How To Conduct End-To-End Testing With MailSlurp
If you send email from an application, you probably have tests that ensure your code is attempting to send that email. But do you have a right way of testing whether that email got sent? MailSlurp is an awesome free tool that helps you do that. It allows you to easily generate random inboxes to send email to and then confirm delivery through its API.
In this post, we will use the MailSlurp Javascript SDK to write an end-to-end test for a node function that sends an email. We’ll be using SparkPost to send the email. If you aren’t already sending email, here’s a good place to start (for free). For our test we’ll use Jest, but any framework should work just fine.
Our code will be using a few es2015 features, so be sure to be running a version of node that supports them (version 6.4.0 or above should be fine). We’ll be installing dependencies using npm version 5.6.0.
All the code for this demo can be found here.
Getting Set Up
Alright, let’s get started! The first step is to get set up with a MailSlurp account. It’s quick and free: Sign up for MailSlurp. Next, log in to your dashboard and grab your API key.
Once you have your API Key, we can get started with the test setup. Make sure you have these dependencies in your package.json – here’s what ours looks like, using the SparkPost client library:
"dependencies": {
"sparkpost": "^2.1.2",
"mailslurp-client": "^0.1.1514850454",
"jest": "^22.1.2"
}
And run npm install
Here is our full package.json. Normally packages like Mailslurp client and jest should go under “dev-dependencies”, but we kept it simple for this demo.
Now from the top of our test file, we require Mailslurp-client and our sendEmail
code:
// test file: mailslurp.test.js
const MailslurpClient = require('mailslurp-client');
const sendEmail = require('./sendEmail.js'); // our SparkPost email function
You would import whatever function you use to send email.
Right after, we initialize the MailSlurp client. This is also a good spot to store your API key in a variable.
// initialize the MailSlurp Client
const slurp = new MailslurpClient.InboxcontrollerApi()
// Sign up at MailSlurp.com to get one of these for free.
const slurpKey = process.env.MS_APIKEY;
We used environment variables to access ours; if you store credentials a different way, that’s ok too. Our slurpKey variable will be the first parameter we pass to every MailSlurp client method.
Next step is to create an inbox we can send to with the MailSlurp client’s createRandomInboxUsingPOST
method:
describe('SendEmail Tests', () => {
let address;
let inboxId;
beforeAll(() => {
// Creates the inbox and set address & inboxId for use in tests
return slurp.createRandomInboxUsingPOST(slurpKey)
.then(({ payload }) => {
address = payload.address; // the email address we will be sending to
inboxId = payload.id; // UUID used to identify the inbox
});
});
/* tests will go here */
});
The important things here are:
- Execute this code before you run your tests
- Store both address & id from the response as variables, so you can send to and access your inbox
Now for the actual test we want to send an email (sendEmail()
function for us) and then use the MailSlurp client’s getEmailsForInboxUsingGET
method to get the email from the inbox:
test('Email delivers', () => {
// increase the default jest timeout to wait for delivery
jest.setTimeout(60000);
// pass address given to us by MailSlurp
return sendEmail(address)
.then(({ total_accepted_recipients }) => {
// make sure SparkPost accepted the request
expect(total_accepted_recipients).toEqual(1);
// Get the list of emails from the inbox
return slurp.getEmailsForInboxUsingGET(slurpKey, inboxId,
{
minCount: 1, // minimum number of emails to wait for, default wait time is 60s
})
.then(({ payload }) => {
// console.log(payload) // uncomment this to see the full email
expect(payload).toHaveLength(1);
const [email] = payload;
expect(email.subject).toEqual('MailSlurp Test Email');
});
});
});
Email delivery can take more than a few seconds, so make sure your test waits around long enough for the email to be delivered. In the code snippet above, we change the timeout threshold to handle that. Note that we are passing address
to our sendEmail
function, and then passing inboxId
to getEmailsForInboxUsingGET
.
For this test we first asserted that MailSlurp returned an array of emails with a length of 1 (we only sent one email). Then to make sure it was the email we sent, we asserted that its subject was ‘MailSlurp Test Email’ as defined in sendEmail.js
That’s it! Run the test however you usually do. Ours is set up with npm scripts in the package.json, which runs with npm test
.
Next Steps
There is plenty of room for more assertions in our example test. When using an email delivery service like SparkPost in combination with complex HTML templates, an email’s body can get pretty complicated. To make assertions about an email’s actual content, we suggest using an advanced email body parser. Once you are able to extract the HTML/text content from the body, you could easily use snapshot tests to ensure you are sending the content you want.
If this was interesting to you, you should check out the rest of MailSlurp features. You can reach us on Twitter for any questions. And you can also send 15,000 emails a month for free on SparkPost! Special thanks to Jack Mahoney for building MailSlurp and answering my questions for this post.
–Jose
The post End-To-End Email Testing With MailSlurp appeared first on SparkPost.
Top comments (3)
😕
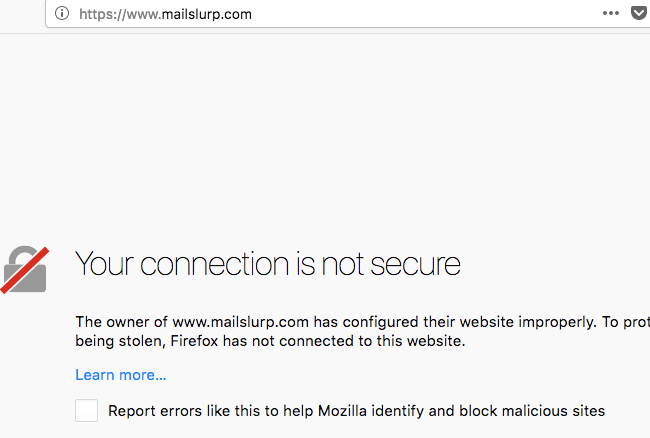
Oh no! I’m able to connect through https on chrome/safari, on the road and don’t have firefox on my phone. Is this still a problem?
It's fixed now! 😎