Hold on, aspiring React JS devs! It's important to comprehend a few basic JavaScript ideas before delving into the world of virtual components and state management. Consider it as creating a solid base upon which to build your masterpiece in React. Ignoring these fundamentals might cause confusion, irritation, and eventually a subpar development experience. So saddle up and get ready to learn these fundamentals of JavaScript before starting your React trip!
1. JavaScript Fundamentals: The Bedrock of Your Code
- Variables & Data Types:
Discover how to use appropriate variable declarations and data types to store and manipulate data, including strings, integers, and objects. Gain an understanding of the scope and the behaviour of variables in various blocks and functions.
- Control Flow & Functions:
Learn how to create reusable functions that take parameters, carry out specified actions, and return values. To manage program flow, comprehend control flow statements like if-else, switch statements, and loops (for, while, etc.).
- Operators & Expressions:
Math is not a scary thing! Discover how to use both simple and complex operators, such as assignment, logical, comparison, and arithmetic operators. Recognize how expressions operate by putting operands and operators together to create values.
// Get the canvas element
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Draw a rectangle
ctx.fillStyle = 'red';
ctx.fillRect(10, 10, 100, 50);
// Draw a circle
ctx.beginPath();
ctx.arc(150, 50, 20, 0, 2 * Math.PI);
ctx.fillStyle = 'blue';
ctx.fill();
// Draw a line
ctx.strokeStyle = 'green';
ctx.lineWidth = 5;
ctx.beginPath();
ctx.moveTo(50, 150);
ctx.lineTo(200, 150);
ctx.stroke();
2. Object-Oriented Programming: Building Blocks of Complex Apps
- Classes & Objects:
Learn how to construct and utilize classes and objects to get started with object-oriented programming (OOP). Acquire knowledge of constructors, methods, properties, and inheritance to develop modular and reusable programs.
- Prototypal Inheritance:
Discover JavaScript's special inheritance paradigm, known as prototypal inheritance, in which objects inherit attributes and functions from their prototype objects. Recognize how this varies from conventional class-based inheritance and learn how to take use of it.
class Ball {
constructor(x, y, radius, color) {
this.x = x;
this.y = y;
this.radius = radius;
this.color = color;
this.dx = 2;
this.dy = 3;
}
draw(ctx) {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, 2 * Math.PI);
ctx.fillStyle = this.color;
ctx.fill();
}
update() {
this.x += this.dx;
this.y += this.dy;
if (this.x + this.radius >= canvas.width || this.x - this.radius <= 0) {
this.dx *= -1;
}
if (this.y + this.radius >= canvas.height || this.y - this.radius <= 0) {
this.dy *= -1;
}
}
}
const ball = new Ball(50, 50, 10, 'red');
function animate() {
ball.update();
ball.draw(ctx);
requestAnimationFrame(animate);
}
animate();
3. Asynchronous Programming: Handling the Flow of Time
*- Callbacks & Promises: *
Master the art of handling asynchronous operations in JavaScript using callbacks and promises. Understand how callbacks work and how promises provide a cleaner and more predictable way to manage asynchronous code flow.
*- Async/Await: *
Embrace the power of async
and await
keywords to write cleaner and more readable code for asynchronous operations. Learn how to handle multiple asynchronous operations concurrently and avoid callback spaghetti!
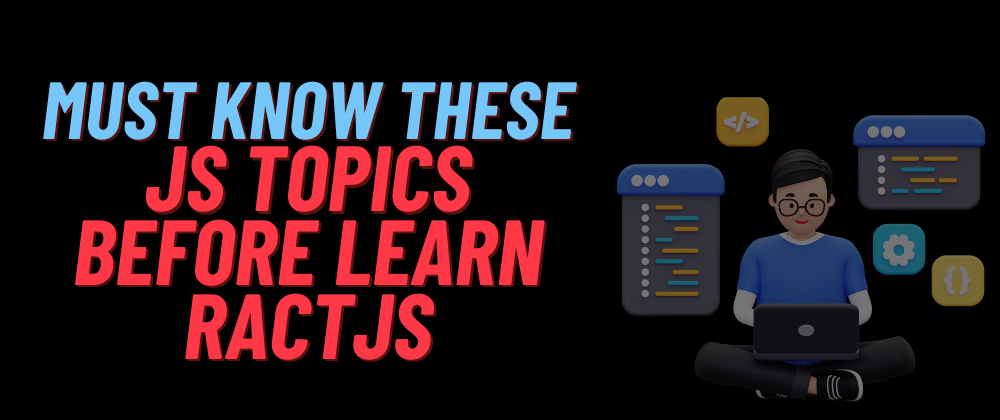async function fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
}
fetchData().then(data => {
// Update the UI with the fetched data
const element = document.getElementById('data-container');
element.innerHTML = data.map(item => `<li>${item.name}</li>`).join('');
});
4. DOM Manipulation: Bringing Your UI to Life
- DOM Fundamentals:
Recognize how your website's HTML elements are represented by the Document Object Model (DOM). Discover how to use JavaScript to access and edit DOM elements, changing their content, styles, and attributes.
- Events & Event Listeners:
Learn how to utilize events and event listeners to record user interactions with your website. Discover how to manage key presses, scrolls, clicks, and other user events by adding event listeners to DOM elements.
5. Debugging & Error Handling: Your Superpowers for Troubleshooting
*- Console & Debugging Tools: *
Learn how to use the browser console and debugging tools to identify and fix errors in your JavaScript code. Understand how to use breakpoints, step through code, and inspect variables to diagnose issues.
*- Error Handling: *
Don't let errors stop you! Learn how to write proper error handling code to gracefully handle runtime errors, log them effectively, and provide helpful feedback to users.
Keep in mind that these are only the fundamental components. After you've grasped these fundamentals, you'll be ready to take on the fascinating world of React JS with assurance and create incredible online applications. So pay attention to these fundamental ideas, put them into practice, try new things, and don't be embarrassed to ask for assistance!
Bonus SEO Tips:
Use relevant keywords throughout the article, including "JavaScript," "React JS," "beginners," and "fundamentals."
Include internal links to other relevant articles on your website.
Optimize your article for mobile devices.
Promote your article on social media and other online communities.
Top comments (0)