Decision-making in any programming language is as significant as it is in our lives. In a programming language, decision-making is determining the order of statements to be executed depending on a specific condition or repeating the execution of statements until a specified condition is met.
Conditional statements are a crucial part of decision-making in programming, and they are also referred to as decision-making statements. They are simple programming language commands that control a program’s execution flow and decide which part of the program should get executed. More precisely, they carry out different actions depending on whether the given condition is true or false.
In this article, we shall discuss different types of Python conditional statements with their syntaxes, flowcharts, and examples. So, without further ado, let us begin.
Python Conditional Statements
Python has five different conditional statements, namely if, if-else, else-if (elif), nested-if, and elif ladder. Let us discuss each of these statements in detail.
1. If Statement
Python ‘if’ statement decides whether to execute certain statements or not. If a condition specified in the ‘if’ statement is true, it executes the code present inside the ‘if’ block; otherwise, it will not. The rest of the code outside the ‘if’ block will be executed, regardless of whether the condition in ‘if’ is true or false.
Syntax:
if (expression):
statement(s)
Flow chart:
Example:
x, y = 34, 98
If (y>x):
result = "x is less than y."
print(result)
print("Welcome to TechGeekBuzz!")
Output:
In the above example, we have taken two variables, x, and y, with values 34 and 98, respectively. We have specified a condition in the ‘if’ statement (y>x).
The statements in the ‘if’ block will be executed only if the given condition evaluates to true. Otherwise, the final print statement will be printed.
As the value of y is greater than x, i.e., 98 > 34, the statements inside the ‘if’ block will be executed, and the result will be:
x is less than y.
Welcome to TechGeekBuzz!
2. If-else Statement
The Python ‘if-else’ statement implies that if the condition specified in ‘if’ evaluates true, then execute the set of code present in the ‘if’ block. Otherwise, execute the set of code present in the ‘else’ block. The rest of the statements outside the ‘if’ and ‘else’ block will be executed.
Syntax:
if (expression):
statement(s)
else:
statement(s)
Flowchart:
Example:
a = 23
if (a > 20):
print("a is greater than 20.")
else:
print("a is less than 20.")
print("Welcome to TechGeekBuzz!")
Output:
In the above example, we have taken a variable ‘a’ whose value is 23 and specified a condition (a>20) in the ‘if’ statement.
If the condition in ‘if’ evaluates to true, the statement inside the ‘if’ block will be executed; otherwise, the statement in the ‘else’ block will be executed.
The condition in the ‘if’ statement evaluates to true as 23 is greater than 20. Therefore, the statement in the ‘if’ block will be executed, and the result will be:
a is greater than 20.
Welcome to TechGeekBuzz!
3. Else-If (elif) Statement
We generally use the Python ‘elif’ statement when we need to justify more than two results. This statement enables us to check for multiple conditions. It implies that if the condition in the ‘if’ statement evaluates to false, move to the ‘elif’ statement, evaluate its condition and if it results in false, execute the ‘else’ block.
Syntax:
if (expression):
statement(s)
elif (expression):
statement(s)
else:
statements(s)
```
`
####Flowchart:
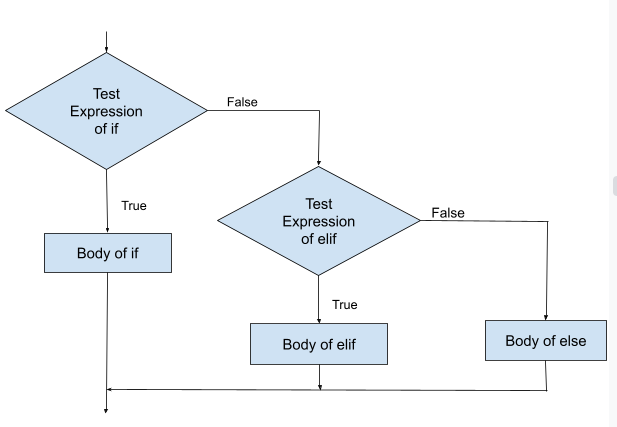
####Example:
`
```
n = 5
if (n<0):
print("n is a negative number")
elif (n==0):
print("n is zero")
else:
print("n is a positive number")
print("Welcome to TeckGeekBuzz!")
```
`
####Output:
In this example, we have assigned a value of 5 to a variable ‘n’, and we have to check whether ‘n’ is a negative number, zero, or a positive number.
If the condition in the ‘if’ statement results in true, the statement inside the ‘if’ block will be executed. Otherwise, the condition in the ‘elif’ statement will be checked. If it evaluates to true, the statement inside the ‘elif’ block will be executed. Otherwise, the statement in the ‘else’ block will be executed.
We have specified the condition (n<0) in the ‘if block’, which evaluates to false as n is not less than 0. Therefore, the control flow moves to the ‘elif’ block, having the condition ‘n==0’. This condition also results in false. Finally, the statement in the ‘else’ block is true and thus the last print statement will be executed. The output will be:
`
```
n is a positive number.
Welcome to TechGeekBuzz!
```
`
###4. Nested if-else Statement
The Python nested if-else statement implies that ‘if’ or ‘else’ statements can have another ‘if’ or ‘if-else’ statement within it. We can check multiple conditions using the nested if-else statement.
####Syntax:
`
```
if (expression):
if (expression):
statement(s)
else:
statement(s)
else:
statement(s)
```
`
####Flowchart:
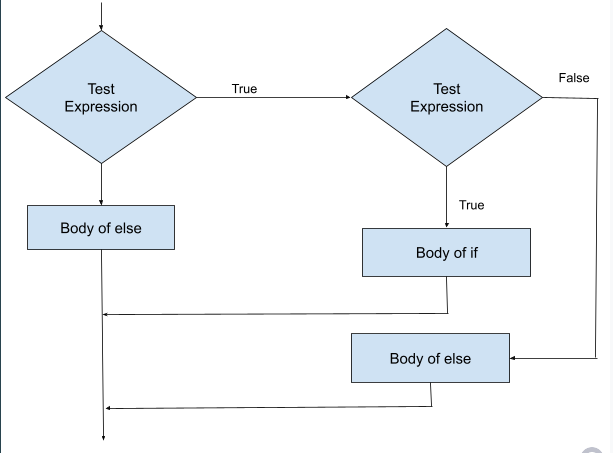
####Example:
`
```
p = 20
if (p>0):
#First if
if (p<30):
print("p is smaller than 30.")
#Nested if
#The below 'if' statement gets executed only
#if the above one holds true
if (p<15):
print("p is smaller than 15 too.")
else:
print("p is greater than 15.")
else:
print("p is a negative number.")
print("Welcome to TechGeekBuzz!")
```
`
####Output:
In this example, we have a variable whose value is 20. The ‘if’ statement has the condition ‘p>0’. If this condition evaluates to true, then the set of code inside the ‘if’ block will be executed. Otherwise, the statement in the ‘else’ block will be executed.
The condition ‘p>0’ holds true, and the set of code inside the ‘if’ block gets executed. This ‘if’ block has ‘nested if’. The control flow moves to the second ‘if’ only in case the condition of the first ‘if’ evaluates to true.
We have the condition ‘p<30’ in the first ‘if’, which holds true, and the statement inside this ‘if’ block gets executed. Now the control flow moves to the second ‘if’ with the condition ‘p<15’, which does not hold true. Finally, the statement in the ‘else’ block gets executed, followed by the last print statement of the program.
The output will be:
`
```
p is smaller than 30.
p is greater than 15.
Welcome to TechGeekBuzz!
```
`
###5. Else-if (elif) Ladder
As its name suggests, an elif ladder is a sequence of ‘elif’ statements in a program. It helps us to check multiple conditions. As soon as the condition in any of the ‘elif’ statements evaluates to true, it executes a set of code present in that ‘elif’ block.
####Syntax:
`
```
if (expression):
statement(s)
elif (expression):
statements(s)
elif (expression):
statement(s)
.
.
.
else:
statement(s)
```
`
####Flowchart:
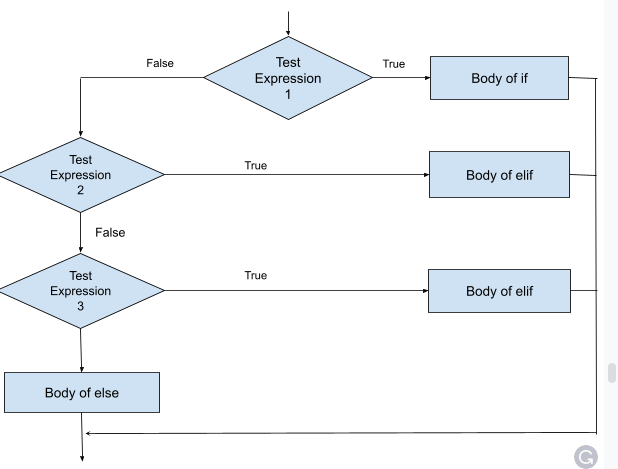
####Example:
`
```
marks = 95
if (marks<35):
print("Fail")
elif (marks>35 and marks<60):
print("Second Class")
elif (marks>60 and marks<80):
print("First Class")
else:
print("Distinction")
```
`
####Output:
Here, we have a variable ‘marks’ with a value of 95. The ‘if’ statement has the condition ‘marks<35’, which does not hold true, therefore, the control flow moves to the first ‘elif’ statement, having the condition ‘marks>35 and marks<60’. As the value of marks is 95, the condition of the first elif statement evaluates to false.
Now, the condition of the second ‘elif’ statement, ‘marks>60 and marks<80’, is checked, which does not hold true. Finally, the statement in the ‘else’ block gets executed, and the result will be:
`
```
Distinction
```
`
###6. Shorthand if and if-else Statements
We can use shorthand if and if-else statements when there is a single line of code to execute in ‘if’ or ‘else’ blocks.
####Syntax of Shorthand if:
`
```
If condition: statement
```
`
####Example of Shorthand if:
`
```
i = 10
If i<20:
print("i is less than 20.")
```
`
####Output:
`
```
i is less than 20.
```
`
####Syntax of Shorthand if-else:
`
```
statement_when_True if condition else statement_when_False
```
`
####Example of Shorthand if-else:
`
```
i = 20
print(True) if i<15 else print(False)
```
`
####Output:
`
```
False
```
`
###Conclusion
This is all about Python conditional statements. We have covered five different types of Python conditional statements. These statements control the execution of a program. The only thing to remember while using Python conditional statements is to use indentation properly.
We use the ‘if’ statement to execute a block of code when the condition evaluates to true. If the condition in ‘if’ fails, we execute the code specified in the ‘else’ block. When there are multiple possibilities as an outcome, or we need to check for multiple conditions, we use the ‘elif’ statement. We can add as many ‘elif’ statements depending upon the number of conditions, and hence it forms an elif ladder.
Top comments (0)