PDF/A (Portable Document Format Archivable) is an archival file format. It can preserve the details of a document and reproduce it in exactly the same way regardless of how long the document has been stored. Sometimes, you may need to convert PDF to PDF/A for long-term document archiving purposes. In this article, I will demonstrate how to convert PDF to PDF/A or PDF/A to PDF using Java.
Add Dependencies
In order to perform the conversions between PDF and PDF/A, this article uses Spire.PDF for Java library. Before coding, you need to include the Jar file of the library in your Java project as a dependency.
Method 1: If you are using maven, you can easily import the JAR file in your application by adding the following code to your project's pom.xml file.
<repositories>
<repository>
<id>com.e-iceblue</id>
<name>e-iceblue</name>
<url>https://repo.e-iceblue.com/nexus/content/groups/public/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>e-iceblue</groupId>
<artifactId>spire.pdf</artifactId>
<version>8.11.8</version>
</dependency>
</dependencies>
Method 2: If you are not using maven, you can download the JAR file from the official website, extract the zip file and then import the Spire.Pdf.jar file under the lib folder into your project as a dependency.
Convert PDF to PDF/A Using Java
Spire.PDF for Java supports converting PDF to various PDF/A formats, including:
- PDF/A-1a
- PDF/A-1b
- PDF/A-2a
- PDF/A-2b
- PDF/A-3a
- PDF/A-3b
The following steps demonstrate how to convert a PDF document to the above listed PDF/A formats:
- Initialize an instance of the PdfStandardsConverter class, and pass the file path of the PDF document to the class constructor as a parameter.
- Convert the document to Pdf/A-1a using PdfStandardsConverter.toPdfA1A() method.
- Convert the document to Pdf/A-1b using PdfStandardsConverter. toPdfA1B() method.
- Convert the document to Pdf/A-2a using PdfStandardsConverter. toPdfA2A() method.
- Convert the document to Pdf/A-2b using PdfStandardsConverter. toPdfA2B() method.
- Convert the document to Pdf/A-3a using PdfStandardsConverter. toPdfA3A() method.
- Convert the document to Pdf/A-3b using PdfStandardsConverter. toPdfA3B() method. ```java
import com.spire.pdf.conversion.PdfStandardsConverter;
public class ConvertPdfToPdfA {
public static void main(String[] args) {
//Initialize an instance of the PdfStandardsConverter class and pass the PDF file path to the class constructor as a parameter
PdfStandardsConverter converter = new PdfStandardsConverter("Input.pdf");
//Convert the PDF document to PdfA1A
converter.toPdfA1A("output/ToPdfA1A.pdf");
//Convert the PDF document to PdfA1B
converter.toPdfA1B("output/ToPdfA1B.pdf");
//Convert the PDF document to PdfA2A
converter.toPdfA2A( "output/ToPdfA2A.pdf");
//Convert the PDF document to PdfA2B
converter.toPdfA2B("output/ToPdfA2B.pdf");
//Convert the PDF document to PdfA3A
converter.toPdfA3A("output/ToPdfA3A.pdf");
//Convert the PDF document to PdfA3B
converter.toPdfA3B("output/ToPdfA3B.pdf");
}
}
The converted PDF/A-1a document:
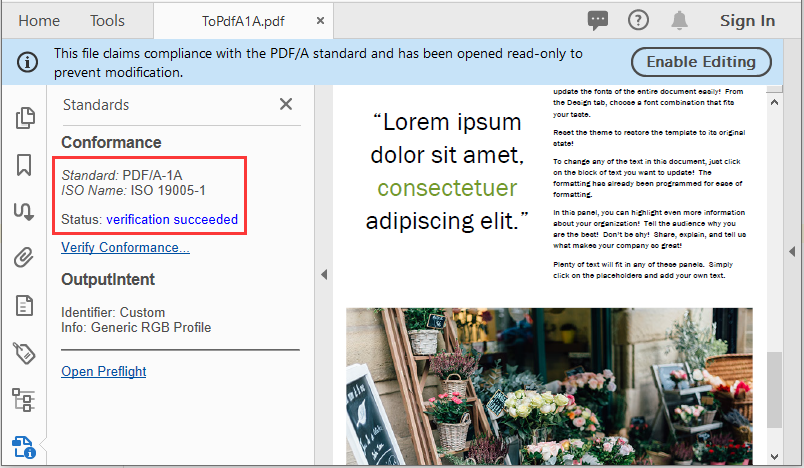
## Convert PDF/A to PDF Using Java
Compared with PDF, PDF/A prohibits some features that are unsuitable for long-term archiving, including JavaScript, audio and video content, encryption, etc. Therefore, you may sometimes need to convert a PDF/A document to a normal PDF document to perform some of the restricted features.
The following steps demonstrate how to convert a PDF/A document to a normal PDF document:
- Initialize an instance of the **PdfDocument** class.
- Load the PDF/A document using **PdfDocument.loadFromFile()** method.
- Initialize an instance of the **PdfNewDocument** class to create a new PDF file.
- Set the conformance level of the new PDF document as PdfCompressionLevel.None using **PdfNewDocument.setCompressionLevel()** method.
- Iterate through all pages in the PDF/A document.
- Add pages of the same page size to the newly created PDF document using **PdfNewDocument.getPages().add()** method.
- Create templates from the pages of the PDF/A document, and draw the templates onto the pages of the new PDF document using **PdfPageBase.createTemplate().draw()** method.
- Save the result document using **PdfDocument.save()** method.
```java
import com.spire.pdf.PdfCompressionLevel;
import com.spire.pdf.PdfDocument;
import com.spire.pdf.PdfNewDocument;
import com.spire.pdf.PdfPageBase;
import com.spire.pdf.graphics.PdfMargins;
import java.awt.geom.Dimension2D;
public class ConvertPdfAToPdf {
public static void main(String[] args) {
//Initialize an instance of the PdfDocument class
PdfDocument doc = new PdfDocument();
//Load the PDF/A document
doc.loadFromFile("output/ToPdfA1A.pdf");
//Initialize an instance of PdfNewDocument
PdfNewDocument newDoc = new PdfNewDocument();
//Set the conformance level of the new PDF document as none
newDoc.setCompressionLevel(PdfCompressionLevel.None);
//Loop through all pages in the PDF/A document
for (PdfPageBase page : (Iterable<PdfPageBase>)doc.getPages())
{
Dimension2D size = page.getSize();
//Add pages to the new PDF document
PdfPageBase p = newDoc.getPages().add(size, new PdfMargins(0));
//Create templates from the pages in the PDF/A document and draw them onto the pages in the new PDF document
page.createTemplate().draw(p, 0, 0);
}
//Save the result document
newDoc.save("NormalPdf.pdf");
}
}
The converted normal PDF document:
Top comments (1)
Thanks for sharing.
Use the website for Pdf to word conversion
Pdf to tiff