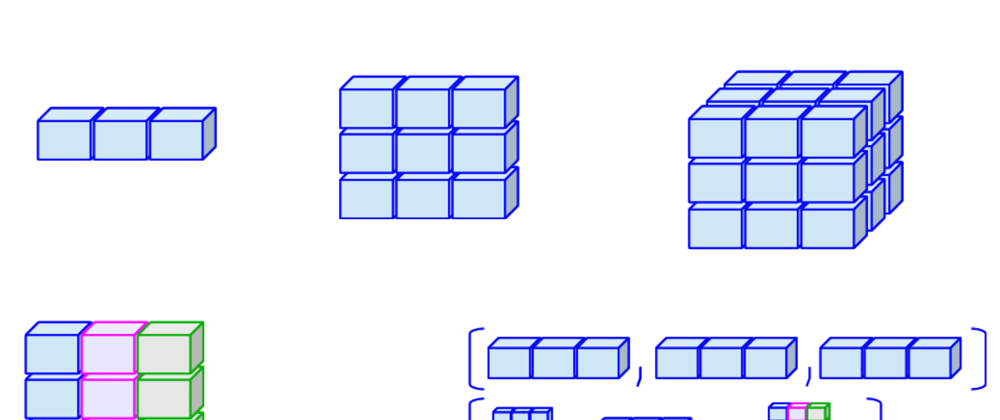
Hello Programmers,
Most of us are scared of algorithms, and don't ever start to learn it. But we shouldn't be scared of it. An algorithm is just s...
For further actions, you may consider blocking this person and/or reporting abuse
Wow. I'll learn this.
Glad that you liked it.
You have an error in LSP value. It must be
[0, 0, 0, 3, 5, 0]
instead of[0, 0, 0, 1, 2, 0]
You actually have a wrong LPS algorithm. Here's the right one:
There was a small typo at line 8. Wrote "i" instead of "1".
Thanks for pointing.
LPS value is actually correct. It is [0, 0, 0, 1, 2, 0]
I had a small typo in LPS algorithm. I added "i" instead of "1".
Thanks for pointing.
Yeah, that all because of the type. It changed the value and it also causes infinite loop.
Nevertheless that was a pleasure to read your article!
Hey please can you tell me which website did you use to add those code snippets?
Those code snippets are not added via any website. I wrote the article in markdown along with the code, and the snippets' design is native to dev.to platform itself.
You got the O(n^2) complexity wrong. O(n^2) is quadratic complexity, a special case of polynomial complexity O(n^k).
Exponential complexity is O(2^n) and none of your example algorithms have exponential complexity.
Yes, you are right. Thaks for this. I will fix it soon.
wouldnt life be amazing if everything was O(1)
Wonderful post, thank you.
Radix sort improvement to handle negative integers, in my own writing:
In binary search, you have an infinite loop for non-existent member search.
Correct while loop exit term should be:
Very well explain Appreciated your efforts... Really Nice.
Website
This is gold, thank you for sharing this!
Thank you Bruno. 🙏
I am glad that you found this useful. 😊
Very nice article, thanks
Thanks for your kind words Alejandro. It means a lot. 😊
Isn’t it a bit cheating to say that in the radix sort algorithm k x m = n?
I mean, it is true but if the largest number in the array is at least propotional to the size of the array (which is very likely) then k becomes Ω(log m) (lower bound) and in this case for being more fair with the other algorithms, if we define n to be the length of the array we will get something close to O(n log n).
Just putting it here for those who would confuse and think that radix sort is absolutely better then quick sort or merge sort. It depends on the data and the free space you have.
Super post! So much great content shared here. Algorithms are such a pain to learn and really hold some developers back from applying for jobs.
Thank you Andrew. 😊
This motivates me to write more.
Hmm, you just let me know I don't have to buy a course to learn algorithms, I love your content, eyes on you bro, thanks for making me happy.
This is the best comment I have ever got.
Thanks for being so kind, brother. 🙏
You literally made my day. 😊
Have you thought about making a website with algorithms explained in javascript?
Hey Amr,
Thank you so much for your interest. I haven't thought about it, but it seems to be a really great idea.
I will definitely think about it. Thank you for the suggestion man.
Awesome one.
Thank You, Youdiowei.
This really means a lot.
This is great 👌
Thank You for the kind words Brinth.
Thanks a lot decker.
Hey Swastik,
Awesome article, any chance you can share how you created the visuals for the tutorial. TIA
shouldn't KMP algorithm return the index?
cryto