Yo, (scarf) dog. I heard you like functions, so I put a function in ur function so that you can function while it functions. < / oldMemes >< / badJoke >
ANYWAY, so my friend was starting to learn how to code and she needed help with understanding what the teacher was asking her to do.
This is what she sent me:
/*
Create a function that takes in two inputs.
One should be a function and the other should be
the argument to call the input function with.
Then in the function you define call the passed in function
with the input argument.
*/
...excuse me, but lolwut?
Here's the example function she sent:
function sayHi(b,c){
c=prompt("Greet me!");
b(c);
Okay, that's a bit more clear, I guess.
So, here we go:
From what I understand, we're looking to build a function that runs another function. I used the concept of Pokémon evolution with stones to illustrate this.
Evolution is the overall universal function.
function evolutionFn(pokemon, stone){
stone = prompt('Which stone will you use?');
return pokemon(stone);
}
Pokémon themselves are separate functions, but still universally utilize the same evolution function. The best example to use would be Eevee because the possibilities of their evolution are vast. (But for now, we're referencing their basic gen 1 evolutions).
const eevee = (x) => {
let userInput = x.toLowerCase();
if ( userInput === 'fire' ){
return 'Congrats! You now have a Flareon!'
}else if( userInput ==='thunder' ){
return 'Congrats! You now have a Jolteon!'
} else if( userInput === 'water' ){
return 'Congrats! You now have a Vaporeon!'
} else {
return 'Huh. It didn\'t work.'
}
}
I also created Pikachu to illustrate that a completely different Pokemon can utilize this method of evolution as well.
const pikachu = (x) => {
let userInput = x.toLowerCase();
if ( userInput === 'thunder'){
return 'Congrats! You now have a Raichu!'
} else {
return 'Huh. It didn\'t work.'
}
}
Put it all together and we get the following:
function evolutionFn(pokemon, stone){
stone = prompt('Which stone will you use?');
return pokemon(stone);
}
const eevee = (x) => {
let userInput = x.toLowerCase();
if ( userInput === 'fire' ){
return 'Congrats! You now have a Flareon!'
}else if( userInput ==='thunder' ){
return 'Congrats! You now have a Jolteon!'
} else if( userInput === 'water' ){
return 'Congrats! You now have a Vaporeon!'
} else {
return 'Huh. It didn\'t work.'
}
}
const pikachu = (x) => {
let userInput = x.toLowerCase();
if ( userInput === 'thunder'){
return 'Congrats! You now have a Raichu!'
} else {
return 'Huh. It didn\'t work.'
}
}
console.log(evolutionFn(eevee));
// example: if prompt => 'fire or FIRE or even FiRe',
// it will say "Congrats! You now have a Flareon!"
// if it's anything else, the console will return "Huh. It didn't work."
console.log(evolutionFn(pikachu));
// Should return "Congrats you now have a Raichu"! etc. etc.
Play with it live on Repl.it!
And there you have it-- Function composition: when you want to use a big function to execute little functions that essentially will output the same basic thing.
Also, fun fact-- you've been bamboozled! If you've ever used .map(), .split(), .join(), .reverse(), you have already experienced Function Composition! We see this in action when we use JavaScript methods ALL. THE. TIME.
Thanks for reading!
If you'd like to keep in touch with me, follow me on Twitter! DMs are open.
Also, sign up for my newsletter where I give you tips/tricks on how to survive coding bootcamp and post-bootcamp/learning on your own by sharing some personal (sometimes embarassing) stories!
Top comments (15)
Nice, this is the beauty of JavaScript. Passing in functions as arguments make the language to do some interesing stuff..
Also not relevant to composition but using switch statement is better inside eevee function since there are more than 2 different conditionals.
Higher order functions are not unique to JavaScript tho, so it's just a really nice feature.
Good to know :)
True. I wanted to use the switch function, but since my friend was a complete newbie and was already inundated with all the info, I stuck with what she knew: if/else. lol
I'm new to Dev world as well, And your example was explained really well, But I didn't understand why the Evolution function needs a second argument in it's definition.. As we are prompting the user to input the second argument.
I was under the thought that I needed a “holder” for the prompt so we can pass that through the individual Pokémon function to get their evolution result.
Also, I wanted to help my friend meet assignment requirements, which asked for 2 arguments.
But, I should definitely try it out without the second parameter.
*article
Ohhh my god lmao those 3 idiots.
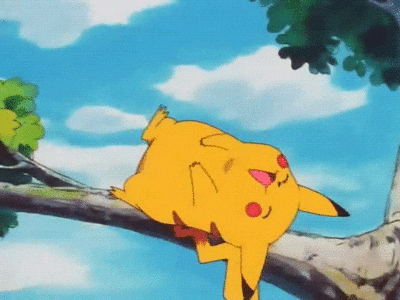
I loved this. Absolutely loved it. But I'm pretty sure I need a lie down - never was any good at Pokemon :)
I can't even with the latest stuff with "IV"s and shiny hunting stuff these days. But I'm glad you enjoyed the article!!
Awesome wat of explaining this!
Thank you!!!
OMGGGG I loved, I love explain things using pokemon too, your article was very good, I will share it with the people of the Brazilian communities <3 Tnkss Cat
I always forget that .map(), .split(), .join(), and .reverse() are examples of function compositions. But your Pokemon references really helped me understand them more!
I'm glad it helped!! :)
Some comments may only be visible to logged-in visitors. Sign in to view all comments.