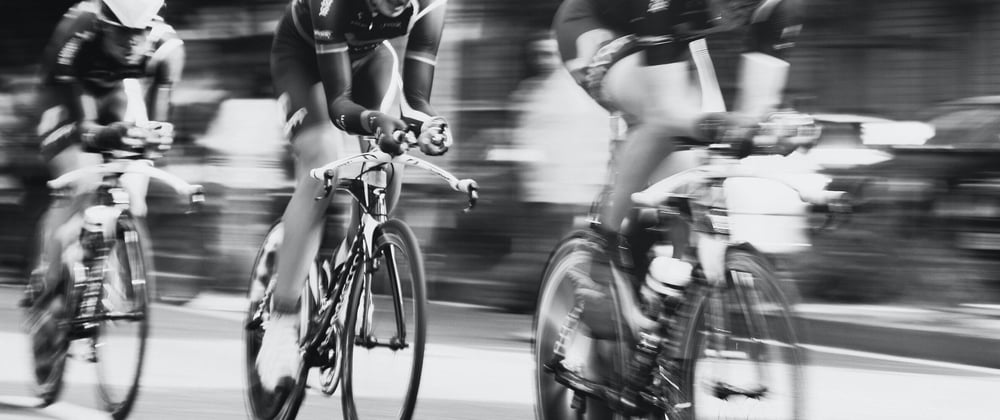
Convenient and useful techniques to reduce the lines of code and pace up your Dev Work!
In our daily tasks, we get to write functions su...
For further actions, you may consider blocking this person and/or reporting abuse
min:
Math.min(...numbers)
max:
Math.max(...numbers)
Exactly, not sure of the advantage of using reduce over these common functions.
I'd go further and agree with this thread and follow up video that advise against using reduce in nearly every situation. It's often used when a simpler or more readable alternative is available, and leads to harder to read, harder to maintain code. As proven with this example above.
Well, of course above methods seems simpler for finding min/max but one should not underestimate the power of reduce in JavaScript. There are several technique where you can solve problems mostly in one reduce statements only.
But in this case a helper method that does the full job exists so why bother with reduce for this use case? I admittedly do not use it enough but just saying
I've made a simple benchmark, it seems that reduce() is faster than Math.max()
dev-to-uploads.s3.amazonaws.com/up...
That's awesome @liquorburn . I have added the fastest one only.
It's actually the spread operator that's taking the time there. Though admittedly, there aren't many cases you'd have a hardcoded list of values to find the max of, it's worth pointing out.
dev-to-uploads.s3.amazonaws.com/up...
Thanks for reminding this tip @martinmuzatko .
Please what tool is used to make this code snippet embedment on the pages?
These are just code test only. Use 3 backticks before and after your code. Check the preview.
About the logical operators in 5, you should always be aware of their defined behavior:
a && b
yields b if a is truthy, otherwisefalse
a || b
yields the first value of a, b that is truthyThis means that
a || b && c
will return a if it a is truthy. If you are using a tool like prettier, you can just use brackets everywhere and let it figure out where they can be removed safely.I second that @lexlohr ! Nice suggestion to keep in mind.
Cool
Have a github too so other can collab.
Also fix the bug in
Thanks @manoharreddyporeddy . Astonishing Idea of GitHub. Will create the repo and update the link in the post itself for further collaboration.
Nevertheless, what error are you pointing out in above snippet?
can be
OR
Reason is a number can only be < or > or === 97.
There can't be a 4th option.
Hope it's clear now.
Will wait for your github link.
Oh I got that one now! Updated.
Here is the link for the GitHub repo, github.com/kapilraghuwanshi/quick-.... Need to finalize the repo structure and PR etc, if you have suggestions please add to that repo.
Looks like the classic FizzBuzz test
Looks more like “Hey, I know a bit of JS” rather than tips really. Let’s start with one, can you think of a way to make the matrix in a bit more efficient manner (hint: 2 iterations less), so it starts looking like a tip that will make me more efficient as advertised
Well thanks for the comment @snigo . And I didn't want to sound like “Hey, I know a bit of JS”, if I did, share the points/sentences, will try to fix them.
However, as I pointed out earlier in the post that - there can be more than one approaches to the same problem, so feel free to share yours here.
Enjoy:
Now, number two: what's going to be the sum of an empty array in your example?
How about these two?
If we talk about efficiency, then we first need to figure out
.map
or.from
:map
: maps over elements returning new array 👎from
: mapFn maps over elements in place 👍So
from
, which leaves us with the question what we're going to create our array from, right? So if we compare:...it will boil down to the question what's more efficient to create
Array(3)
or{ length: 3 }
, and given arrays in JS are just objects it really comes to the number of properties we need to create for the object. How many properties doesArray(3)
have? (hint: 4) How many properties does{ length: 3 }
have? (hint: 1)I hope this will clear things a bit
Awesome and detailed explanation @snigo . ✌🏻
I have created GitHub Repo, feel free to contribute to that.
Thanks!🤗
Never use ternary operators to replace more than a single
if...else
. Otherwise, it quickly becomes unreadable and error-prone. The example from your post is an excellent proof of this :'DComment should warn that if you use || to provide some default value, you may encounter unexpected behaviors if you consider some falsy values as usable (e.g., '' or 0).
You're making me relive production drama right here :(
check more functions like this
devsmitra.github.io/javascript-qui...
I would advise to not use nested ternary operators as it is considered a code smell
Can you share the disadvantages with code snippets ?
Sorry I don't have the time nor the patience at the moment but I can at least detail my answer :
Simply, if you think about the Signal-to-Noise ratio, nesting ternary operator will more often lead to error when sigh treading and ask more energy to be understood for the sake of a very few line of code.
In this particular case, writing a more explicit comparison will be better for the whole understanding of the code flow. with "ifs" or juste split it in more lines of ternary attribution.
Also, if you should write half a dozen comparison for the attribution of a variable, you should put it in a specific function that will be named accordingly and this will greatly improve this part of the code.
For more information, you can look in the ESLint documentation for this specific code smell : eslint.org/docs/rules/no-nested-te...
Also thank you for your post !
Unfortunately, in the real life, data are much more complex, I think if you can share about how to composite functions then it should be very helpful.
My tips
Good article man, I just have a couple of comments further to the above:
6: I believe this approach only works if you are using strings or numbers, not objects
17: Earlier today, I learned that parseInt can also be used in this way. The second argument defines the number system base
Just add "javascript" after your opening code block backticks for syntax highlighting.
Thanks @kiranrajvjd for the tip. Updated to make it more colourful!
Dear Kapil Raghuwanshi,may I translate your article into Chinese?I would like to share it with more developers in China. I will give the original author and original source.
Sure @qq449245884 , go ahead and share the GitHub link too, besides the article to contribute.
ok,than you very much!
@qq449245884 you can share the link for your article here!
Pretty gorgeous recap, definitly a must have for hackatons & dailycoding, thanks a lot !
Thanks @youpiwaza . I have written for the same aim.
Your post is well-written! I just launched a site bitcoinforecast.io and I developed it myself using ES6 JS. Your post covers the strategies I used to built the app. Keep up the great work Kapil.
Thanks @joshternyak . 😀
Thanks bro...
All my pleasure @mohammadmesbaulhaque !
really amazing and helpful, thank u!!
2D matrix: Array(5).fill(Array(5).fill(0))
@raulcg More crispier one!👍🏻