A product page is a key component of an e-commerce website or application that displays detailed information about a specific product. It serves as a dedicated page for a single product, providing users with all the relevant details they need to make a purchasing decision.
How to start project to create Product Page :
1. Set up the project:
- Create a new React project using Create React App or your preferred setup method.
- Install any additional dependencies you may need, such as React Router for handling navigation or Axios for making HTTP requests.
2. Create the ProductPage component:
- Create a new file called ProductPage.js and import React at the top.
- Create a functional component named ProductPage.
- Inside the component, return JSX elements to display the product information, such as the product name, description, price, and an image.
- You can also add additional components or sections to enhance the page, such as a product reviews section or related products section.
3. Style the ProductPage component:
- Create a CSS file, such as ProductPage.css, to style the ProductPage component.
- Add styles to position and style the elements on the page, such as applying CSS classes to elements or using a CSS-in-JS solution like styled-components.
4. Add routing:
- If you want to navigate to the product page from another page, you'll need to set up routing.
- If you're using React Router, define a route in your main App component that maps to the ProductPage component.
- Link to the product page from other components or pages using the Link component from React Router.
5. Fetch product data:
- If your product information is stored in a database or an API, you'll need to fetch the data.
- Inside the ProductPage component, you can use the useEffect hook to fetch the data when the component mounts.
- Use a library like Axios or the Fetch API to make an HTTP request to retrieve the product information.
- Set the fetched data in the component's state or use a state management library like Redux to manage the data.
6. Display the fetched product data:
- Once the data is fetched, you can update the JSX in the ProductPage component to display the product information dynamically.
- Use the data from the state or props to populate the product name, description, price, and image.
7. Test and refine:
- Run your React application and navigate to the product page to see the product information rendered.
- Make any necessary adjustments to the styling, layout, or functionality based on your requirements.
- Test the page with different products and ensure that the data is fetched and displayed correctly.
Now, Discuss in details and create product page in react js
Step 1: Set up your development environment
- Install Node.js: Visit the official Node.js website (https://nodejs.org) and download the LTS version suitable for your operating system. Follow the installation instructions.
- Verify the installation: Open your terminal or command prompt and run the command
node -v
to check if Node.js is installed successfully. Similarly, runnpm -v
to check the installed version of npm.
Step 2: Create a new React project
- Open your terminal or command prompt.
- Navigate to the directory where you want to create your React project.
- Run the following command to create a new React project using Create React App: ```
npx create-react-app my-product-page
Replace `my-product-page` with the name you want to give your project.
- Wait for the command to finish creating the project. It will automatically set up a basic React project structure and install the necessary dependencies.
Explore more on React official website [https://react.dev/
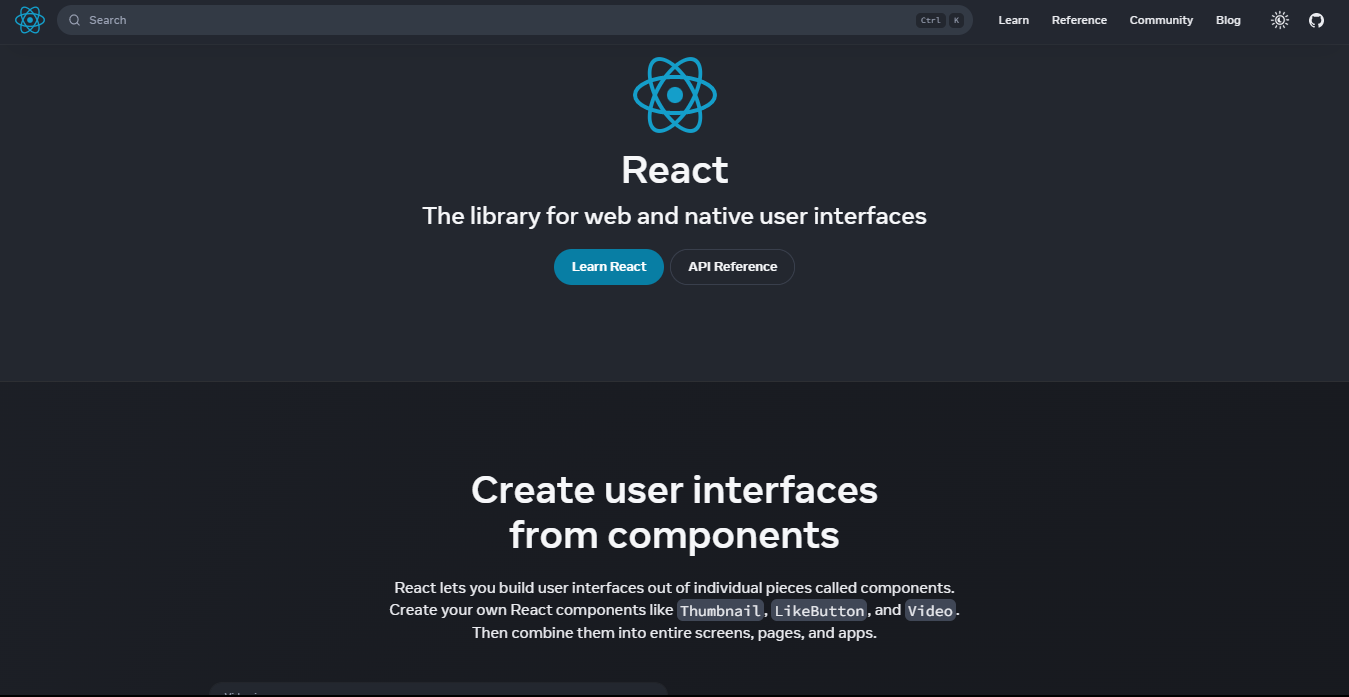](https://react.dev/)
##### Step 3: Navigate to the project directory
- After the project is created, navigate to the project directory by running:
cd my-product-page
Replace `my-product-page` with your project's name.
##### Step 4: Start the development server
- Once you're inside the project directory, run the following command to start the development server:
npm start
- This command will start the React development server and launch your application in a browser. You can view your React app by opening the provided URL (usually http://localhost:3000) in your browser.
##### Step 5: Customize and develop your React app
- Open your code editor and navigate to the project directory.
- Start customizing your React app by modifying the files in the `src` directory. You can edit the `App.js` file to make changes to the main application component.
**`npm` and `npx` are both command-line tools that come with Node.js, but they serve different purposes:**
1. npm (Node Package Manager):
- `npm` is a package manager for Node.js and JavaScript projects.
- It is used to install, manage, and update dependencies for your project.
2. npx (Node Package Runner):
- `npx` is a tool that comes bundled with `npm` (version 5.2+).
- It allows you to run command-line tools and executables without installing them globally.
- `npx` automatically installs the required package temporarily and executes it, ensuring you're using the latest version.
### Add Tailwind CSS to a React project:
Step 1: Install Tailwind CSS dependencies
- Open your terminal or command prompt and navigate to your project directory.
- Run the following command to install the necessary dependencies:
npm install tailwindcss postcss autoprefixer
Step 2: Set up Tailwind CSS configuration
- Create a `tailwind.config.js` file in the root of your project.
- Paste the following content into the `tailwind.config.js` file:
```javascript
module.exports = {
purge: [],
darkMode: false,
theme: {
extend: {},
},
variants: {},
plugins: [],
}
Step 3: Create a PostCSS configuration file
- Create a
postcss.config.js
file in the root of your project. - Paste the following content into the
postcss.config.js
file: ```javascript
module.exports = {
plugins: [
require('tailwindcss'),
require('autoprefixer'),
],
}
Step 4: Import Tailwind CSS styles
- Open the `src/index.css` file in your project.
- Remove the existing content and replace it with the following line to import Tailwind CSS styles:
```css
@import 'tailwindcss/base';
@import 'tailwindcss/components';
@import 'tailwindcss/utilities';
File Structure of Our project is :
my-product-page/
│
├── node_modules/
│
├── public/
│ ├── index.html
│ └── favicon.ico
│
├── src/
│ ├── index.js
├── index.css
│ ├── App.js
│ ├── components/
│ │ └── Product/
│ │ ├── ProductPage.js
│ │ └── ProductItem.js
│ ├── assets/
│ │ └── images/
│ │ └── logo.png
│ └── styles/
│ └── main.css
│
├── postcss.config.js
├── package.json
|── README.md
├── tailwind.config.js
Start Coding....
- Create a new React component for the product page. Let's call it
ProductPage.js
. ```jsx
import React from "react";
export default function ProductPage() {
return (
<>
{/* Remove py-8 /}
{/ Card 1 /}

Featured
iphone XS
4 days ago
The Apple iPhone XS is available in 3 colors with 64GB memory. Shoot amazing videos
12 months warranty
Complete box
Bay Area, San Francisco
$350
{/ Card 1 Ends */}
</>
);
}
2. In your main App.js component, import the ProductPage component and render it.
```jsx
import React from 'react';
import ProductPage from './ProductPage';
const App = () => {
return (
<div>
<h1>My E-commerce App</h1>
<ProductPage />
</div>
);
};
export default App;
- Create the product data in
ProductItem.js
file : ```js
const products = [
{
id: 1,
name: 'iphone XS',
price: 350,
imgurl : 'https://cdn.tuk.dev/assets/templates/classified/Bitmap (1).png',
date : '4 days ago',
desc : 'The Apple iPhone XS is available in 3 colors with 64GB memory. Shoot amazing videos',
warranty : '12 months warranty',
place : 'Bay Area, San Francisco'
// Add more properties as needed
},
{
id: 2,
name: 'iphone XS',
price: 350,
imgurl : 'https://cdn.tuk.dev/assets/templates/classified/Bitmap (1).png',
date : '4 days ago',
desc : 'The Apple iPhone XS is available in 3 colors with 64GB memory. Shoot amazing videos',
warranty : '12 months warranty',
place : 'Bay Area, San Francisco'
// Add more properties as needed
},
// Add more product objects
];
export default products;
Update ProductPage.js file to display the data from ProductItem.js File :
import React from "react";
import products from './product/ProductItem';
export default function ProductPage() {
return (
<>
<div className="bg-gray-100 ">
{/* Remove py-8 */}
<div className="mx-auto container py-8">
<div className="flex flex-wrap items-center lg:justify-between justify-center">
{products.map((item) => (
<div key={product.id} className="mx-2 w-72 lg:mb-0 mb-8">
<div>
<img src={item.imgurl} className="w-full h-44" />
</div>
<div className="bg-white">
<div className="flex items-center justify-between px-4 pt-4">
<div>
<svg xmlns="http://www.w3.org/2000/svg" className="icon icon-tabler icon-tabler-bookmark" width={20} height={20} viewBox="0 0 24 24" strokeWidth="1.5" stroke="#2c3e50" fill="none" strokeLinecap="round" strokeLinejoin="round">
<path stroke="none" d="M0 0h24v24H0z" fill="none" />
<path d="M9 4h6a2 2 0 0 1 2 2v14l-5-3l-5 3v-14a2 2 0 0 1 2 -2" />
</svg>
</div>
<div className="bg-yellow-200 py-1.5 px-6 rounded-full">
<p className="text-xs text-yellow-500">Featured</p>
</div>
</div>
<div className="p-4">
<div className="flex items-center">
<h2 className="text-lg font-semibold">{item.name}</h2>
<p className="text-xs text-gray-600 pl-5">{item.date}</p>
</div>
<p className="text-xs text-gray-600 mt-2">{item.desc}</p>
<div className="flex mt-4">
<div>
<p className="text-xs text-gray-600 px-2 bg-gray-200 py-1">{item.warranty}</p>
</div>
<div className="pl-2">
<p className="text-xs text-gray-600 px-2 bg-gray-200 py-1">Complete box</p>
</div>
</div>
<div className="flex items-center justify-between py-4">
<h2 className="text-indigo-700 text-xs font-semibold">{item.place}</h2>
<h3 className="text-indigo-700 text-xl font-semibold">${item.price}</h3>
</div>
</div>
</div>
</div>
))}
</div>
</div>
</div>
</>
);
}
</code></pre></div><h2>
<a name="look-like-this-" href="#look-like-this-">
</a>
Look like this :
</h2>
<p><img src="https://dev-to-uploads.s3.amazonaws.com/uploads/articles/h3zfhw69aq4jsn1aiea0.png" alt="Output"></p>
<blockquote>
<p>Thank you.❣️</p>
</blockquote>
Top comments (1)
wow this is good thankyou.