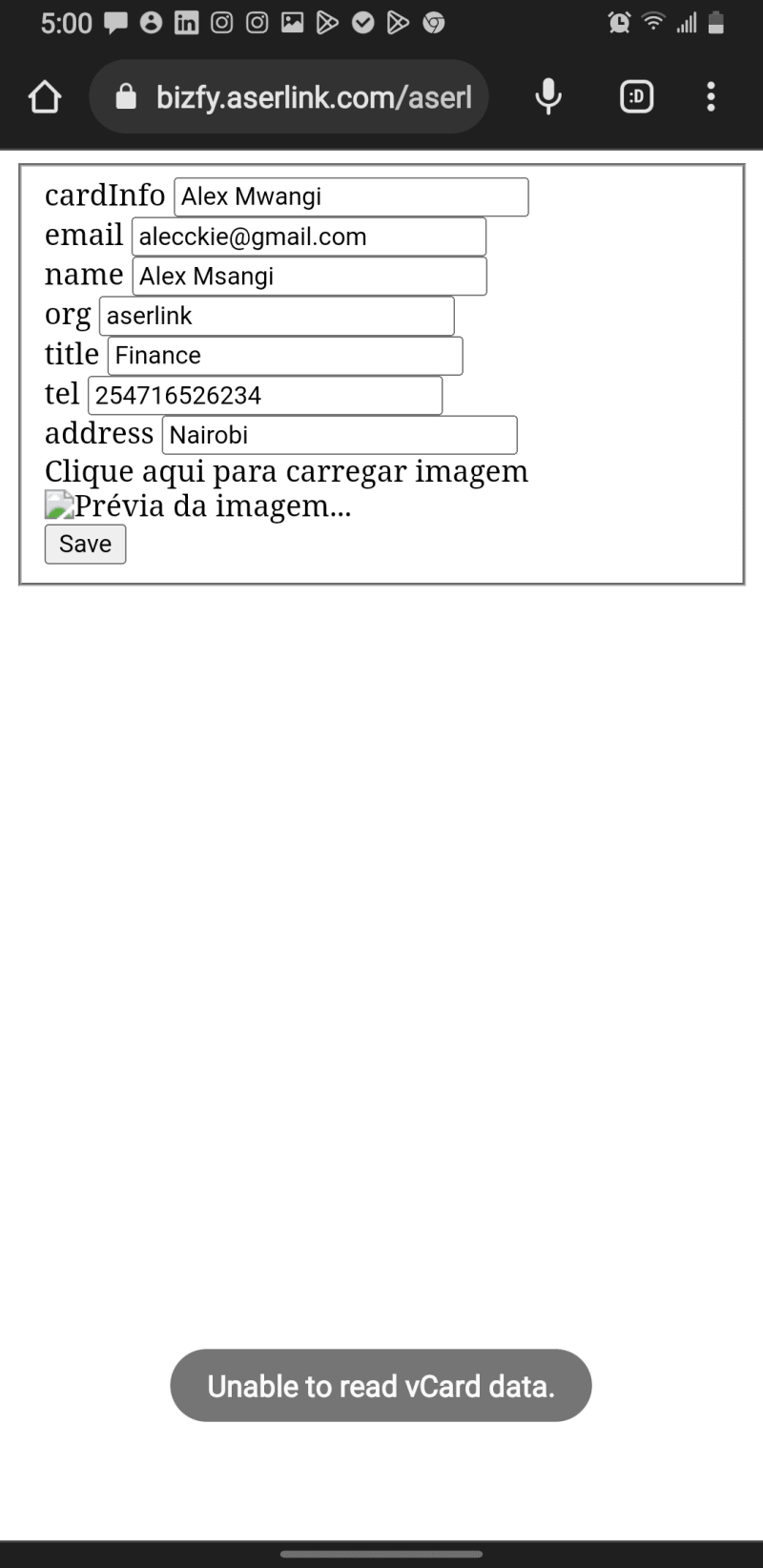