Cover Photo by Ken Suarez on Unsplash
Contents
- Short short Intro
- 1. Run command prompt in the currently focused folder
- 2. Run command prompt followed by certain commands
- 3. Search on Google the currently selected text
- 4. Open applications/websites using shortcuts
- 5. Open new explorer window with the same path as the currently focused folder
- 6. Copy to clipboard the HEX color of the pixel under your cursor
- 7. Adjust the main volume using the mouse scroll wheel
- 8. Auto-complete syntaxes with Hotstrings
- Honorable mentions
- Fin
Short short Intro
In this post, we'll summarize all the macros created with AutoHotKey (AHK) in the previous parts (+extras). However, there is no need to read those parts as I'll provide a fast and short step by step guide on how to set AHK.
Let's get started!
1. Run command prompt in the currently focused folder
If you don't have AutoHotKey installed, simply download it from here, then run the setup. Now we just need to create a file with .ahk
extension (eg. myMacros.ahk
). After you run this file (double click it), a small green H icon will pop up letting you know that the script is running in the background. Now, let's open the .ahk
file with any text editor and add these lines:
^!u:: ; Use ctrl+alt+u to open cmd in current selected folder
{
Send, !d
Send, ^c
Sleep 50
Run cmd, %clipboard%
Return
}
Save this file, and click on Reload This Script
from the AHK icon. Now, open any folder, and press ctrl+alt+u
to open cmd prompt with the folder's current path:
We can also keep in mind the key notations in AHK for Control
, Shift
, Windows
, and Alt
:
Key notation in AHK | Actual Key |
---|---|
^ | Control |
+ | Shift |
! | Alt |
# | Windows Key |
2. Run command prompt followed by certain commands
From now on we will reopen myMacros.ahk
with a text editor, and we'll just keep adding more macros, save the file, then click on Reload this Script
.
We can add more commands after opening the command prompt. For example, we can run git status
right after (or npm start
, python ./manage.py runserver
etc):
^!+u:: ; Use ctrl+alt+shift+u to open cmd in current selected folder and run git status
{
Send, !d
Send, ^c
Sleep 50
Run cmd, %clipboard%
Sleep 100
Send, git status
Sleep 100
Send, {Enter}
Return
}
Or, if you use Jupyter Notebook in a specific drive partition and/or environment, instead of opening it with command prompt, you can just use a shortcut like ctrl+alt+j
(or even ctrl+win+j
or whatever you want) and write all the corresponding commands automatically. Just add this macro:
^!j:: ; use ctrl+alt+j
{ ; to open jupyter notebook in g: in tf_gpu conda env
Run cmd, g:
Sleep 100
Send, activate tf_gpu
Sleep 1000
Send, {Enter}
Send, jupyter notebook
Sleep 2000
Send, {Enter}
Return
}
Or you can fast open Python in command prompt by pressing ctrl+alt+p
:
^!p:: ; use ctrl+alt+p
{ ; to open cmd prompt in python
Run cmd, C:\Users\MyUserName
Sleep 100
Send, python
Sleep 100
Send, {Enter}
Return
}
3. Search on Google the currently selected text
Add these lines in your myMacros.ahk
to search on Google the selected text using Ctrl+Alt+c
:
^!c:: ; use ctrl+alt+c to search on google
{
Send, ^c
Sleep 50
Run, http://www.google.com/search?q=%clipboard%
Return
}
If you have a mouse with 3 side buttons, you can even assign this macro by pressing Left Click while holding the middle left-sided mouse button (More details in my previous post):
XButton2 & LButton:: ; Search currently selected text on Google
{
Send, ^c
Sleep 100
If InStr(clipboard, "http")
Run, %clipboard%
Else
Run, https://www.google.com/search?q=%clipboard%
Return
}
; Note: if the selected text is a link, open it in a new tab
; Otherwise, search the selected text on Google
We can also search on other websites, such as Pexels and Unsplash.
^#u:: ; use ctrl+win+u to search on Unsplash
{
Send, ^c
Sleep 50
Run, https://unsplash.com/s/photos/%clipboard%
Return
}
^#p:: ; use ctrl+win+p to search on Pexels
{
Send, ^c
Sleep 50
Run, https://www.pexels.com/search/%clipboard%
Return
}
4. Open applications/websites using shortcuts
We can open any application using any shortcut key. For reference, we can use the following keys (without overwriting the system's shortcuts):
-
RAlt+(anyKeyLetter)
orRAlt+(anyNumber)
-
Ctrl+Alt+(anyKey)
(But be aware that some applications might use these key combinations, you can check using DefKey.com) Ctrl+Shift+Alt+(anyKey)
-
Alt+Shift+(anyKey)
(Check here) Ctrl+Win+(anyKey)
Ctrl+Win+Alt+(anyKey)
Here are some examples:
^#1::Run "https://mail.google.com/mail/u/0/#inbox" ; use ctrl+win+1 to open gmail 1
^#2::Run "https://mail.google.com/mail/u/1/#inbox" ; use ctrl+win+2 to open gmail 2
^#3::Run "https://translate.google.ro/?hl=ro&tab=wT" ; use ctrl+win+3 to open Google Translate
^+!1::Run "https://www.google.com" ; use ctrl+shift+alt+1
^+!2::Run "https://dev.to" ; use ctrl+shift+alt+2
^+!3::Run "https://www.linkedin.com" ; use ctrl+shift+alt+3
^+!4::Run "https://www.spotify.com/" ; use ctrl+shift+alt+4
^+!5::Run "https://www.mixcloud.com/" ; use ctrl+shift+alt+5
^+!6::Run "https://www.freecodecamp.org/learn/"
^!t::Run cmd, C:\Users\Username ; use ctrl+alt+t to run Cmd
^!w::Run winamp.exe ; use ctrl+alt+w to run Winamp
^!s::Run C:\Program Files\Sublime Text 3\sublime_text.exe
^!v::Run C:\Users\Username\AppData\Local\Programs\Microsoft VS Code\Code.exe
5. Open new explorer window with the same path as the currently focused folder
I often use Win+E
default shortcut to open the Explorer, but oftentimes I need to open a new window within the same location to move/organize some files. So why not use Ctrl+Win+E
shortcut to do that?
^#e:: ; use ctrl+win+e
{ ; to open new explorer window with the same selected folder
Send, !d
Sleep 50
Send, ^c
Sleep 100
Run, Explorer "%clipboard%"
Return
}
We can do even more by opening the new explorer and have both windows side by side, and even have the new window go up one level:
^+#e:: ; use ctrl+shift+win+e
{ ; to open new explorer window with the same selected folder
Send, #{Left}
Sleep 50
Send, !d
Sleep 50
Send, ^c
Sleep 100
Run, Explorer "%clipboard%"
Sleep 900
Send, !{Up}
Sleep 600
Send, #{Right}
Return
}
6. Copy to clipboard the HEX color of the pixel under your cursor
We can copy the HEX color under our cursor using Ctrl+Win+LeftClick
:
; Copy to clipboard the HEX color of the pixel under your cursor using CTRL+Win+LeftClick
^#LButton::
{
MouseGetPos, MouseX, MouseY
PixelGetColor, color, %MouseX%, %MouseY%, RGB
StringLower, color, color
clipboard := SubStr(color, 3)
Return
}
7. Adjust the main volume using the mouse scroll wheel
You can adjust your main volume with key combinations such as RAlt & NumpadAdd
/RAlt & NumpadSub
, or with your mouse wheel while holding Right Alt
:
RAlt & WheelUp::Volume_Up
RAlt & WheelDown::Volume_Down
Or, you can hold down one of the side buttons on your mouse while scrolling (if you have such a mouse)
XButton1 & WheelUp::Volume_Up
XButton1 & WheelDown::Volume_Down
We can also play/pause our current playing song using a left-side mouse button:
XButton1::Media_Play_Pause
We can do so much more using our side mouse buttons! You can read more about mouse macros in my previous post.
8. Auto-complete syntaxes with Hotstrings
These are not exactly keyboard shortcuts, but rather abbreviations expansions. More important, we can use any keywords that we like in order to auto-complete syntaxes such as print statements and loops, in any programming language:
:*:printc::printf('%d\n', num);
:*:logjs::
{
Send, console.log();{Left}{Left}
Return
}
:*:printjava::
{
Send, System.out.println();{Left}{Left}
Return
}
:*:writecs::
{
Send, Console.WriteLine();{Left}{Left}
Return
}
::forC::
(
for (int i = 0; i < n; i++) {
)
::forJs::
(
for (let i = 0; i < arr.length; i++) {
)
::forPy::for i in range(0, len(arr)):
:*:forMatlab::
(
for i = 1:step:length(arr)
end
)
::switchJs::
(
switch() {
case 0:
break;
case 1:
break;
default:
)
I've made a complete guide on using AutoHotKey's hotstrings here.
Honorable mentions
Put PC to sleep
We can get our PC into sleep mode by using a key shortcut like RAlt+PauseKey
:
; Put PC in sleep mode
RAlt & Pause::DllCall("PowrProf\SetSuspendState", "int", 0, "int", 0, "int", 0)
Empty recycle bin
We can empty the recycle bin by using WinKey+Del
:
#Del::FileRecycleEmpty ; use win+del to empty recycle bin
Get the current date/hour
We can use AutoHotKey's Hotstrings to write the current system's date & time:
:*:datenow::
{
FormatTime, DateString, , dddd, MMMM dd, yyyy
Send %DateString%
Return
}
:*:timenow::
{
FormatTime, DateString, , HH:mm
Send %DateString%
Return
}
:*:datetoday::
{
FormatTime, DateString, , yyyy-MM-dd
Send %DateString%
Return
}
:*:datetmr::
{
Date += 1, Days
FormatTime, DateString, %Date%, yyyy-MM-dd
Send %DateString%
Reload ; Return and clear variables
}
Use arrow keys as Home/End while holding RAlt
If you like cutting, pasting, and selecting lots of text. These "macros" might be handy:
; Arrow keys as Home/End
RAlt & Left::
{
If GetKeyState("Shift", "P")
Send +{Home}
Else
Send {Home}
Return
}
RAlt & Right::
{
If GetKeyState("Shift", "P")
Send +{End}
Else
Send {End}
Return
}
Disable CapsLock key
Well, if you find yourself accidentally switching the CapsLock state from time to time... you can actually disable it completely. At the very first beginning of the .ahk
file, just add the following:
; Set Lock Keys permanently off
SetCapsLockState, AlwaysOff
Return
This is especially useful if we are using 60% keyboards. However, if you need to use CapsLock now and then, you can make it a little harder to toggle it's state, by pressing Alt+CapsLock
instead:
; Set Lock Keys permanently off by default
SetCapsLockState, AlwaysOff
Return
Alt & CapsLock::
If GetKeyState("CapsLock","T")
SetCapsLockState, AlwaysOff
Else
SetCapsLockState, On
Return
You can find more on AHK's GetKeyState()
here.
Fin
Mountains Photo by Benjamin Voros on Unsplash
That was it! I'm hoping that you found some of these macros actually useful in your day to day life... or maybe you got some other ideas for your workflow.
Have a wonderful day,
R.B.
Top comments (3)
Hence, several Best Custom Writing and universities provide with guidelines which apart from mentioning the word limit, also mentions the font type, size and color.
Here there are two important questions that may occur. One: can you exceed with the provided limit bar? A thorough instructions column must be read before making any decision. This is because of some reasons.
This article is worth it for #6 alone; no more color picker browser extensions for me!
Hello, glad you enjoyed this article.
However, meanwhile, I found another method to pick up and even edit colors on the fly -> If you're using Windows 10, you can download and install Microsoft Powertoys, then hit WIN+SHIFT+C keys to pick a color on your screen!
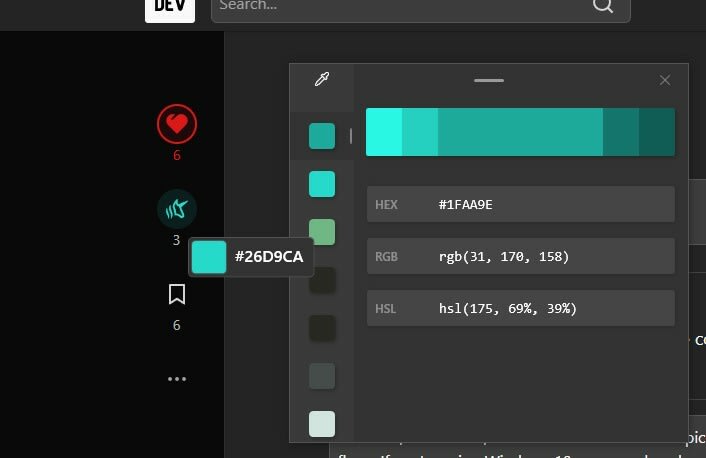