So after the first day at my coding program we went over variable declaration and the variety of ways to utilize them but came to a block when it came to declaring a variable as null what would or could be a method of utility of declaring a variable as null any thoughts?
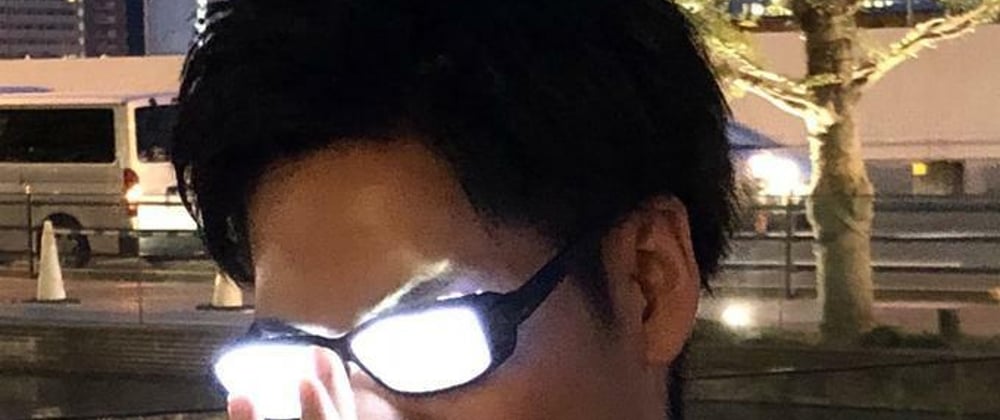
For further actions, you may consider blocking this person and/or reporting abuse
Top comments (2)
Mozilla Developer Network (MDN):
Typically it's a good idea to have a look at the MDN Web Docs first when it comes to JavaScript and browser web APIs.
Now some people find this confusing but as MDN states:
or put differently
null
is a reference that indicates the absence of an object where one might be expected.However many developers have generalized
null
simply as the absence of any value (notice the absence of "object", so this includes primitive values) and go on to act on the convention to usenull
when they programmatically bind the absence of a value to a variable name.The thing is that there are areas of JavaScript that don't play along with that perspective.
ES2015 introduced default parameters:
3
one
isundefined
so the default value forone
is used instead; so the result is5
one
isnull
which does not fall back to the default value. Instead later thenull
is explicitly converted to to0
using the nullish coalescing operator; so the result is 4.This doesn't change even when we pass a default object:
Optional Chaning
So when it comes to default parameters
undefined
represents the absence of any value - to be replaced with the default value.Another example:
values
has a hole (orempty
) at index1
while there is anundefined
value at index2
. Accessing either index with the bracket notation will result in anundefined
value. Only hasOwnProperty(index) reveals that both are actually different, i.e. that there is anundefined
value at index2
while there is a missing (absent) value at1
.Interestingly when specifying the
arrayLength
with the Array constructor a sparse array is created; that means that it isn't filled withundefined
values but instead (conceptually) only consists of holes (empty values).Now some people may take holes as a example of
undefined
being used to indicate "uninitialized" butundefined
can be bound to any variable name just like any other primitive value.Now if we divert to TypeScript for a moment there is the notion of optional parameters:
What is the type of
x
? It'snumber | undefined
, i.e. either somenumber
orundefined
— but notnull
(because this needs to be consistent with how JavaScript default parameters behave).Similarly there are optional properties:
Again the type of
xPos
andyPos
isnumber | undefined
. However here the property can be missing entirely (similar to an array hole) or it could exist with a value ofundefined
(though Exact Optional Property Types can disallow theundefined
value and require an absent property instead).Note that TypeScript's contribution guidelines state: "Use
undefined
. Do not usenull
".Much earlier (2014) Douglas Crockford also argued against using
null
.That said you will always encounter
null
.Example Document.querySelector():
"Return value
An Element object representing the first element in the document that matches the specified set of CSS selectors, or
null
is returned if there are no matches."querySelector()
is expected to return an object. When no matching object is found the intentional absence of any object value is communicated withnull
.Edit: Intent to stop using
null
in my JSI am also working on JavaScript 'basics'. I do not have much information on 'null' but here is an example of it being used in a recent project.
My code (it's short):
const myToDo = ['Cook', 'Study', 'Work']
let input = prompt('What would you like to do: (New, List, Delete, Quit)'); //let input = prompt starts our whole 'to-do' list function.
while (input === "New") {
let newToDo = prompt("What would you like to 'ADD' to the list?");
if (newToDo !== undefined) {
alert(
${newToDo} added to the list.
);}
}
When the variable newToDo prompts and asks the question: "What would you like to 'ADD' to the list?" If you hit 'cancel' before making any input, the default response comes up as my alert function and says 'null added to the list'.
So, I'd say that null can be declared as a variable... but it's also used to declare a 'no-command' sort of thing I suppose.
Definition of null = having a no value, or a value of zero.