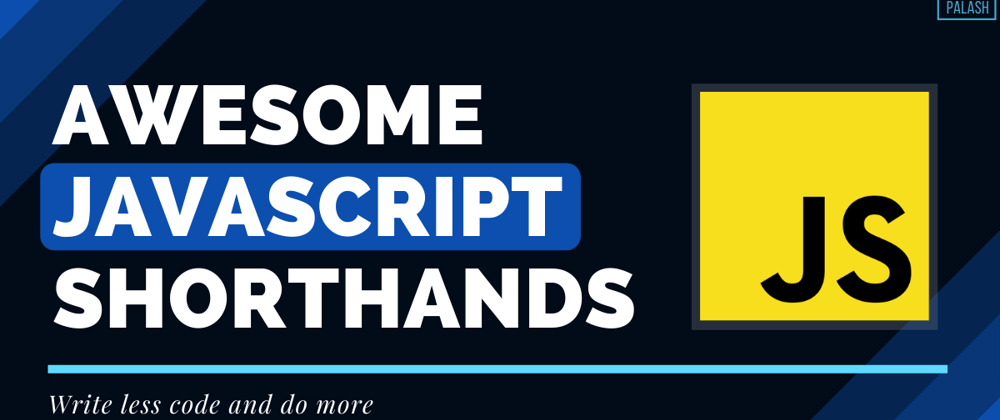
Hi everyone 👋
Today I wanted to share with you 10 awesome JavaScript shorthands that will increase your speed by helping you to write less code an...
For further actions, you may consider blocking this person and/or reporting abuse
If you don't need the
this
context you can shorten even further when you use arrow functions.in your example
it can be shortened to
The params of your forEach (item, index) will get passed to the console.log function :)
if you are using custom functions to pass into things like
.forEach
you can go from this example:to this
Hope that helps someone!
Edit: adding example of printMessage
Final note: doing this kind of extraction/abstraction of functionality can help better describe what's happening in your code without needing to add code comments and it can help you unit test your code later on with more context. When you build a larger system, you could have these type of helper functions extracted out of your main code as well. 🚀
Thanks for sharing! 😊
I think this shorthand:
If you are logging it can be simplified like this:
Thanks for your feedback. ❤️
I have already mentioned that shorthand in the "7. Arrow Functions" section. I hope that is fine.
Happy Coding!
Generally for .. of works fine, but was not supported on some older browsers on Android. So, referring to my personal experience, in a real application it can help to use for .. in to get better compatibility.
Thanks for sharing shorthands
Thank you so much. Glad you liked it! 😃
Thanks for sharing your feedback! 😃
Never seen the
+
prefix one, I look forward to adding this to a PR to confuse everyone. Cheers.Glad you liked it. Have fun adding it to a PR! 😄
Good one 🐨
Thank you so much! 😃
Thanks for sharing!
me too