NPM (Node Package Manager) packages are an essential component of Node.js. They allow developers to share their solutions with the wider Node.js community. NPM packages consist of reusable blocks of code that can be easily integrated into a project with a single command. By utilizing NPM packages, developers can avoid reinventing the wheel and focus on building.
Each NPM package is identified by a unique name and version number, allowing developers to specify and install specific versions of packages in their projects.
NPM packages include a wide range of tools such as frameworks like Express or React, libraries like jQuery, and task runners such as Gulp, and Webpack.
In this guide, you will learn how to create and publish an NPM package. Let’s get to it.
Prerequisites
To follow the steps detailed in this article, you must have the following:
- Basic understanding of Node.js
- Node.js installed
- An NPM registry account
- A code editor, preferably Visual Studio Code
What is NPM?
NPM stands for Node Package Manager. It is the default package manager of Node.js. NPM provides two main functionalities:
- NPM CLI: The NPM CLI, also known as 'npm', is a command-line utility that enables developers to install, manage, and publish packages.
- NPM Registry: is a repository where developers can publish both public and private packages, making them accessible to others.
Packages are simply JavaScript projects.
NPM is the biggest JavaScript package manager. As of the time of writing this article, there are over 2.6 million packages available on the official NPM registry, which range from small utility packages to large-scale frameworks. These packages have been downloaded over 216 billion times.
This success can be attributed to Node.js' active and thriving ecosystem.
In Node.js, every project contains a metadata file named package.json
at its root, which is created and maintained by NPM. This file contains vital information about a project, including its name, version, description, author, license, dependencies, and scripts. The package.json
file serves as a central configuration file for Node.js projects and is crucial in managing dependencies.
Dependencies are packages installed in a Node.js project.
Additionally, NPM also provides some quintessential commands to do several tasks such as initializing a project, installing a package, and publishing a package. The most common commands include npm init
, npm publish
, npm install
NPM is critical in the Node.js ecosystem, as it promotes collaboration, solution sharing, faster development, and better software quality. It has become a must-have tool for Node.js developers and is widely used in open-source and enterprise projects.
How to create an NPM package
Before following the steps in creating an NPM package, it is recommended to decide on a name for your NPM package first. Do well to visit the NPM registry to confirm the availability of your desired name.
If your desired name is taken, consider choosing a different name. Attempting to use a name that is already taken (or something similar) will cause Node.js to throw an error.
For example: If a package named rgbcolorpicker
already exists, you won't be able to publish a package with the name rgb-color-picker
. Make sure to choose a unique and available name for your package.
However, if you're still keen on using the taken name, you can publish the package as a scope package. More information on how to do this will be provided later.
Now that you have your package name. Let’s continue:
Setting up an account
- First, create an account on the NPM registry website. If you already have an account, log in.
Keep your credentials safe as they will be required to publish your package.
Project structure
- Create the following files and folders required for this project:
My-First-Package
└─ main.js
Below is a visual illustration:
Project initialization
- Open your terminal, navigate to the root of your working directory, and initialize your project by running this command:
npm init
- You will prompted by npm to provide certain information to generate a
package.json
file.
The importance of a
package.json
file in an NPM package cannot be overstated.
-
To assist you in creating the
package.json
file, here is a guide on how to enter the necessary information:- package-name: Here, enter your selected package name. Ensure it is in lowercase and make sure to remove any trailing spaces.
- version: It's recommended to keep the initial value and update the version of the package as you make changes.
- description: Describe your package.
- entry point: This is the entry point of your package. Keep the initial value unless you plan on using a different file as your entry point.
- test command: If you have any test commands for your package, enter them here. Leave this field blank if you have none.
- git repository: Enter the link to the Git repository where this package is located.
- keywords: Provide relevant keywords such as "library", "framework", or "task-runner" to help others easily find your package.
- author: Enter your name or alias.
- license: Here, enter a license if you have one. If not, leave this field blank.
Here is a visual representation that shows how to correctly enter your information:
Writing your code
- Open your
main.js
file and insert the provided code snippet:
function generatePassword(length, includeUppercase, includeNumbers, includeSpecialChars) {
if (!length || typeof length === "string"){
return new Error ("Please provide password length")
}
const lowercaseChars = 'abcdefghijklmnopqrstuvwxyz';
const uppercaseChars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ';
const numberChars = '0123456789';
const specialChars = '!@#$%^&*()_-+=<>?/{}[]';
let validChars = lowercaseChars;
let password = '';
if (includeUppercase) validChars += uppercaseChars;
if (includeNumbers) validChars += numberChars;
if (includeSpecialChars) validChars += specialChars;
for (let i = 0; i < length; i++) {
const randomIndex = Math.floor(Math.random() * validChars.length);
password += validChars[randomIndex];
}
return password;
}
module.exports = generatePassword
-
In the code snippet above:
- The
generatePassword
function is designed to create a password and requires four arguments to run. The first argument is the desired length of the password. The remaining arguments are optional and are used to determine if the password should include uppercase letters, numbers, or special characters. - The function is then exported so others can access it.
- The
Test your package
The next step is to test the password-generator package to ensure it works as intended.
- Navigate to your terminal and enter the command: ```
npm link
> _The npm link command allows you to create a symbolic link between a package and the global npm registry, making it accessible to other projects on your machine without needing to install it. This is particularly useful during development, as it allows you to quickly test changes without having to publish and update the package each time you modify._
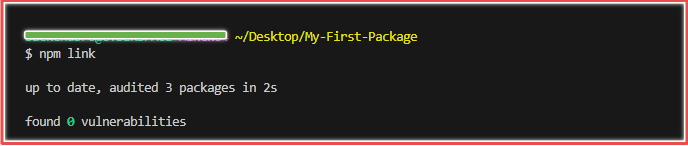
- Create a new folder and file to require the password-generator package
Test-Folder
└─ test.js
- Make sure to navigate to the **`Test-Folder`** working directory in your terminal and run the commands sequentially
npm init -y
npm link
> _To initialize a directory or folder, you can use the **`npm init`** command. By adding the **`-y`** flag, you can skip the prompts and save time. The **`test-folder`** is specifically used for testing, so there is no need to spend time providing any necessary information._
> _The **`npm link <packagename>`** command will add the specified package to your project_
>
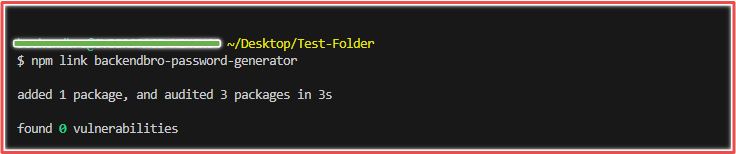
The **`npm link <packagename>`** command created a **`node_modules`** folder. This folder holds packages installed by npm. To find your package, navigate to the corresponding folder inside **`node_modules`**

- Open the **`test.js`** file and require the package.
const passwordGen = require('backendbro-password-generator')
const password = passwordGen(10, true, false, true)
console.log(password)
- To complete the testing process, execute the command
node test.js

Voila! The package works as suppose. Let’s continue by publishing to the NPM registry.
## Publishing to the NPM Registry
After testing your NPM package, you can publish it to the NPM registry. Here's a simple guide to follow:
### Login to the NPM registry:
- To get started, you need to create an [account](https://www.npmjs.com/). If you already have one, log in.
### Publish:
- Open your terminal, navigate to your package directory, and run the command:
npm login

- You will be redirected to the NPM registry. Enter the One-Time Password (OTP) sent to your email address to complete the authentication process.

- To publish your package, enter the command provided below:
npm publish

Yay! Your package has been successfully published to the NPM registry. You can now search for it on the registry.

## Publishing Scoped Packages
In previous sections, we talked about how to publish packages as scoped packages to work around using an already-taken package name. Scoped packages allow you to create a namespace for your package to avoid naming conflicts.
Scoped packages are private by default. However, you can choose to grant access to specific individuals who are allowed to use your package. Private packages require a paid subscription plan, but there is no need to worry about that. You can publish your scoped package as public to avoid any fees.
If you decide to make your scoped package public after publishing it, you can easily change its visibility by updating the access field in your package.json file from **restricted** to **public**.
Creating and publishing scope packages is a simple process. To do so, follow the steps below:
### Project structure
Create a new project
Scoped-package
└─ main.js
### Project initialization
- To begin, you need to initialize your directory by running the following command:
npm init --scope=@username
- When entering the package name in the npm prompt, follow this format: **@username/package-name**. See the example image below.

### Writing your code:
- Copy the **`generatePassword`** function from the previous section and paste it into the **`main.js`** file
### Testing the package:
- Follow the same procedure as described in the previous section to test your package.
### Publishing:
- To publish your scope package, open your terminal and run the command given below:
npm publish --access public
> _Remember: By default, scoped packages are private. Another way to make a package public is by setting the **`access`** flag to public._

Ta-da! You have successfully published your first scope package. Visit the NPM registry to view it.

## Installing the NPM package
After publishing a package to the NPM registry, it is best practice to install and use it to ensure it works as a regular package.
### Project structure
Create a new project and initialize it using **`npm init`**.
Package
└─ main.js
### Installation
Use the following command to install the newly published package.
npm install packagname
> You can use **`i`** instead of **`install`** in the npm command. For example, instead of typing **`npm install`**, you can type **`npm i`**

### Usage
Open your **`main.js`** file and require the newly installed package.
const passwordGen = require("backendbro-password-generator")
const password = passwordGen(10, true, true, true)
console.log(password)
### Run your app
Simply run your application using:
node main.js

## Conclusion
Knowing how to create NPM packages is a vital skill for Node.js developers. It enables them to contribute to the open-source community and simplify their workflows by avoiding the need to reinvent the wheel.
In this guide, you have learned what NPM and NPM packages are and their importance to software development. You have also learned how to create and publish packages on the NPM registry.
You too can become a part of the ever-evolving Node.js landscape by sharing your package. Join in on this journey, share your solutions, and let's shape the future of software development together, one package at a time.
Thank you for staying with me and have a great time coding! 😊
## Useful Resources
- [NPM Official Documentation](https://docs.npmjs.com/)
Top comments (9)
I had a delightful time reading your article on creating and publishing an NPM package! Your step-by-step guide was both informative and approachable. As someone who’s dipped their toes into the world of package development, I appreciate the clarity you provided.
Choosing a unique package name is indeed crucial, and your advice on avoiding name clashes was spot-on.
Keep up the fantastic work, and I look forward to more insightful articles from you. Cheers! 🎉
Thank you so much for this heartwarming comment.
Hi Ukagha Nzubechukwu!
Great article, very useful
Thanks for sharing
Thank you very much.
I love this! Keep it up.
Thanks, I appreciate the kind words.
Great stuff, +1 on using npm link to test
I had to 😌
Great @backendbro , well structured and really useful article! 👌