Django is a powerful and versatile web framework that allows you to build web applications quickly and efficiently. In this blog post, I will show you how to get started with Django web development and create a simple blog app.
First, you need to install Django on your system. You can use pip, a package manager for Python, to do that. Open a terminal and run the following command:
pip install django
This will install the latest version of Django on your system. You can check the version by running:
django-admin --version
Next, you need to create a project folder and a Django project. A project is a collection of settings, apps, and files that make up your web application. To create a project, run the following command in your terminal:
django-admin startproject blog_project
This will create a folder called blog_project with some files inside it. The most important file is manage.py, which is used to run various commands for your project.
Now, you need to create an app inside your project. An app is a component of your web application that handles a specific functionality, such as blog posts, users, comments, etc. To create an app, run the following command in your terminal:
python manage.py startapp blog_app
This will create a folder called blog_app with some files inside it. The most important file is models.py, which is used to define the data models for your app.
A data model is a representation of the data that you want to store in your database. For example, for a blog app, you might want to store information about blog posts, such as title, content, author, date, etc. To define a data model, you need to use the Django ORM (Object-Relational Mapper), which is a layer of abstraction that allows you to interact with the database using Python code.
To define a data model for blog posts, open the models.py file in your blog_app folder and add the following code:
from django.db import models
from django.contrib.auth.models import User
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
author = models.ForeignKey(User, on_delete=models.CASCADE)
date = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
This code defines a class called Post that inherits from models.Model, which is the base class for all Django data models. The class has four attributes: title, content, author, and date. Each attribute is an instance of a field class that defines the type and constraints of the data. For example, title is a CharField that can store up to 100 characters, content is a TextField that can store unlimited text, author is a ForeignKey that references another model (User), and date is a DateTimeField that automatically sets the current date and time.
The str method is a special method that returns a human-readable representation of the object. In this case, it returns the title of the post.
After defining the data model, you need to register it with the admin site. The admin site is a built-in feature of Django that allows you to manage your data through a web interface. To register the Post model with the admin site, open the admin.py file in your blog_app folder and add the following code:
from django.contrib import admin
from .models import Post
admin.site.register(Post)
This code imports the Post model from models.py and registers it with admin.site.
Now, you need to create the database tables for your data model. To do that, you need to run two commands: makemigrations and migrate. Makemigrations creates migration files that store the changes you made to your data model. Migrate applies those changes to the database. Run the following commands in your terminal:
python manage.py makemigrations
python manage.py migrate
This will create and apply the migrations for your Post model.
At this point, you have successfully created a Django project, an app, and a data model for blog posts. You can now run the development server and access the admin site. Run the following command in your terminal:
python manage.py runserver
This will start the development server on http://127.0.0.1:8000/. To access the admin site, go to http://127.0.0.1:8000/admin/ and log in with the username and password you created when you ran migrate for the first time.
You should see something like this:
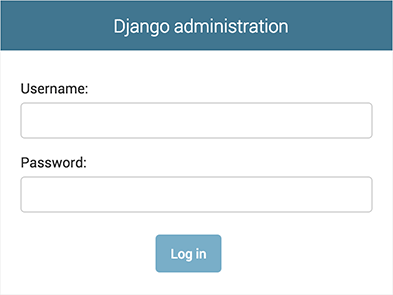
You can click on Posts to add, edit, or delete blog posts using the admin interface.
Congratulations! You have just created a simple blog app using Django web development.
Top comments (0)