My first post in dev.to.
I have learnt how to automate the creation of nice html tables using pretty-html-table package. This package is embedding very nicely with other packages used to send emails.
In this post I am going to cover:
- Sending Email using SMTP
- Convert pandas dataframe to pretty HTML table
- Sending the email with HTML content
Setup SMTP to send Email
Python comes with the built-in smtplib module for sending emails using the Simple Mail Transfer Protocol (SMTP). I have used Gmail SMTP server to send emails, but the same principles apply to other email services. Google’s Gmail SMTP server is a free SMTP service which anyone who has a Gmail account can use to send emails.
- Gmail SMTP server address: smtp.gmail.com
- Gmail SMTP username: Your Gmail address
- Gmail SMTP password: Your Gmail password (Unique 12 letter password). Click here to set your password
- Gmail SMTP port (TLS): 587 ```
from smtplib import SMTP
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_mail(body):
message = MIMEMultipart()
message['Subject'] = 'Top 5 Economies of the World!'
message['From'] = '<sender>@gmail.com'
message['To'] = '<receiver>@gmail.com'
body_content = body
message.attach(MIMEText(body_content, "html"))
msg_body = message.as_string()
server = SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(message['From'], 'Unique password')
server.sendmail(message['From'], message['To'], msg_body)
server.quit()
### <u>Create a Dataframe</u>
import pandas as pd
def get_gdp_data():
"""
GDP data
:return:
"""
gdp_dict = {'Country': ['United States', 'China', 'Japan', 'Germany', 'India'],
'GDP': ['$21.44 trillion', '$14.14 trillion', '$5.15 trillion', '$3.86 trillion', '$2.94 trillion']}
data = pd.DataFrame(gdp_dict)
return data
### <u>Convert pandas dataframe to HTML table</u>
Pretty HTML table package integrates very well with other python packages used to send emails. Just add the result of this package to the body of the email.
from send_email import send_mail
from get_data import get_gdp_data
from pretty_html_table import build_table
def send_country_list():
gdp_data = get_gdp_data()
output = build_table(gdp_data, 'blue_light')
send_mail(output)
return "Mail sent successfully."
send_country_list()
Done!
Octocat will take you to my GitHub repository...
[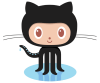](https://github.com/siddheshshankar/pyHTML)
Top comments (10)
Thanks for sharing..
Can we send list of mail address in message['To'] parameter?
I tried that getting below error('list' object has no attribute 'encode')
Do you have solutions for this to send mail to multiple users at once?
Thank you for this post about my library. It is meant to be used by as many people as possible so I appreciate you sharing your experience in this platform.
Do not hesitate to star the library as well, it is helpful for us that build content for the whole community.
github.com/sbi-rviot/ph_table
Best,
Sbi-rviot
Thank you soooo much! I had no idea that pretty_html_table existed and was struggling with it so badly
Welcome
This package eliminates dependency on HTML and CSS to format tables.
Hi,
Thanks for sharing this code. I want to add multiple tables using exec stored procedure. I am able to get the desired result for just one table, but struggling to get the others in the same email body. Can you please help?
Can we add headers here as we do in HTML using header tags and any idea on sending two different Dataframes in the same email?
Yes, you can add all type of tags and send 2 or more dataframes in a single email.
Thank you. I was able to do it later :)
Thanks, exactly what I need
Thanks for sharing.