Before you send a Word document to someone else, you may consider converting the document to PDF so it looks the same on different devices and can be viewed by recipients who don’t have MS Word installed. This article will demonstrate how to convert Word documents to PDF, PDF/A and password protected PDF in Java using Spire.Doc for Java library.
Add Dependencies
Method 1: If you are using maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>https://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>e-iceblue</groupId>
<artifactId>spire.doc</artifactId>
<version>5.4.2</version>
</dependency>
</dependencies>
Method 2: If you are not using maven, you can download the JAR file from this link, extract the zip file and then import the Spire.Doc.jar file under the lib folder into your project as a dependency.
Convert Word to PDF in Java
Convert Word to PDF is extremely easy with Spire.Doc for Java. You just need to follow the two steps below:
- Create an instance of Document class and pass the file path of the Word document to the class’s constructor as a parameter.
- Call the Document.saveToFile(filePath, FileFormat.PDF) method to save the document as PDF. ```java
import com.spire.doc.Document;
import com.spire.doc.FileFormat;
public class ConvertWordToPdf {
public static void main(String[] args){
//Create a Document instance and load a Word document
Document doc = new Document("Sample.docx");
//Save the document to PDF
doc.saveToFile("ToPdf.pdf", FileFormat.PDF);
}
}
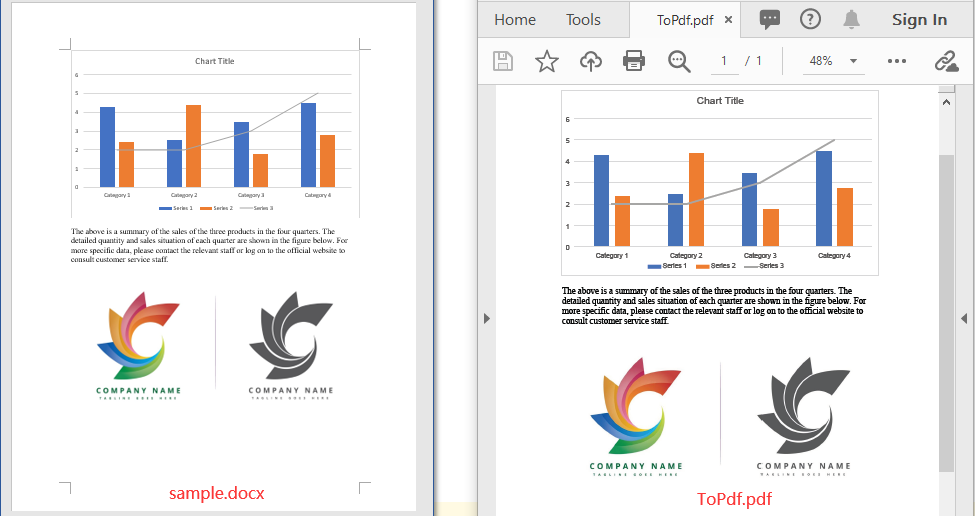
## Convert Word to PDF/A in Java
PDF/A is a special PDF format designed for long-term preservation of electronic documents. Spire.Doc for Java supports converting Word to the following PDF/A documents:
- PDF/A-1a
- PDF/A-1b
- PDF/A-2a
- PDF/A-2b
- PDF/A-2u
- PDF/A-3a
- PDF/A-3b
- PDF/A-3u
- PDF/x-1a:2001
The following are the steps to Convert a Word document to a PDF/A document:
- Create an instance of **Document** class and pass the file path of the Word document to the class’s constructor as a parameter.
- Create an instance of **ToPdfParameterList** class.
- Specify the conformance level of the PDF document using **ToPdfParameterList.setPdfConformanceLevel(PdfConformanceLevel)** method.
- Call **Document.saveToFile(filePath, ToPdfParameterList)** method to save the Word document to PDF.
```java
import com.spire.doc.Document;
import com.spire.doc.ToPdfParameterList;
import com.spire.pdf.PdfConformanceLevel;
public class ConvertWordToPdfA {
public static void main(String[] args){
//Create a Document instance and load a Word document
Document doc = new Document("Sample.docx");
//Create a ToPdfParameterList instance
ToPdfParameterList parameterList = new ToPdfParameterList();
//Set the conformance level of the PDF
parameterList.setPdfConformanceLevel(PdfConformanceLevel.Pdf_A_1_A);
//Save the document to PDF/A
doc.saveToFile("ToPdfA.pdf", parameterList);
}
}
Convert Word to Password Protected PDF in Java
You can also encrypt the PDF with password during the Word to PDF conversion. The following are the steps to do so.
- Create an instance of Document class and pass the file path of the Word document to the class’s constructor as a parameter.
- Create an instance of ToPdfParameterList class.
- Set open and permission passwords for the PDF using ToPdfParameterList.getPdfSecurity().encrypt(openPassword, permissionPassword, PdfPermissionsFlags, PdfEncryptionKeySize) method.
- Call Document.saveToFile(filePath, ToPdfParameterList) method to save the Word document to PDF. ```java
import com.spire.doc.Document;
import com.spire.doc.ToPdfParameterList;
import com.spire.pdf.security.PdfEncryptionKeySize;
import com.spire.pdf.security.PdfPermissionsFlags;
public class ConvertWordToPasswordProtectedPDF {
public static void main(String[] args){
//Create a Document instance and load a Word document
Document doc = new Document("Sample.docx");
//Create a ToPdfParameterList instance
ToPdfParameterList toPdf = new ToPdfParameterList();
//Set open password and permission password for PDF
String password = "password";
toPdf.getPdfSecurity().encrypt(password, password, PdfPermissionsFlags.None, PdfEncryptionKeySize.Key_128_Bit);
//Save the Word document to PDF with password
doc.saveToFile("ToPdfWithPassword.pdf", toPdf);
}
}
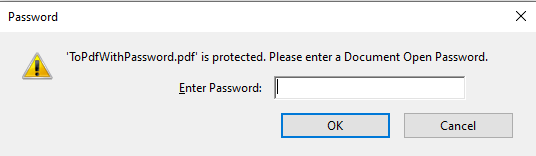
## Conclusion
This article introduces how to convert Word to PDF using the Document.saveToFile() method provided by Spire.Doc for Java. In addition to PDF, you can also use the Document.saveToFile() method to convert Word document to other file formats like Rtf, Html, Odt, Txt, Epub, PostScript, Xml, Svg, XPS and more.
Top comments (2)
Hi there... you forgot to mention an annoying line at the top of the document... this is not usable unless you buy a licence. But you should be able to get rid of the line using spire.doc.free
Spire Doc.
Free version converting word documents to PDF files, you can only get the first 3 page of PDF file.
Upgrade to Commercial Edition of Spire.Doc e-iceblue.com/Introduce/doc-for-ja....