Hi folks, in the part 1 blog I shared the steps for setting and running up the node.js port. Today I want to continue this journey by creating routes. I choose the Todos app for which I'll write Create, Read, Update, and Delete (CRUD) todos APIs.
For this series, I'm following an excellent video tutorial from Traversy Media
Table Of Contents
Create todosController
Create a backend
folder in your project and add a controllers
folder in it.
All the features logic will go here with separate controller files. So now I'll add a todosController.js
file and create simple CRUD functions.
const getTodos = (req, res) => {
res.status(200).json({message: 'Get todos'})
}
const setTodo = (req, res) => {
res.status(200).json({message: 'Set todo'})
}
const updateTodo = (req, res) => {
res.status(200).json({message: `Update todo ${req.params.id}`})
}
const deleteTodo = (req, res) => {
res.status(200).json({message: `Delete todo ${req.params.id}`})
}
module.exports = {
getTodos,
setTodo,
updateTodo,
deleteTodo
}
I'll get the id
in the query param for update and delete todo API. I am just returning a static JSON message right now. I'll later replace it with the actual code.
Create a routes file
So now I'll add a todoRoutes.js
file inside /backend/routes
folder and initialize the router in it.
const express = require('express');
const router = express.Router();
const {getTodos, setTodo, updateTodo, deleteTodo} = require('../controllers/todosController')
router.route('/').get(getTodos).post(setTodo)
router.route('/:id').put(updateTodo).delete(deleteTodo)
module.exports = router;
Connect route in server.js file
And in server.js
file, I'll use this route like that
...
// add these lines to accept req body for POST call
app.use(express.json())
app.use(express.urlencoded({extended: false}))
app.use('/api/todos', require('./routes/todoRoutes'))
app.listen(port, () => {
...
P.S. Move your server.js file inside the backend folder. I didn't do it in the previous blog.
Now run the server and go to the postman and check your first route. It will give a result like this.
In the next blog, I'll connect the project with MongoDB and write the logic to add, update, delete and get todos.
Thank you for reading!
Feel free to connect on Twitter
Top comments (2)
I created a project structure through express-generator and implemented the second part.
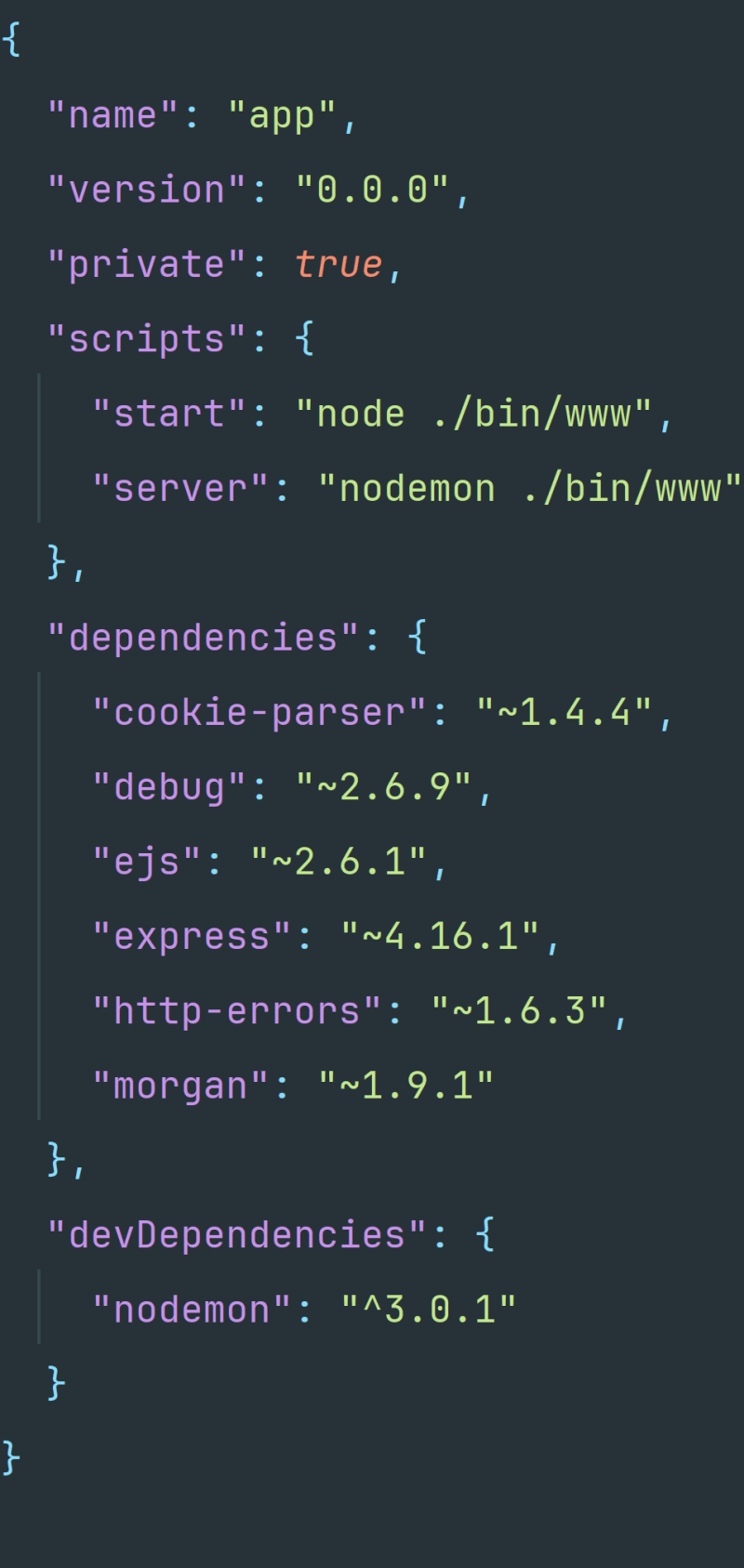
Hi, thank you for the nice blog. I have 1 problem. When i move server.js file to backend folder this will appear.

Is it necessary to rewrite the path to the file somewhere after the update?