How to generate a QR Code in C#:
- Create or Open a visual studio Project
- Install IronQR Library
- Write a Code to create QR codes.
- Style Our QR Code, Add a Logo, Set dimensions, and change the Color
- Read the QR Code
Quick Response (QR) codes have become integral to our digital lives, facilitating seamless information sharing and accessibility. Creating QR codes in C# is a common requirement in various applications, from marketing to inventory management. This article will guide you through the steps of creating QR codes in C# using IronQR and provide insights into key aspects of the process.
Understanding QR Codes
Before diving into the C# implementation, let's briefly understand QR codes and how they work. QR codes represent two-dimensional barcodes capable of storing diverse data, encompassing text, URLs, contact details, and other information types. They consist of black squares arranged on a white square grid, and their structure allows for efficient encoding and decoding of information.
Choosing a C# Library
Several libraries are available for generating QR codes in C#. One popular choice is the IronQR library, which is a QR Code library. It supports a variety of QR code formats and provides AI models to read and generate QR codes. There are some other .Net barcode dll available that provide QR code functionality, but they are not specialized, unlike IronQR.
What is IronQR:
IronQR is a powerful C# QR Code library for .NET applications, providing developers with a user-friendly API for effortless QR code generation and reading. IronQR ensures flexibility in integrating QR codes into diverse projects by supporting various output formats, including images (PNG, JPEG, GIF, TIFF, SVG, BMP), streams, and PDFs. IronQR ensures broad cross-platform compatibility, spanning across various .NET versions and supporting languages like C#, F#, and VB.NET. This adaptability caters to a diverse range of application types, encompassing web technologies like Blazor and WebForms, mobile platforms including Xamarin and MAUI, desktop frameworks such as WPF and Windows Forms, as well as console applications.
IronQR establishes itself as a reliable and effective solution by providing features like advanced machine learning for QR code detection, styling options, fault tolerance, and compatibility with Windows, Linux, and macOS environments. IronQR stands as a trusted and efficient solution, emphasizing accuracy, speed, and ease of use for millions of engineers worldwide.
Now, Let' start our tutorial by Creating or opening an existing visual studio Project.
Create or Open a visual studio Project:
Create or open existing Projects. The project can be of any type including Blazor, MAUI, ASP.NET MVC, ASP.NET Web APIs, ASP.NET Web Forms, Windows Forms, and console applications. In this example, I will be creating a Console Application. The same code work for all project type. The steps for creating a new project in Visual Studio 2022 (you may use any Visual Studio Version ) is as:
- Open Visual Studio 2022.
- File > New > Project...
- Choose "Console App" under the "C#" category. Click Next.
- Enter a name for your project (e.g., "QR Code Generator").
- Choose .NET Framework, I have chosen .NET 8. You may choose any as per your requirements.
- Click "Create." The Project will be created as shown:
Install IronQR Nuget Package:
We need to install the IronQR Nuget Package in our project to use its functionalities. Follow the steps to install the Library.
- In the top menu, go to Tools > NuGet Package Manager > Package Manager Console to open the Package Manager Console.
- In the Package Manager Console, use the Install-Package command to install the package. ```
Install-Package IronQR
Press Enter to execute the command, and NuGet will download and install the package along with its dependencies.
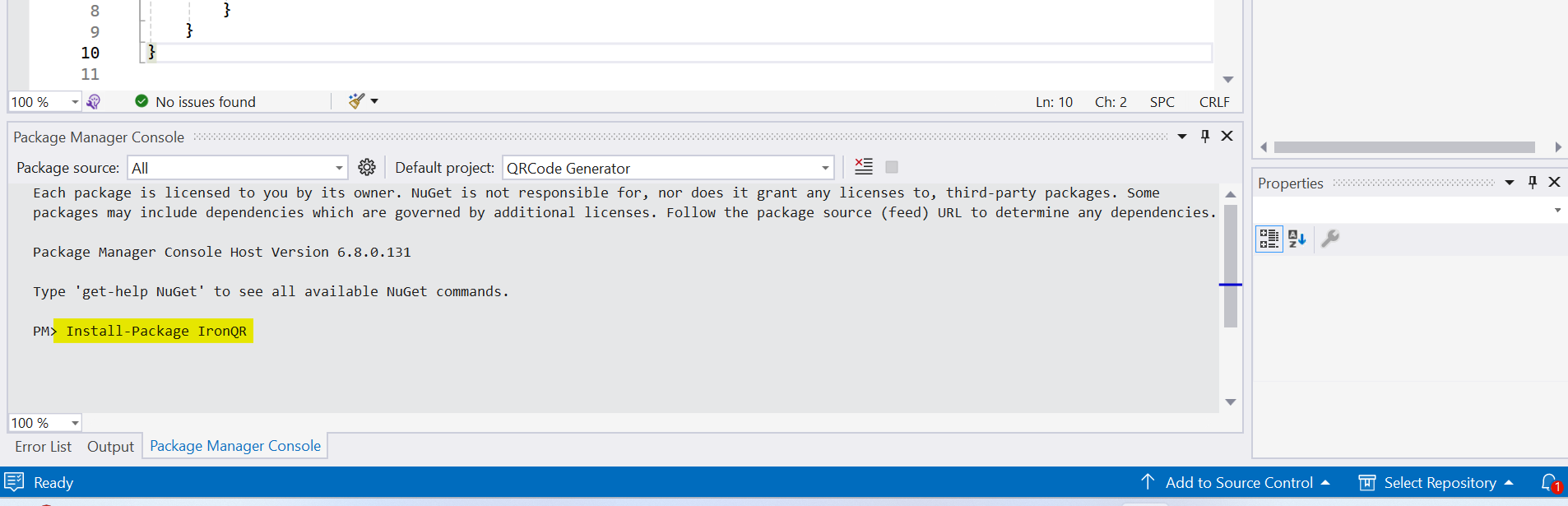
##Write a Code to Generate a QR code
Now, let's create a simple C# program to [generate a QR code](https://ironsoftware.com/csharp/qr/examples/generate-qr-code/) using the IronQR library. First, import the necessary namespaces:
using IronQr;
using IronSoftware.Drawing;
Next, you can define a method to generate a QR code and save it as an image file:
static void Main(string[] args)
{
QrCode myQr = QrWriter.Write("My First QR");
// Save QR Code as a Bitmap
AnyBitmap qrImage = myQr.Save();
// Save QR Code Bitmap as File
qrImage.SaveAs("qrCode.png");
}
The above C# Code demonstrates the generation and saving of a QR code using an IronQR library. It starts by creating a QrCode instance through a QrWriter class or method, embedding the text "My First QR." The QR code is then converted into a bitmap represented by an object named qrImage. Finally, this bitmap is saved as a PNG image file named "qrCode.png" using the SaveAs method.
###Output QR Code image file
The QR code image is as:
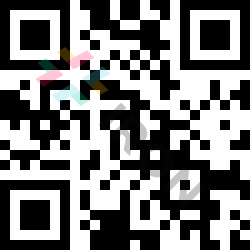
##Generate Advance QR Code:
We can [style our QR code](https://ironsoftware.com/csharp/qr/examples/generate-styled-qr/) by setting its dimensions, adding a logo, changing color, etc by using Iron QR.
Add the following namespace:
using IronQr;
using IronSoftware.Drawing;
using Color = IronSoftware.Drawing.Color;
Write the following code to style your QR Code.
static void Main(string[] args)
{
// Set QR options
QrOptions options = new QrOptions(QrErrorCorrectionLevel.High);
// Create a QR Code object
QrCode myQr = QrWriter.Write("My Advance QR Code", options);
// Fancy style options
AnyBitmap logoBmp = new AnyBitmap("logo.jpg");
QrStyleOptions style = new QrStyleOptions
{
Dimensions = 300, // px
Margins = 10, // px
Color = Color.MediumVioletRed,
Logo = new QrLogo
{
Bitmap = logoBmp,
Width = 100,
Height = 100,
CornerRadius = 2
}
};
// Save QR Code as a Bitmap
AnyBitmap qrImage = myQr.Save(style);
// Save QR Code Bitmap as File
qrImage.SaveAs("myStyledQRCode.png");
}
The above code example illustrates the generation and styling of a QR code using an IronQR library. QR QR code options are set, specifying a high error correction level. Subsequently, We have set the QR Code Square grid color to "Medium Violet Red", set the dimension to 300, and the margin to 10. Finally, the styled QR code is saved as a bitmap, and the resulting image is saved as a PNG file named "myStyledQRCode.png." This example showcases a more advanced usage, incorporating styling features and logo customization to enhance the visual presentation of the QR code.
###Output QR Code:
The generated QR code image is:
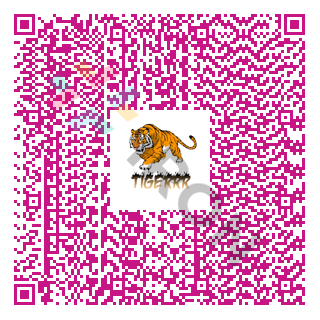
##Read QR Codes:
IronQr also provides methods for [reading QR codes](https://ironsoftware.com/csharp/qr/examples/read-qr-code/). Let's write a code to read QR codes.
static void Main(string[] args)
{
var inputBmp = AnyBitmap.FromFile("myStyledQRCode.png");
// Load the asset into QrImageInput
QrImageInput imageInput = new QrImageInput(inputBmp);
// Create a QR Reader object
QrReader reader = new QrReader();
// Read the Input an get all embedded QR Codes
IEnumerable<QrResult> results = reader.Read(imageInput);
foreach(QrResult qrResult in results)
{
Console.WriteLine(qrResult.Value);
}
}
The above C# example demonstrates the example of reading and extracting QR codes from an image. In the Main method, an image file named "myStyledQRCode.png" is loaded as an AnyBitmap using the FromFile method. Subsequently, the loaded bitmap is utilized to create a QrImageInput object, and a QrReader instance is created. The Read method is then invoked on the reader to extract QR codes from the input image, and the results are enumerated through a loop. For each detected QR code, its value is printed to the console. This example highlights the process of reading QR codes from an image file, completing the end-to-end cycle of generating and later interpreting QR codes using the library.
###Output:
The QR Code value printed on the console as:
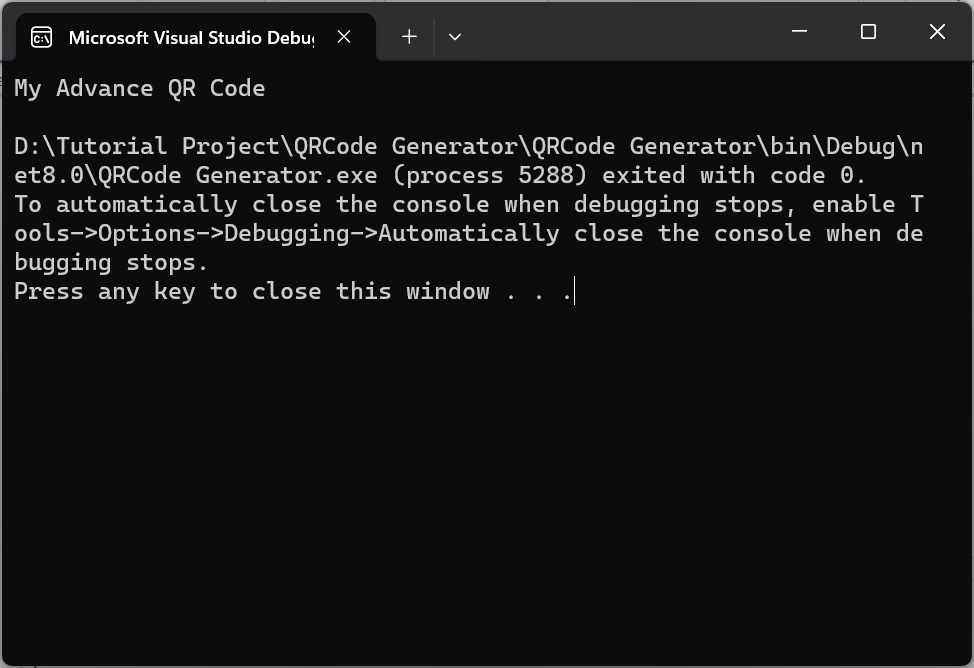
##Conclusion:
In conclusion, the IronQR library serves as a comprehensive and versatile solution for QR code generation and manipulation in C#. From the basic generation of QR codes to advanced styling options, such as adding logos and customizing dimensions and colors, IronQR empowers developers to seamlessly integrate [QR code functionality](https://ironsoftware.com/csharp/qr/tutorials/csharp-qr-code-generator/) into various .NET applications. The library's cross-platform compatibility and support for multiple .NET versions, coupled with features like advanced machine learning for QR code detection, underscore its reliability and efficiency. With the ability to read QR codes from images, IronQR completes the cycle of QR code utilization. Overall, IronQR stands as a trusted and user-friendly tool, emphasizing accuracy, speed, and ease of use for developers worldwide. IronQR offers a free trial for users to evaluate the product before opting for a [commercial license](https://ironsoftware.com/csharp/qr/licensing/) suited to their specific requirements with a money-back guarantee.
For those working with barcodes, [IronBarcode](https://ironsoftware.com/csharp/barcode/) is an excellent tool to consider. It offers robust features for reading and creating barcodes, making it a reliable choice for any barcode-related tasks. With its user-friendly interface and extensive documentation, IronBarcode simplifies the integration of barcode functionality into your projects.
Top comments (1)
FYI, IronBarcode requires a license ($500+) to use outside of your development environment. I'd recommend using something else if you're just trying to make some QR codes.