👋 Hey all,
In this blog, we will explore the different types of comments and techniques that can be used in the Swift programming language.
Table Of Contents
Comments are an essential part of any codebase, allowing developers to convey important information about the code to other developers who may be reading it. We will discuss the types of comments and also discuss the best use cases for each one. Whether you are a beginner or an experienced developer, this post will give you a deeper understanding of how to use comments effectively in your Swift code.
# Types of comments
In most programming languages, comments are typically written in two ways:
- Single-Line comment : Single line comments start with "//" and continue until the end of the line. ```
// This is a single line comment
- **Multi-Line comment** :
Multi-line comments start with _"/*"_ and end with _"*/"_. They can span multiple lines.
/* This is a
multi-line comment */
It is also possible to nest multi-line comments by starting a new multi-line comment inside an existing one.
/*
This is a
multi-line comment
/*
nested multi-line comment
*/
*/
In addition to the traditional comments, Swift also has a unique type of comment known as:
- **Documentation comment** :
It is used to provide information about the code and is typically used to document the purpose, usage, and behavior of classes, methods, and properties. It is placed immediately before the code it describes.
Swift documentation comments can be written in two formats:
_A. Each line is preceded by a triple slash (///):_
/// This is a documentation comment
///
/// Here is the description
_B. Javadoc-style block comments (/** … */):_
/**
This is a documentation comment
Here is the description
*/
<a name="ways-to-use-comments"></a>
## [#](#ways-to-use-comments) Ways to use comments
There are several ways to use comments based on their purpose:
**1. Informative Comment:**
These provide a brief explanation of what the code is doing or what a specific function or variable is used for.
// This function calculates the average of two numbers
func average(a:Int, b:Int) {
// code
}
**2. Inline Comment:**
These comments provide explanations or clarifications for specific lines of code and are placed on the same line as the code they describe.
let x = 5 // This is an inline comment
**3. Debugging Comment:**
These functions are used as a comment for understanding what's happening in the app and for finding and fixing errors during the development process. The most common functions are:
_print()_, _dump()_, _debugPrint()_, _NSLog()_ and _os_log()_.
func average(a: Int, b: Int) -> Float {
let avg = (a + b) / 2;
debugPrint(avg);
return avg;
}
`debugPrint(avg)` is used as a comment during the development process.
**4. Organizational Comment:**
These are comments that are used to organize the code and leave reminders for yourself or other developers.
- `MARK:` syntax is used to create a clear visual separation in the source code, often used to organize code by functionality or feature.
// MARK: - View Life Cycle
- `TODO:` syntax is used to indicate that a specific task or issue needs to be addressed in the future.
// TODO: Add error handling
- `FIXME:` syntax is similar to TODO comments, but they indicate that there is an issue with the code that needs to be fixed as soon as possible.
// FIXME: Memory leak
Here is a screenshot of an example:
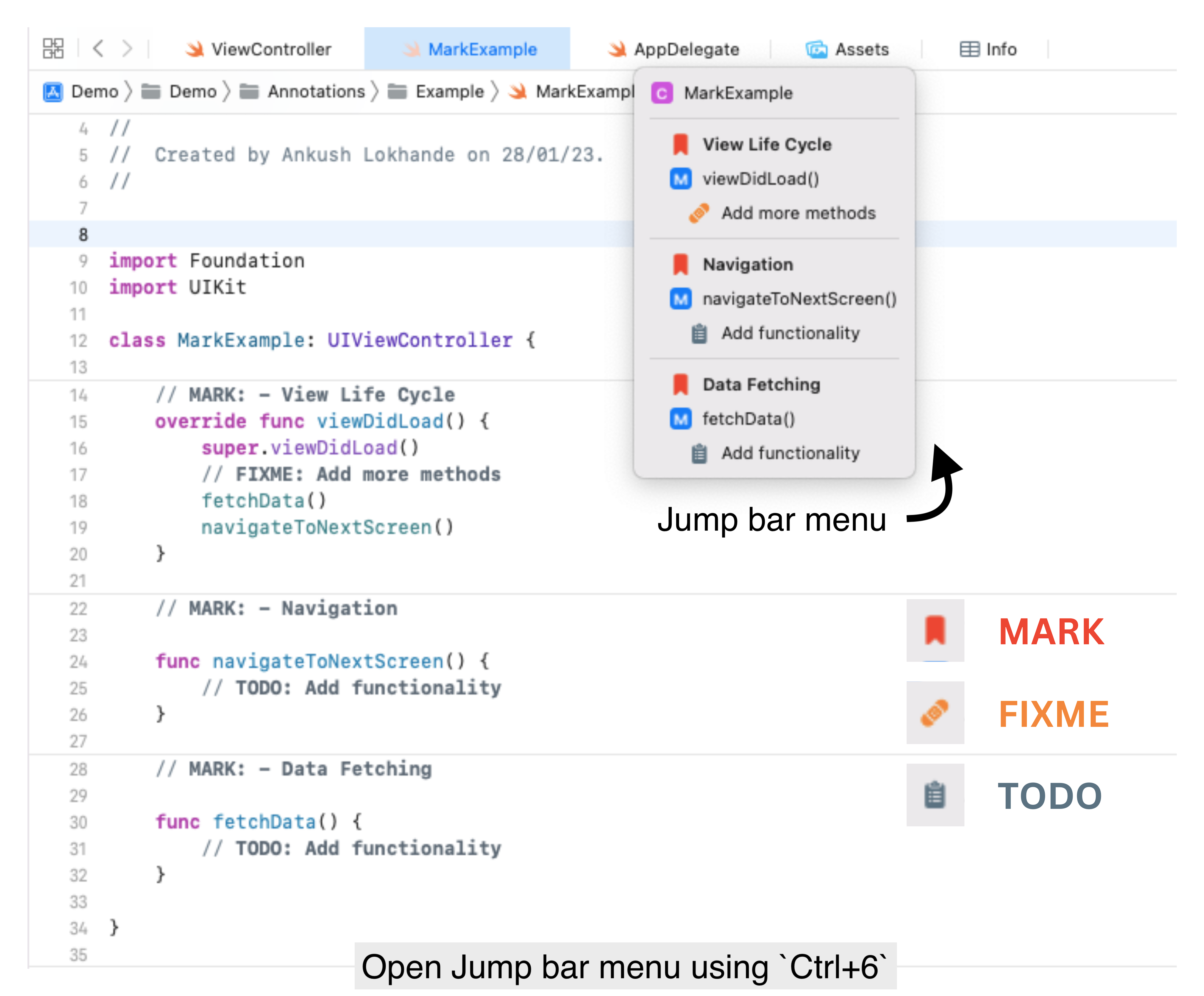
**Note:**
_`MARK:-` separates a section of code from the previous one, while `MARK:` groups sections together with the previous one._
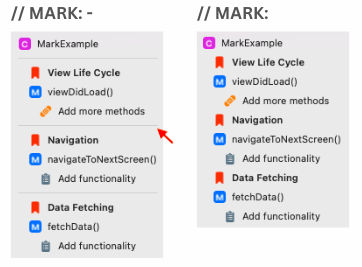
Other organizational tags also perform similar behavior.
**5. Deprecation Comment:**
These are comments that are used to indicate that a function or class is deprecated and should no longer be used.
@available(*, deprecated, message: "Use 'newFunction()' instead", renamed: "newFunction")
func oldFunction() {
// code
}

**6. Preprocessor Directives Comment:**
These are the directives tag can be used as a comment to include custom warning and error messages in your code.
- `#warning`
warning("This is a warning message.")

- `#error`
error("This is a error message.")

**7. Documentation Comment:**
These are comments that are used to document the purpose, usage, and parameters of a function or class.
/**
Call alert to handle the crashes and errors.
Use this method to to call alert at any instance
- Note: This method not required any instance of object, It calls globally.
{% raw %}`
`
GNMAlertController().showError(message: "Key not found")
- Parameters:
- message: - Error message
(String)
- message: - Error message
- Returns: null
*/
internal func showError(message: String) {
// code
}
` Here is the preview of documenation:
Here is the callouts list, You can also use as needed: Attention, Author, Authors, Bug, Complexity, Copyright, Date, Experiment, Important, Invariant, Note, Parameters, Postcondition, Precondition, Remark, Requires, Returns, Since, Todo, Version and Warning.
# Let's Wrap!
Commenting your code is an efficient way to improve readability, understandability, and maintainability for scalable projects. However, for small projects, clear and effective code alone may be sufficient.
Here is a link that provides tips on how to code effectively: Utilizing Clear Code as a Tool for Documentation
By following the above-mentioned techniques, you can ensure that your code is easy to understand, navigate, and maintain.
If you found this blog helpful or have any further questions, we would love to hear from you. Feel free to reach out and follow us on our social media platforms for more tips and tutorials on iOS development.
Top comments (0)