Liquid syntax error: 'raw' tag was never closed
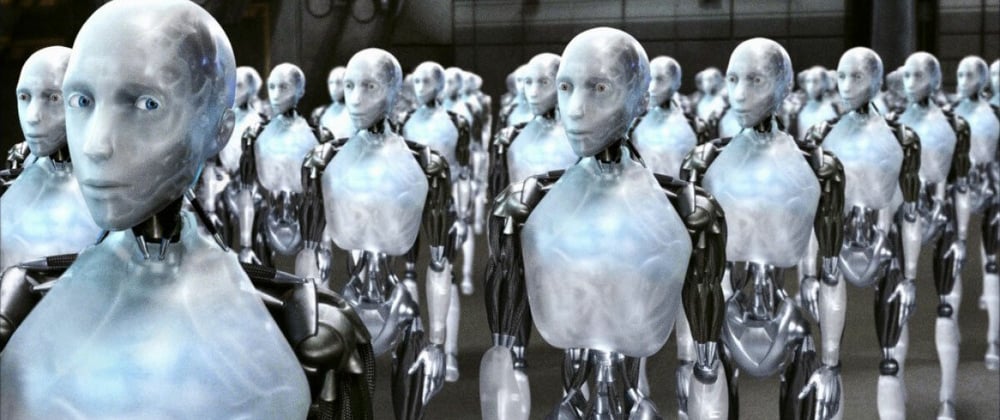
For further actions, you may consider blocking this person and/or reporting abuse
Liquid syntax error: 'raw' tag was never closed
For further actions, you may consider blocking this person and/or reporting abuse
Developer Service -
Lucy Linder -
Kazuhiro "Kaz" Sera -
Thomas Epelbaum -
Top comments (0)