Bootstrap is a holy Grail for most of us - it’s the most popular HTML, CSS and most of all JS library in the world. And as a Christmas miracle—Bootstrap v5.3.0-alpha1 has arrived during the holiday break, so… let’s dig inside and check how to use bootstrap in React.
Bootstrap 5 is a popular front-end framework that is widely used for building responsive and mobile-first websites. With the release of Bootstrap 5, developers have access to a wide range of new features and improvements e.g. react-bootstrap, that makes building dynamic web applications much easier. In this tutorial, we will look at how to use Bootstrap 5 in React to build dynamic and responsive web applications.
So let’s start by setting up a React project aka react-bootstrap and installing the required dependencies. Then, we will explore how to use the various components that Bootstrap 5 provides, such as buttons, forms, and modals. We will also learn how to customize the styles of these components to match the design of your web application, import bootstrap dist CSS, import app, react application, import react and…
Throughout the tutorial, we will try to provide examples of how to implement each component and explain the concepts behind them. The aim is to decompose react-bootstrap & bootstrap, that you will have a solid understanding of how to use Bootstrap 5 in React and be able to build responsive and mobile-friendly web applications with ease.
So, if you’re looking to build dynamic and responsive web applications with React, this tutorial is for you! Even if your’re afraid right now how to install bootstrap or import app (not saying to import bootstrap dist CSS) - with our step-by-step instructions and practical examples, you will be able to take your React skills to the next level and start building professional-quality web applications fast and furious. ;)
Introduction
What Bootstrap 5 is and why it’s useful
Bootstrap 5 is a front-end framework for developing responsive, mobile-first websites and applications. It was created by Twitter and is now maintained by a group of developers as an open-source project. Bootstrap 5 provides a set of CSS and JavaScript components, such as buttons, forms, navigation menus, modals, and more, that make it easy to quickly build and style web pages making the same bootstrap classes, bootstrap dist css bootstrap.min.css and hell lot of other things easy like a Sunday morning.
Bootstrap 5 is based on a 12-column grid system that allows developers to create flexible, responsive layouts, even if you’re not ok with e.g. import bootstrap dist css. It also has a number of utility classes for controlling spacing, visibility, and other styling-related properties. In addition, Bootstrap 5 includes a comprehensive set of pre-designed UI components, such as alerts, badges, cards, and carousels, that can be easily customized to meet the needs of your project. We remember when not long ago one of our UI companions - Harry asked us about bootstrap dist css bootstrap.min.css - if import bootstrap dist css vs import react solves the issue. To be honest, even if you want to import react aka import app and install bootstrap, you need just a few bootstrap components.
One of the key benefits of Bootstrap 5 is that it makes it easy to build websites and applications that look good and function well on a variety of devices, including desktop computers, laptops, tablets, and smartphones. This is because Bootstrap 5 is built with responsive design in mind, and it includes a set of CSS media queries (bootstrap dist css bootstrap.min.css) that automatically adjust the layout of your web pages based on the size of the viewport.
Another advantage of Bootstrap 5 is that it provides a consistent look and feel for your website or application, through the bootstrap minified css file. This is because all of the UI components are designed to work together, and they use a common set of styles and patterns. This makes it easy to create a consistent visual style throughout your project, and it also helps to reduce the amount of custom CSS code that you need to write (just npm install).
In conclusion, Bootstrap 5 is a useful tool for web developers because it makes it easier and faster to build responsive, mobile-first websites and applications. It provides a set of pre-designed UI components, a 12-column grid system, and a range of utility classes, all of which make it easier to create beautiful and functional web pages.
What is React.js?
React.js is an open-source JavaScript library for building user interfaces. It was developed and maintained by Facebook. React allows developers to build reusable UI components and manage the state of their applications efficiently within (and not only) react application.
React uses a virtual DOM, which is a lightweight in-memory representation of the actual DOM, to increase the speed and efficiency of updates and rendering. When a user interacts with a React application npm install, the virtual DOM updates, and React then efficiently updates the actual DOM to reflect those changes. There’s no wonder then, that many treat it as the most popular css framework.
React is also highly modular (that’s why you can use bootstrap with react), making it easy to break down complex user interfaces into smaller, reusable components. These components can be reused across multiple pages, making it easier to maintain and scale a React application.
React’s popularity is largely due to its simplicity and flexibility, which makes it a great choice for both small and large-scale web applications. It can be used with a variety of programming languages, including JavaScript, TypeScript, and Dart. It’s also highly compatible with other popular libraries and frameworks, such as Redux, for state management, and React Native, for building mobile applications.
In conclusion, React.js is an efficient, modular, and flexible library for building user interfaces. Its virtual DOM, reusable components, and compatibility with other libraries make it a popular choice for web developers.
Setting up a React.js Project:
Installing Node.js
React.js itself does not require Node.js like bootstrap dist css bootstrap.min.css. However, Node.js is often used in the development of React applications, as it provides a server-side environment for running JavaScript code. Node.js is used to run a local development server, manage dependencies, and build the application for production.
There are Top 3 reasons why Node.js is commonly used with React:
Package Management: Node.js comes with a package manager called npm, which allows developers to easily install and manage the dependencies of their React application.
Development Server: A development server can be set up using Node.js, which is useful for testing and debugging the application during development.
Build Tools: Node.js provides a number of tools for building and compiling React applications, such as Babel and Webpack.
To install Node.js, follow these steps:
Download the latest version of Node.js from the official website (https://nodejs.org/en/download/).
Run the installer and follow the instructions to complete the installation process.
Verify the installation by opening a terminal or command prompt and running the following command: node -v. This should display the version of Node.js that you have installed.
You can also check if npm is installed by running the following command: npm -v. This should display the version of npm that you have installed.
After installing Node.js (not bootstrap cdn or importing bootstrap - commonly misunderstood when npm install), you can use it to manage the dependencies of your React application and run a local development server. You can also use npm to install other tools that you might need for your React projects, such as Babel and Webpack.
Creating a New React.js Project
Create React App (CRA) is a command line tool that makes it easy to create and run a React.js project. It’s the recommended way to create a new React.js project as it sets up a project with a default directory structure and includes all the necessary dependencies and tools through e.g. npm install and npm install bootstrap. Here’s how to create a new React.js project using Create React App:
Install Create React App on your computer by running the following command in your terminal:
npx create-react-app my-app
Change into the newly created project directory:
cd my-app
Start the development server by running the following command:
npm start
Open your web browser and navigate to http://localhost:3000. You should see the default “Welcome to React” message on the page.
You can now start building your React.js application with react-bootstrap packages. You can edit the src/App.js file to begin adding your components and logic.
Here’s a simple example of how to create a component in React.js and render it in your App.js file (useful for bootstrap’s dropdown component and simple theme switcher component as well):
import React, { useState } from "react";
function ExampleComponent() {
// Declare a state variable called "count" and initialize it to 0
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
function App() {
return (
<div>
<ExampleComponent />
</div>
);
}
export default App;
This example creates a component ExampleComponent that displays a count and increments it every time a button is clicked. The component is then rendered in the App component (remember to export the default header and specific components for CSS frameworks when npm install bootstrap and imports bootstrap.
Installing Bootstrap 5 in React project
You can install Bootstrap 5 (Bootstrap CDN) in a Create React App (CRA) project by using npm (importing bootstrap). Here are the steps to install and use Bootstrap 5 in a CRA project:
Open a terminal in your project directory and run the following command to install Bootstrap 5:
npm install bootstrap
Import the Bootstrap CSS and JavaScript files in your src/index.js file. Add the following code at the top of the file:
import "bootstrap/dist/css/bootstrap.min.css";
That’s it! You can now start using Bootstrap 5 classes and components in your React components. For example, to create a button with the Bootstrap classes, you can write:
import React from "react";
function Example() {
return <button className="btn btn-primary">Click me</button>;
}
export default Example;
Note that you can also use other tools like CoreUI for React.js, React Bootstrap, or reactstrap to integrate Bootstrap 5 with React (remember bootstrap cdn?). These tools provide pre-built React components that wrap up Bootstrap 5 classes and deliver native support for bootstrap javascript components, making it easier to use Bootstrap in your React projects.
Customizing Bootstrap 5
The framework is built with Sass, which is a CSS preprocessor that provides additional features like variables, mixins, and functions. You can use these features to customize Bootstrap 5 as many bootstrap classes and create a custom build that meets the specific needs of your project within bootstrap cdn.
To use Bootstrap 5 with Sass in a Create React App (CRA) project, you can follow these steps:
Install the necessary dependencies using npm:
npm install node-sass bootstrap
Create a custom Sass file in your project, for example, src/custom.scss. In this file, you can override the default Bootstrap 5 styles and define custom styles.
Import your custom Sass file in your src/index.js file, for example:
import "./custom.scss";
In your custom.scss file, you can import the Bootstrap 5 Sass files:
// Custom.scss
// Option A: Include all of Bootstrap
// Include any default variable overrides here (though functions won't be available)
@import "~/bootstrap/scss/bootstrap";
// Then add additional custom code here
sh
or
// Custom.scss
// Option B: Include parts of Bootstrap
// 1. Include functions first (so you can manipulate colors, SVGs, calc, etc)
@import "~bootstrap/scss/functions";
// 2. Include any default variable overrides here
// 3. Include remainder of required Bootstrap stylesheets (including any separate color mode stylesheets)
@import "~bootstrap/scss/variables";
@import "~bootstrap/scss/variables-dark";
// 4. Include any default map overrides here
// 5. Include remainder of required parts
@import "~bootstrap/scss/maps";
@import "~bootstrap/scss/mixins";
@import "~bootstrap/scss/root";
// 6. Optionally include any other parts as needed
@import "~bootstrap/scss/utilities";
@import "~bootstrap/scss/reboot";
@import "~bootstrap/scss/type";
@import "~bootstrap/scss/images";
@import "~bootstrap/scss/containers";
@import "~bootstrap/scss/grid";
@import "~bootstrap/scss/helpers";
// 7. Optionally include utilities API last to generate classes based on the Sass map in `_utilities.scss`
@import "~bootstrap/scss/utilities/api";
// 8. Add additional custom code here
Bootstrap 5 UI components for React.js
There are several popular UI component libraries for React that are built using Bootstrap 5 as a foundation. That’s our favorite one: https://coreui.io/react/docs/getting-started/introduction/ :) Some of the most popular include:
CoreUI for React CoreUI for React is a comprehensive UI library for React that extends Bootstrap 5 and includes additional components and features. It is maintained by a team of professionals and offers enterprise-level support (yes, that’s we :))
React-bootstrap: React-bootstrap is a library that provides Bootstrap 5 components as React components with bootstrap stylesheet, react component and npm install react. So you can easily add bootstrap and export default post to prove installed bootstrap. It is designed to work seamlessly with React, allowing developers to build dynamic user interfaces with ease.
Reactstrap: Reactstrap is another library that provides Bootstrap 5 components as React components that enables to import individual components with a bootstrap stylesheet. It is designed to be simple, lightweight, and easy to use, making it a popular choice for React developers.
Each of these libraries offers a variety of pre-built UI components that can be easily integrated into a React project. They can save time and effort by providing a solid foundation for building UI components (but also module bundler, main sass file and built in bootstrap classes) and can help ensure consistency and maintainability across an application.
CoreUI for React.js
What is CoreUI for React.js?
CoreUI for React is a UI components library for building user interfaces in React.js projects. It provides a collection of pre-built components, such as buttons, forms, tables, and more, that can be used to quickly build user interfaces. The library is based on the popular CoreUI framework, which provides a set of styles and UI bootstrap components for building responsive, modern websites and web applications.
React components are built using the latest web technologies and best practices, ensuring that they are optimized for performance and accessibility within bootstrap library. So you don’t need to search react courses , as each react bootstrap component is designed to be easily customizable, so you can easily change the look and feel of your application to match your brand and design guidelines. Additionally, the library provides a set of tools and plugins to help you streamline your development process and quickly build complex user interfaces. Javascript file, post component, import popper or search for other javascript frameworks? Not this time… :)
CoreUI for React extends Bootstrap 5 and provides all of the Bootstrap 5 components, as well as additional components not found in Bootstrap, such as a DateRangePicker, MultiSelect, and DataTables. This makes it a versatile and comprehensive solution for building user interfaces in React.js projects.
In addition, CoreUI for React is maintained by a team of professionals, ensuring that the library is always up-to-date with the latest web technologies and best practices. The library also offers enterprise-level support, making it an ideal choice for large-scale projects and organizations that require a high level of reliability and support.
Overall, CoreUI for React is a well-maintained and comprehensive UI components library that extends Bootstrap 5 and provides additional components and tools to help you quickly build user interfaces in React.js projects. Whether you’re starting a new project or adding new features to an existing one, CoreUI for React is a great choice for building high-quality, responsive user interfaces for any react app.
Installing CoreUI for React.js
To install CoreUI for React in a Create React App (CRA) project, you can use npm. Here’s an example of how to install and use CoreUI in a CRA project:
Open a terminal in your project directory and run the following command to install CoreUI for React:
npm install @coreui/react
Import the CoreUI CSS file in your src/index.js file. Add the following code at the top of the file:
import "@coreui/coreui/dist/css/coreui.min.css";
You can now start using CoreUI components in your Create React App project.
Using CoreUI for React.js in your create react apps
To use CButton and CModal components from CoreUI for React in a Create React App project, follow these steps:
Import the components in your React component file:
import {
CButton,
CModal,
CModalBody,
CModalFooter,
CModalHeader,
CModalTitle,
} from "@coreui/react";
Use the components in your component’s JSX code:
import React, { useState } from "react";
import {
CButton,
CModal,
CModalBody,
CModalFooter,
CModalHeader,
CModalTitle,
} from "@coreui/react";
function App() {
const [visible, setVisible] = useState(false);
return (
<>
<CButton onClick={() => setVisible(!visible)}>
Launch demo modal
</CButton>
<CModal visible={visible} onClose={() => setVisible(false)}>
<CModalHeader onClose={() => setVisible(false)}>
<CModalTitle>Modal title</CModalTitle>
</CModalHeader>
<CModalBody>Woohoo, you're reading this text in a modal!</CModalBody>
<CModalFooter>
<CButton color="secondary" onClick={() => setVisible(false)}>
Close
</CButton>
<CButton color="primary">Save changes</CButton>
</CModalFooter>
</CModal>
</>
);
}
export default App;
In this example, the useState hook is used to manage the visible state, which controls the visibility of the CModal. The setVisible function toggles the state between true and false when the button is clicked. The CModal component is then passed the visible state value as the show prop, so that it is only displayed when visible is true.
And that’s it! You can now use the components in your Create React App project. Keep in mind that you may need to customize the appearance of these components to match the look and feel of your application.
React-Bootstrap
What is React-Bootstrap?
React Bootstrap is a UI components library for building user interfaces in React.js projects. It provides a set of pre-built components, such as buttons, dropdown menu, forms, tables, and more, that can be used to quickly build user interfaces and add bootstrap and import reactdom. So adding bootstrap, import popper, import button or button component is easy as the library is based on the popular Bootstrap framework, which provides a set of styles and UI components for building responsive, modern websites and web applications.
React Bootstrap components are built using the latest web technologies and best practices, ensuring that they are optimized for performance and accessibility with all bootstrap’s built in classes on board. The components are designed to be easily customizable, so you can easily change the look and feel of your application to match your brand and design guidelines. Additionally, the library provides a set of tools and plugins to help you streamline your development process and quickly build complex user interfaces.
Overall, React Bootstrap package provides a comprehensive solution for building user interfaces in React.js projects, whether you’re starting a new project or adding new features to an existing one. With its collection of pre-built components, tools, and plugins, React Bootstrap can help you reduce the time and effort required to build high-quality, responsive user interfaces.
Installing React-Bootstrap
To install React Bootstrap package in a Create React App (CRA) project, you can use npm. Here’s an example of how to install and use React Bootstrap library in a CRA project:
Open a terminal in your project directory and run the following command to install React Bootstrap package:
npm install react-bootstrap bootstrap
Import the Bootstrap CSS file in your src/index.js file. Add the following code at the top of the file:
{/* The following line can be included in your src/index.js or App.js file */}
import 'bootstrap/dist/css/bootstrap.min.css';
sh
You can now start using React Bootstrap components in your React app.
**Using React-Bootstrap in your create react apps**
Here’s a step-by-step guide to integrating React Bootstrap into a Create React App project, and using its Button, Modal, and Form.Control components, with the useState hook for controlling the modal’s visibility:
import React, { useState } from 'react';
import Button from 'react-bootstrap/Button';
import Modal from 'react-bootstrap/Modal';
function App() {
const [show, setShow] = useState(false);
const handleClose = () => setShow(false);
const handleShow = () => setShow(true);
return (
<>
Launch demo modal
Modal heading
Woohoo, you're reading this text in a modal!
Close
Save Changes
</>
);
}
export default App;
In this example, the useState hook is used to manage the show state, which controls the visibility of the Modal. The setShow function toggles the state between true and false when the button is clicked. The Modal component is then passed the show state value as the show prop, so that it is only displayed when show is true.
Note that, like with CoreUI for React.js, you will need to import the individual components you want to use from the react-bootstrap package.
**Reactstrap**
**What is Reactstrap?**
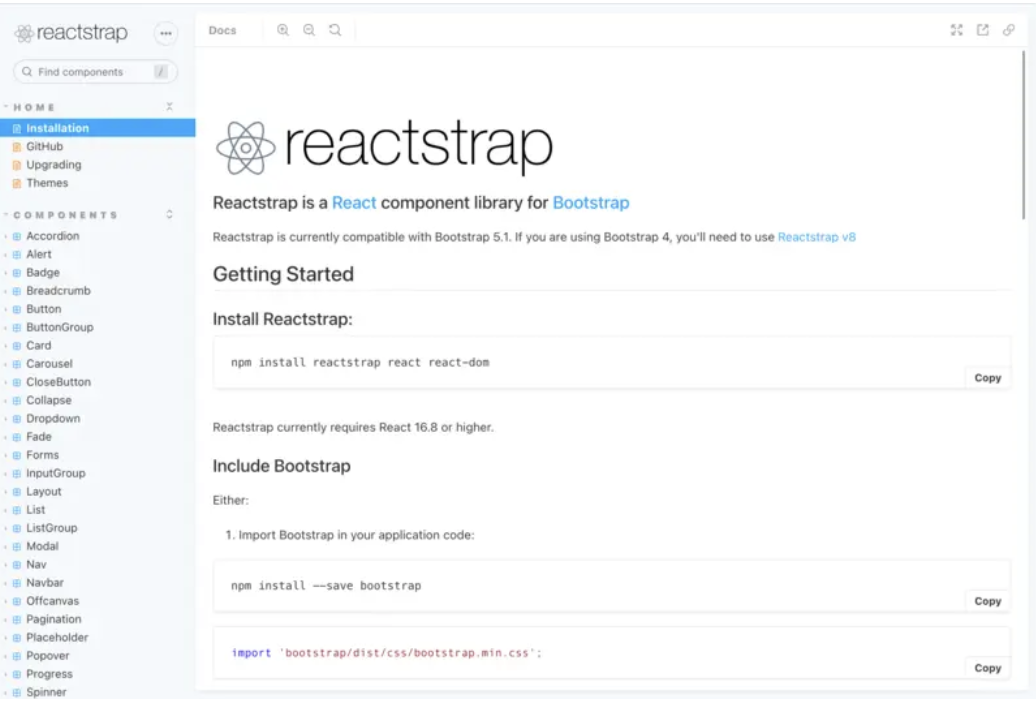
Reactstrap is a UI components library for building user interfaces in React.js projects. It provides a set of pre-built components, such as buttons, dropdown menu, forms, tables, and more, that can be used to quickly build user interfaces. The library is built on top of Bootstrap, which is a popular framework for building responsive, modern websites and web applications.
Reactstrap components are designed to be easily customizable, so you can easily change the look and feel of your application to match your brand and design guidelines. But remember this won’t ever be vanilla bootstrap design. ;) Even if you would import reactdom - no chance, just add bootstrap. The library provides a set of tools and plugins to help you streamline your development process and quickly build complex user interfaces. Additionally, Reactstrap components are built using the latest web technologies and best practices, ensuring that they are optimized for performance and accessibility.
Overall, Reactstrap provides a comprehensive solution for building user interfaces in React.js projects adding bootstrap. With its collection of pre-built components, tools, and plugins, Reactstrap can help you reduce the time and effort required to build high-quality, responsive user interfaces. Whether you’re starting a new project or adding new features to an existing one, Reactstrap can help you quickly and easily build the user interfaces you need.
**Installing Reactstrap**
To install Reactstrap in a Create React App (CRA) project, you can use npm. Here’s an example of how to install and use Reactstrap in a CRA project:
Open a terminal in your project directory and run the following command to install Reactstrap:
npm install reactstrap
You can now start using Reactstrap components in your React components
Note that you will need to import the individual components you want to use from the reactstrap package. Also, Reactstrap depends on Bootstrap’s CSS, so you’ll need to include the Bootstrap CSS file in your project as well. You can do this by adding the following code to your src/index.js file:
import 'bootstrap/dist/css/bootstrap.min.css';
**Using Reactstrap in your create react apps**
Here is an example of how you can use Button and Modal from reactstrap in a Create React App project with the use of useState hook:
Import the necessary components in your React component file:
import React, { useState } from 'react';
import { Button, Modal, ModalHeader, ModalBody, ModalFooter } from 'reactstrap';
Define the state for controlling the modal using useState hook:
const [modal, setModal] = useState(false);
Create a function to toggle the modal:
const toggle = () => setModal(!modal);
Use the imported components to render a button that opens the modal and the modal itself:
return (
<>
Click Me
Modal title
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad
minim veniam, quis nostrud exercitation ullamco laboris nisi ut
aliquip ex ea commodo consequat. Duis aute irure dolor in
reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla
pariatur. Excepteur sint occaecat cupidatat non proident, sunt in
culpa qui officia deserunt mollit anim id est laborum.
Do Something
{' '}
Cancel
</>
);
With this code, you should have a functioning modal triggered by a button in your Create React App project using reactstrap components and the useState hook and import reactdom to add bootstrap.
Benefits of Using Bootstrap 5 and UI Components Libraries in React.js
Bootstrap 5 is a popular CSS framework that provides a set of pre-designed UI components and layout styles. Using Bootstrap 5 in a React.js project has dozen of benefits, but below seem to be the most significant:
**Speed and efficiency:** Bootstrap 5 provides a wide range of pre-designed components that can be easily imported and used in your React.js project, saving you time and effort. This allows you to focus on developing the business logic and functionality of your application, rather than spending time designing and implementing UI components.
**Consistent look and feel:** Bootstrap 5 provides a consistent design language that ensures that your application has a consistent look and feel, which can improve the user experience. This can help to increase user engagement and improve the overall perception of your application.
**Cross-browser compatibility:** Bootstrap 5 is designed to work seamlessly across all modern browsers, including Internet Explorer 11. This means that your application will have a consistent look and feel, regardless of the browser that is being used.
**Responsive design:** Bootstrap 5 is built with a mobile-first approach, meaning that the UI components are optimized for small screens first, and then scale up to larger screens. This makes it easy to create responsive applications that look great on any device.
In addition to using Bootstrap 5, you can also use UI components libraries such as CoreUI for React, React-Bootstrap, or Reactstrap. These libraries provide additional UI components and customizations, and make it even easier to build and style React.js applications.
By using Bootstrap 5 and UI components libraries in a React.js project, you can benefit from a fast and efficient development process, a consistent look and feel, cross-browser compatibility, and responsive design. This can help you to create high-quality, user-friendly applications that deliver a great user experience.
**Resources:**
Official Bootstrap 5 Documentation
Official React.js Documentation
Official CoreUI for React.js Documentation
Official React Bootstrap Documentation
Official reactstrap Documentation
Top comments (0)