Unlike arrays, it's always been tricky to get the object length. Well no more!
Object.keys return an array of all the object's enumerable proper...
For further actions, you may consider blocking this person and/or reporting abuse
Btw, Chrome displays not enumerable properties in the console. It just shows them slightly differently - not as bright as enumerable ones.
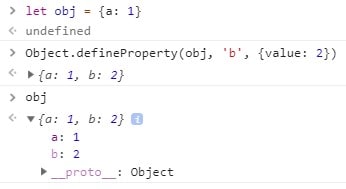
Wooo... that's interesting! good to know, thanks for sharing that! Let me add this my notes 👍
Semantics: A JS object is a collection of properties, keys that map to their respective values, with keys that can only be strings or symbols. It doesn't have a length.
Similar to asking "What is the length of your car?" and giving the answer "The length of my car is 14, because it has 4 doors, a trunk, 6 windows, and 4 wheels".
(Simplified example.)
Using
Object.keys().length
orObject.getOwnPropertyNames().length
gives the length of the list of enumerable keys (or all property names for the latter), not of an object.Not judging though. I just enjoy being explicit/intentional with wording when I can. Good article and thanks for sharing. :)
Something you could add: Using an object as a key calls
.toString()
, which will make all object keys conflict on the key"[object Object]"
. ES6'Map
works with objects but uses their memory address as keys, so an exact copy of an object will have a different key (no deep comparison, for efficiency).Totally fair assessment! I guess I always just associated length to the size, since length is the property being called for array or strings. So naturally when I was thinking of getting the size of an object, I automatically default to referring it to
length
as well 😅 I guess that's why Lodash opted for size and not length. This is probably the best thing with sharing an article. It always opens up a conversation. I'm glad you shared your comment! Now, I learned something. Thank you for explaining with such clarity! 😊You're absolutely right. Semantics, to define the meaning of things, is great to have conversations about if you find the right people.
Meaning is flexible, after all, until something is defined. Exploring this creates new understanding and perspectives, which works wonders to break out of black/white thinking.
Maybe
length
is the wrong term, but it's perfectly reasonable to define an object'ssize
the way it's done in this post.Thanks for chiming in Daniel! Now I can see why Lodash chose
size
instead of length 😅Notice also that JavaScript itself is choosing
size
for types likeSet
orMap
:size
is a more generic (and mathematical) name that suits more needs better, but nitpicking and pedantry aside,length
is perfectly a understandable (and I keep using it forSet
s andMap
s until I remember that's wrong).Clarifying meaning isn't pedantic.
Using
size
andcapacity
instead oflength
on data structures with dynamic lengths was done before JS.There was probably a discussion somewhere, where they argued for/against both.
Python implements
len()
as a function with the magic method__len__
precisely to have one consistent way of retrieving an intuitive "length".I enjoyed this article! "How to get an Object Length" really undersells it. Didn't know about the
enumerable
property - or the weird thing with symbols. Great piece of writing.How would you use these features? I can understand
enumerable
being useful to get some level of 'privacy'/encapsulation into an JavaScript object. Are symbols with keys the same thing but more so? Are there any other uses?Thanks David for the kind feedback! In regards to
symbols
, from the readings I gathered, it seems to serve one main purpose: create property keys without risking name collisions (since symbols are always unqiue). I have not personally used symbols in my daily programming yet. if you know of any other use cases, please do share. Might be an interesting one to cover in a future post, have to dig into it a bit further 😊Great writeup!
The enumerable and Symbols parts were especially excellent.
This made me play around with your example code a bit and I have a question.
How should I handle this case where I don't want the symbol to get counted?
edit:
Came up with this, other solutions?
If you only want the enumerable properties without the symbols. The
Object.keys
method should just work, since it doesn't include that. If I misunderstood your questions, please follow up 🙂All enumerable properties, including ones with Symbols as keys.
Excluding all non-enumerable properties.
an object with:
1 enumerable property with string as key,
2 non-enumerable properties with a string as key,
1 enumerable property with Symbol as key
1 non-enumerable properties with Symbol as key.
Should return 2 as total length. (1 for string key and 1 for Symbol key)
Wait.
At first you said that Object.keys don't return hidden properties.
In the end of the article you use this method to count a number of object's properties. I guess you should use Object.getOwnPropertyNames(animal).length instead of Object.keys(animal).length.
Which part? Hmm...i don't think i see it 😲
Here's your code in the end of the article to count total amount of an object's properties.
Let's take a look at this object:
This object has two properties, but your code above will return 1. So my thought was that it's better to replace
Object.keys
withObject.getOwnPropertyNames
to return correct amount of an object's total properties.Deeply covered, nice!
As a workaround, what about Object.values().length ?
I think
Object.values
andObject.keys
will have the same length, as they both consider only enumerable properties:Docs for Object.values
true story...
I'm getting
3
for thelength
of bothkeys
andvalues
. The arrays are["a", "b", "c"]
and["somestring", 42, false]
, respectively.Yup, that works too! Now that i think of it, i should have cover those examples too 😅 next time 😜
Great write-up!
I've used
getOwnPropertyNames
in the past, along with recursive calls on an object's prototype, to get all of the properties that object has and that it inherited.Yup that's great use case to use
getOwnPropertyNames
. I think the key is knowing what you want and picking the right tool for it. Thanks for sharing your use case! 👍