Hi there!
In this subsequent series of Data Structures, we’ll take on the Palindrome Number challenge. We are given a function that accepts integer x as arguments and returns a boolean value. The function should return true if the arguments passed to it is a palindrome, and false if not.
As usual, we'll cover this in TypeScript but you should be able to follow along regardless of the programming language you use.
Here’s the starter code:
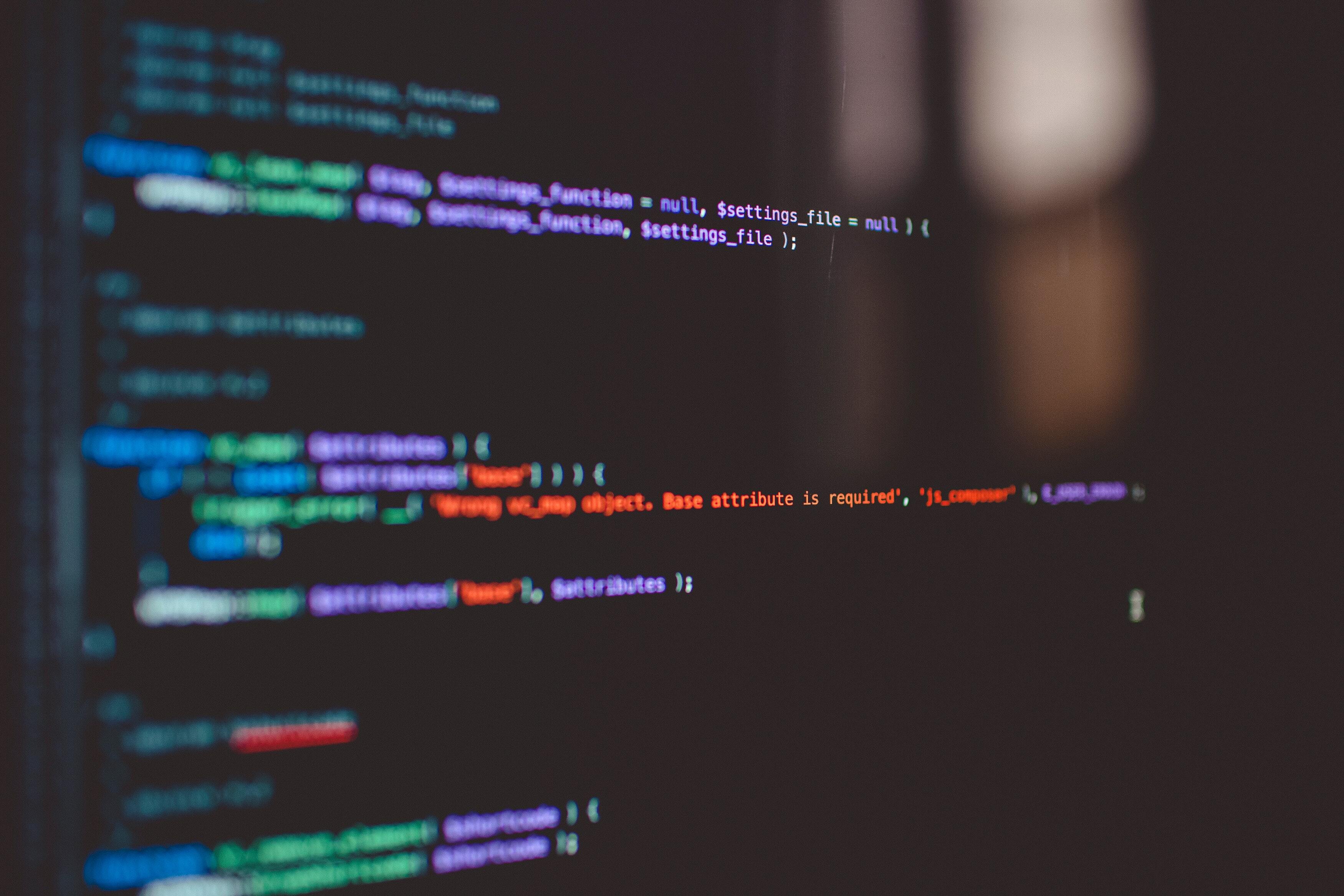
Let’s break down the problem bit by bit. The pattern will be a little bit similar to the reversed vowels of a string. Let’s start by initializing to variables. originalNumber
and reversedNumber
. The originalNumber
variable will hold the original value of x while the reversedNumber
will hold the reversed value of x.
function isPalindrome(x: number): boolean {
let originalNumber = x;
let reversedNumber = 0;
};
Next, we’ll have to loop over x and update the reversedNumber
by adding the last digit of x to it on each iteration.
Suppose the value of x is 125. On the first iteration, the number 5 will be added to reversedNumber
which initially has the value of 0. So after the first iteration, its value should be 5. On the second iteration, its value should be updated to 52, and eventually 521.
Let's use a while loop to achieve this:
function isPalindrome(x: number): boolean {
let originalNumber = x;
let reversedNumber = 0;
while(x > 0) {
let digit = x % 10;
}
};
In the above code, we created a while loop that runs as long as x is greater than zero. We then initialized a variable digit that holds the remainder of x when it's divided by 10. This will filter out the last digit of the variable x. So if x holds the value 125, the last digit (5) will be stored in the digit variable simply because 125/10 will give you 12.5, 5 being the remainder and also the last digit of parameter x.
Now that we have a way of getting the last digit of x on each iteration, we have to store them in the variable reversedNumber
.
function isPalindrome(x: number): boolean {
let originalNumber = x;
let reversedNumber = 0;
while(x > 0) {
let digit = x % 10;
reversedNumber = reversedNumber * 10 + digit
x = Math.floor(x / 10)
}
return originalNumber === reversedNumber;
};
In the above code, we multiply the reversedNumber
by 10 and the last digit of the parameter x to it to maintain their positional values. We then updated the value of x by dividing the current value of x by 10 and ensuring there is no remainder. This operation effectively removes the last digit of x on every iteration. Lastly, we return a boolean from comparing the reversedNumber
to the originalNumber
.
In summary, this code reverses the digits of the input integer and checks if the reversed integer is equal to the original integer to determine whether the input is a palindrome or not.
Top comments (0)