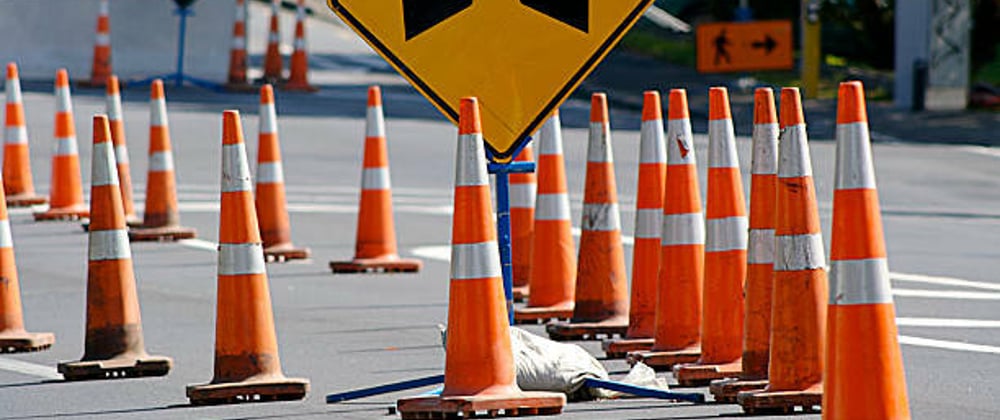
One of the features that is neccessary in a single page application as it pertains to the authentication or its security is the ability to conditio...
For further actions, you may consider blocking this person and/or reporting abuse
Just a little feedback on some things that you might want to correct in your article so that people are not misled.
The isUserAuthenticated function will never return true. It will return "false" or "undefined". Instead of what you have:
const isUserAuthenticated = () => {
if (!authState.token) {
return false;
}
};
You might want to consider simply:
const isUserAuthenticated = () => !!authState.token;
Which will always return a boolean value.
Also, where you're checking if the user is authenticated in your useEffect you have your logic backwards. If the user isn't authenticated you're sending them to the dashboard, not the login page.
You have this:
React.useEffect(() => {
// checks if the user is authenticated
authContext.isUserAuthenticated()
? router.push("/")
: router.push("/dashboard");
}, []);
Which should be:
React.useEffect(() => {
// checks if the user is authenticated
authContext.isUserAuthenticated()
? router.push("/dashboard")
: router.push("/");
}, []);
Dang!!!
Thank you so much for pointing this out. I'll make the necessary change. Thank you once again for your feedback man!
you still haven't updated your article
All done!
Thank you for reminding me
Yghhh
thank you for this article.
I'm glad you found it helpful
Hhh
Nice Article, But why we are not using session ?
Thanks Hidayt!
Funny enough. This was when I first laid my hands on auth in Next.js. Now that I've been accustomed to it. I'm using cookies and session with
getServerSideProps
Hopefully, I'll have an article about it and share it Herr, soon.
Hi Caleb, do you have any open source repo demonstrating your use of cookies and session with
getServerSideProps
?Hi Kehinde,
At the moment, No. But, I'm experimenting with cookies and
getServerSideProps
currently.I'm using nookies (a Next.js cookie helper package) to parse cookies on the client and server.
Perhaps and when it turns out well. I'll share what I learned.
Maybe I am wrong, but is it safe to store token in localStorage? AFAIK localStorage can be accesed by 3rd party scripts :/
You're absolutely correct.
Storing JWT in localStorage isn't the perfect solution. You can try keeping the token in a cookie.
Whatever works best for everyone's use-case.
I feel like useEffect is the wrong hook to use. You're rendering all your components, then checking if the user is authenticated.
Wouldn't it be better just to:
!authContext.isUserAuthenticated() && router.push("/")
Outside of the useEffect?
can we use useLayoutEffect() hook. which runs before the rendering part.
youtu.be/sRDUOd1IkS8
Thank you for this article, but I just got confused a little bit, you didn't use localstorage.getItem at all!, this means whenever the user land on the page he will be not authorized even though the token exists in the localstorage? am I wrong?
Hi Muhnnad,
I'm glad you found it helpful. I'm not using the
getItem
method here because all I'm doing here is to check if the user's auth token is in localStorage.If it is, authenticate the user. If it isn't, re-route them to the login page.
Hence, the need for this snippet, and the one below, in the use Effect hook.
isUserAuthenticated won't work becasue next js have by default ssr. Here's simple solution that put jwt in cookie. it will work otherwise , it will show undefined.
Ok
Ok
Ok
Ok
Ok
Have covered session here.
Handle Protected Route using Session
Great! I see that you're using next-auth here too.
My own case would be for an external backend. Thank you for sharing though.
It works with both cases internal and external backend by using next-auth
Oh! Great!
If you have hundreds of pages, there will be a lot of code duplication with this approach
What would the best approach look like?
You can create a new function inside your auth-context.js like this.
And then use it as a wrapper to your pages.
Example in MyApp component, like this:
Alternatively, you can also add this to any individual page.
Thank you for sharing this @liv_it