Greetings, fellow tech enthusiasts, and welcome to a tech journey like no other! π Today, we're embarking on a roller-coaster ride through the fascinating world of gRPC, Flutter, and Golang, but with a twist β we've spiced it up with a generous serving of humor. Buckle up; it's going to be a wild ride!
In our three-part series, we'll explore the ins and outs of gRPC, the delightful dance between Flutter and Golang, and discover how these technologies can come together to create harmonious applications that can bring a smile to even the most stoic developer's face.
Table of Contents
-
- Create project
- Setup Database model
- Password Hashing
- Environment
- JWT
-
- Service
- Message
A Quick Peek
- Part 1: Authentication & Authorization
- Part 2: Custom Flutter Notifications
- Part 3: Chat Applications with gRPC
gRPC: A Not-So-Boring Bookish and Slightly Amusing Definition
Alright, time to don our academic spectacles and approach this with a hint of whimsy. Imagine you're at a fancy library soirΓ©e, and someone asks, "What, pray tell, is gRPC?" You respond with a grin:
"Gentlefolk, gRPC, which stands for 'Google Remote Procedure Call,' is like the telegraph system of the digital realm. It's a meticulously structured, high-tech method for different programs to chit-chat with each other, just like sending telegrams back in the day, but without the Morse code."
"But wait, there's more! Instead of tapping out dots and dashes, gRPC uses Protocol Buffers, which is like encoding your messages in secret spy code, only it's not so secret. This makes your data compact and zippy, perfect for today's speedy world."
"Picture it as a virtual switchboard operator connecting your apps with finesse. Plus, it plays nice with HTTP/2, which is like giving your data a sleek, sports car to zoom around in, making it super fast."
"So, in essence, gRPC is the gentleman's agreement between software, ensuring they can communicate efficiently and reliably, like two well-mannered tea-drinking robots having a chat. It's the technological equivalent of a courteous bow, allowing different systems to exchange information harmoniously."
gRPC vs REST: The Iron Throne of APIs
Aspect | gRPC | REST |
---|---|---|
Protocol | Uses HTTP/2 for communication. | Typically uses HTTP/1.1, but can use HTTP/2. |
Data Serialization | Uses Protocol Buffers (ProtoBuf) for efficient binary serialization. | Uses human-readable formats like JSON or XML. |
Communication Patterns | Supports unary, server streaming, client streaming, and bidirectional streaming. | Primarily supports request-response (HTTP GET, POST, etc.). |
Payload Size | Smaller payload due to binary serialization, making it more efficient. | Larger payload due to text-based serialization. |
Performance | High performance and low latency, suitable for microservices. | Slightly slower due to text-based serialization. |
Language Agnostic | Supports multiple programming languages, making it polyglot. | Can be used with any language, but not as standardized. |
Code Generation | Generates client and server code automatically from Protobuf definitions. | No automatic code generation for client or server. |
Error Handling | Uses status codes and detailed error messages for robust error handling. | Typically relies on HTTP status codes and custom error messages. |
Tooling | Well-documented and supported by various libraries and tools. | Widely supported with many tools and libraries available. |
Types of gRPC
Here are the primary types of gRPC communication:
Unary RPC (Request-Response): This is the simplest form of gRPC communication. The client sends a single request to the server and waits for a single response. It's similar to traditional request-response interactions.
Server Streaming RPC: In this pattern, the client sends a single request, but the server responds with a stream of messages. It's useful when the server needs to push multiple pieces of data to the client, such as real-time updates.
Client Streaming RPC: Here, the client sends a stream of messages to the server, and the server responds with a single message. This can be beneficial for scenarios like uploading large files.
Bidirectional Streaming RPC: In this type, both the client and server can send a stream of messages to each other concurrently. It's ideal for interactive and real-time applications like chat or gaming.
In this we are going to explore unary gRPC using login,signup and get-user request.
Prerequisites
- Basic understanding of Golang,Flutter and MongoDb.
- Excitement to learn something new.
Setup Basic Project in Golang
- Create new project ```
go mod init
- Install Mongo package for go
go get go.mongodb.org/mongo-driver
- Setup Database Model
- Create package `db`
- Create Struct to access database
- Create new model `user.go`
-
Password hashing : we are going to create function for hashing password and check password, Never store password in simple text.
- Create package
utils
in root.
- Install required package
go get golang.org/x/crypto/bcrypt
.
- Create
HashPasword
and CheckPassword
function in password.go
-
Setup Environment: We required few environment variable like server_address
,mongodburl
and jwt_key
etc.
-
Install Viper package for loading app.env
.
go get github.com/spf13/viper
for more information read about viper
- Create function in
utils
to loadapp.env
file.
- Create
app.env
in root folder.
DB_NAME=grpc
DB_SOURCE=mongodb://localhost:27017
RPC_SERVER_ADDRESS=0.0.0.0:9090
GIN_MODE=debug
TOKEN_SYMMETRIC_KEY=12345678123456781234567812345678
ACCESS_TOKEN_DURATION=600m
- Setup Jwt: We need authorization in few requests so its going to
bearer
token based authentication.- Install required Jwt package
go get github.com/dgrijalva/jwt-go
Note: Not Going to cover Jwt Basics.
- Create new package token and create interface to create token and verify token.
Basic of Protocol Buffer (.proto
).
Service: A unary service in gRPC involves a single request from the client to the server, which then sends a single response back to the client. To define a unary service, you need to create a .proto file that describes the service and the message types it uses.
-
Message: A message is a data structure that represents the information being sent between the client and server. It's defined in your .proto file using the message keyword. Here's a simple example:
syntax = "proto3";
message MyRequest {
string name = 1;
int32 age = 2;
}
message MyResponse {
string greeting = 1;
}
In this example, we have two messages, `MyRequest`and `MyResponse`. `MyRequest` has two fields, name and age, while `MyResponse` has a single field, greeting.
- Importing Data Structures from Another Package:
import "other_package.proto";
message MyRequest {
other_package.SomeMessage some_data = 1;
}
## Create Protocol buffers for our project:
- Services: We will have following services called `login`, `signup` and `get-user`.
- Create `user.proto`, User object to return to the client.
syntax = "proto3";
package pb;
option go_package="github.com/djsmk123/server/pb";
message User{
int32 id=1;
string username=2;
string name=3;
}
- Create `rpc_login.proto`, it will have LoginRequestMessage and LoginReponseMessage
syntax="proto3";
package pb;
import "user.proto";
option go_package="github.com/djsmk123/server/pb";
message LoginRequestMessage{
string username=1;
string password=2;
}
message LoginResponseMessage{
User user=1;
string access_token=2;
}
- Same for `rpc_signup.proto`:
syntax="proto3";
package pb;
import "user.proto";
option go_package="github.com/djsmk123/server/pb";
message SignupRequestMessage{
string username=1;
string password=2;
string name=3;
}
message SignupResponseMessage{
User user=1;
}
- To create message `rpc_get_user.proto`:
syntax="proto3";
package pb;
import "user.proto";
option go_package="github.com/djsmk123/server/pb";
message GetUserResponse{
User user=1;
}
- Create service `rpc_services.proto`
syntax="proto3";
package pb;
option go_package="github.com/djsmk123/server/pb";
import "empty_request.proto";
import "rpc_get_user.proto";
import "rpc_login.proto";
import "rpc_signup.proto";
service GrpcServerService {
rpc SignUp(SignupRequestMessage) returns (SignupResponseMessage){};
rpc login(LoginRequestMessage) returns (LoginResponseMessage){};
rpc GetUser(EmptyRequest) returns (GetUserResponse) {};
}
message EmptyRequest{
}
## Generate code for Golang
- Create `pb` package in root folder.
- Run command to generate equivalent code for golang
protoc --proto_path=proto --go_out=pb --go_opt=paths=source_relative \
--go-grpc_out=pb --go-grpc_opt=paths=source_relative \
proto/*.proto
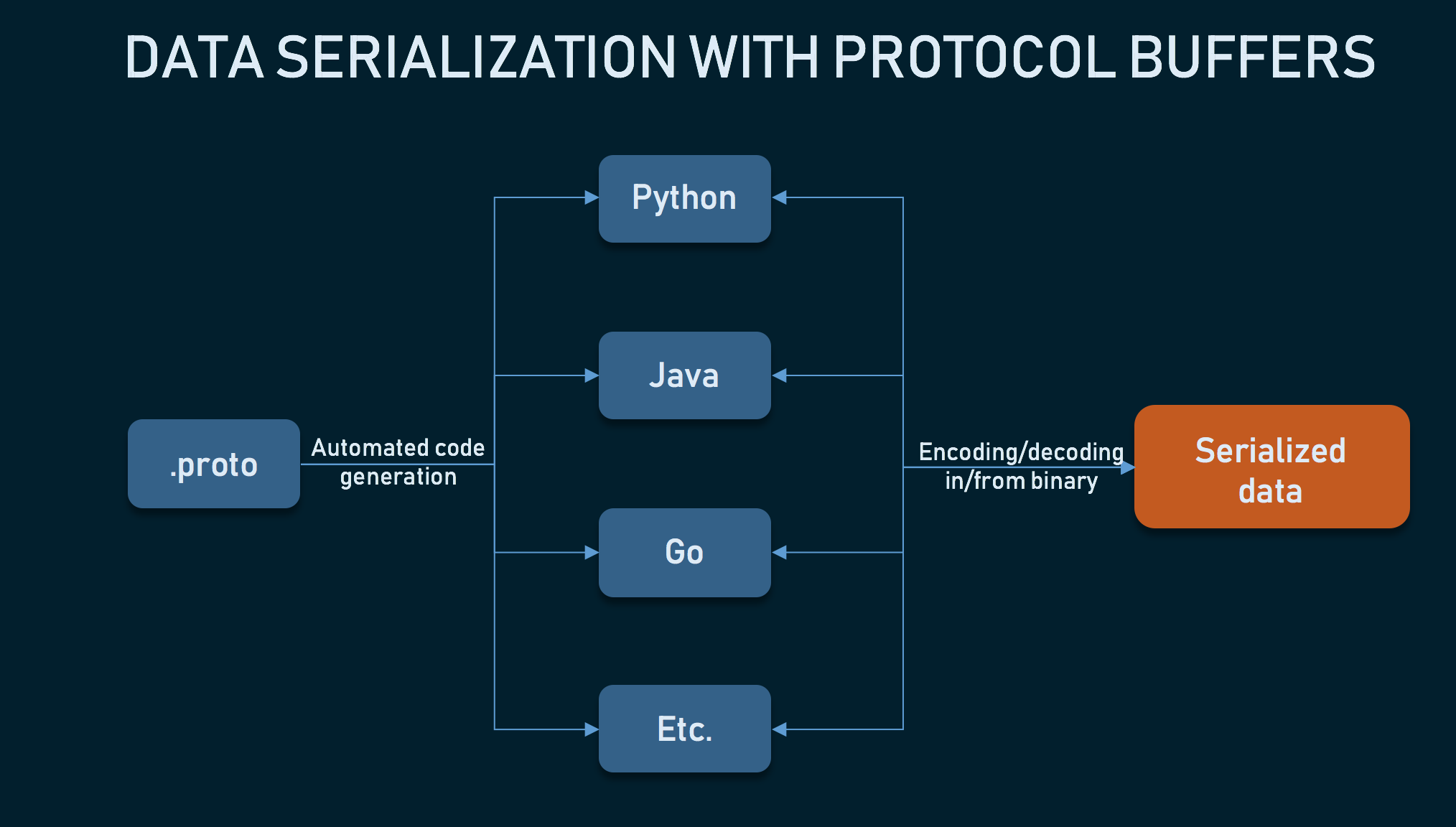
## Create Basic Auth function
- Create `Auth` Package: This package has basic function like `get-user`,`login`, `signup`,`tokenCreation` and function to convert MongoDB userModel to gRPC `user` Messsage.
- Create `converter.go` in `auth` package.
-
Create token_generate.go
to generate token.
-
Create login.go
Create signup.go
- Create
get-user.go
Setup Server and Middlware
- Create
server.go
in gapi
package struct to register GrpcServiceServer
.
- For creating middlware for Authentication, we will need
Unary Interceptors
but before that we will check,if request service required authentication or not, we will list all method that are not required token.
> Note by default all the method have restriction for auth token.
Create services.go
- Create
middlware.go
Connect whole and start server
finally we are going to main function in main.go
,lets load environment, connect database(MongoDb) and RunServer.
Run Server
go run .
## Test server
You can test gRPC services using [`evans-cli`](https://github.com/ktr0731/evans).
- Start CLI Tool
evans --host localhost --port 9090 -r repl
- Call `login` service
call login

!!!Oops,we forgot to define `login`,`get-user` and `signup` from `pb` interface.
- Create `auth.go` in `gapi` package.
Try again
Flutter Project
This Flutter application features four key pages: SplashScreen
, LoginScreen
, SignUpScreen
, and HomeScreen
.
SplashScreen: This initial page verifies the user's authentication status. If authenticated, it communicates with the gRPC
server using the get-user
service and redirects the user to the HomeScreen
. Otherwise, it navigates to the LoginScreen
.
LoginScreen: The LoginScreen presents fields for entering a username
and password
. Upon pressing the submit button, the application invokes the login
service to authenticate the user. The access_token
is saved to local storage, and the user is redirected to the HomeScreen
.
SignUpScreen: The SignUpScreen offers fields for name
, username
, password
, and confirmpassword
. When the user submits the form, the application calls the signup
service on the gRPC
server.
> Note: Not going to create whole UI and remaining Login,but only will cover required parts. You are free to create your own ui or can refer from whole in github repo.
Setup Project
- Add following dependencies into
pubspec.yaml
shared_preferences: ^2.2.1
protobuf: ^3.1.0
grpc: ^3.2.3
- Install `protoc_plugin` for dart.
dart pub global activate protoc_plugin
- Copy `.proto` files from server to root folder of the flutter project.
- Created equivalent code in dart
protoc --proto_path=proto --dart_out=grpc:lib/pb proto/*.proto
- Create channel for gRPC services `services/grpc_services.dart`
- Create AuthServices for different method like
login
,signup
and get-user
- We are done just call function in presentation layer and add your own logic.
OUTPUT
Thanks for joining me on this tech adventure! We hope you've enjoyed the journey through gRPC, Flutter, and Golang as much as I have. But wait, the fun doesn't stop here!
Stay tuned for my upcoming blogs, where I'll sprinkle more humor, insights, and tech wizardry into your day. Who knows, in my next episode, we might just unveil the secret to debugging code with a magic wand (disclaimer: we can't actually do that, but a coder can dream, right?).
Until then, keep coding, keep laughing, and keep those fingers ready on the refresh button because the next tech tale is just around the corner! πβ¨"
Source code
Follow me on
Top comments (6)
One interesting topic is authentication with Web Client. Assume you added gRPC Web Proxy in front of your server to serve Web clients over HTTP 1.1. You still need the JWT token for authentication and client need to store is somewhere. The most apparent place is local storage. Yet this values can be read without any limmits and hence the token can be stolen. In typical Web apps this kind of issues is solved by server-side cookies (cookies which the client acepts and resends to server with every request yet can't read via JS). Anh there no server sode cookies in gRPC :)
If you happen to consider this use case - I am very much interested. I beleive when using Web handling of JWT and translating it to server side auth cookie must be done by the web proxy.
Thanks for feedback.
I consider server side cookies using gRPC gateway to that will verify but It will add complexity to the blog.
I could cover this topic in anotherb blog but before that I have to test and verify the solution.
Indeed, that is not a small/easy topic.. And it tends more towards infrastructure rather than programming.
Great tutorial. I love it π
Thanks
In case of you found typos,mistake in code and any other feedback.
Please comment and let me know