This is a quick step-by-step tutorial designed to guide you through setting up the project. While I strive to provide detailed explanations, feel free to skip ahead and simply follow each step; you should still achieve the desired results.
Set up React Project with Vite
npm create vite <project-title>
- Select
React
->TypeScript
. - Run
npm install
andnpm run dev
to start a local development server.
Setting up Vitest
Vitest test runner is optimised for Vite-powered projects.
npm install -D vitest
npm install -D @testing-library/react @testing-library/jest-dom jsdom
First package, @testing-library/react, is for component testing, second, @testing-library/jest-dom, is for DOM assertions, and lastly, jsdom, is for simulating a browser environment in Node for testing purposes.-
Update vite.config.ts to include testing configurations
///
///
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react';
// https://vitejs.dev/config/
export default defineConfig({
plugins: [react()],
test: {
globals: true,
environment: 'jsdom',
setupFiles: ['./src/setupTests.ts'],
},
});
Changes we made:
- Added type definitions at the beginning of the file
- `globals: true` means global variables will be available during tests like 'describe, it, expect' so we don't have to import it in every test file
- Specified _'jsdom'_ as the test environment, simulating a browser environment
- Defined _'setupFiles'_ to execute necessary code before running the tests. This is a perfect segue to create _setupTests.ts_.
## Create setupTests.ts
1. Create _setupTests.ts_ in the **src** directory
2. Paste this into _setupTests.ts_
import * as matchers from '@testing-library/jest-dom/matchers';
import { expect } from 'vitest';
expect.extend(matchers);
Here, we bring Jest's DOM matchers into Vite, making testing feel more familiar and easier for users familiar with Jest.
3. Add `"test": "vitest"` to _package.json_

This will allow you to run your tests using `npm test`.
Onto, to our first test!
## Writing our first test
1. Let's create a new file called _App.test.tsx_ in the **src** folder.
2. Here's a passing test for you to get started:
import { screen, render } from "@testing-library/react";
import App from "./App";
describe("App tests", () => {
it("should render the title", () => {
render();
expect(
screen.getByRole("heading", {
level: 1,
})
).toHaveTextContent("Vite + React");
});
});
But oh wait! It's all red!
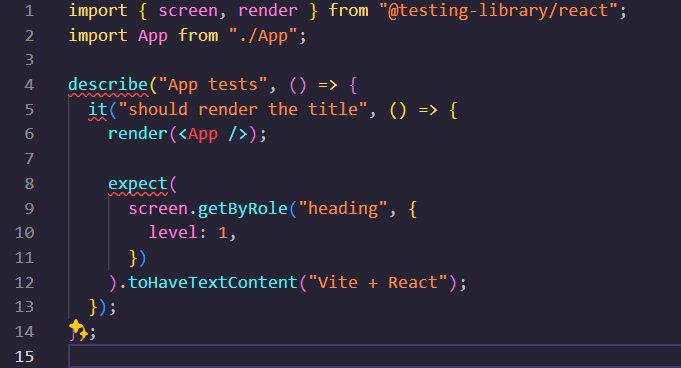
The tests will pass, we just need to tell TypeScript to include type definitions for Vitest global variables and Jest DOM matchers.
3. Add `"types": ["vitest/globals", "@testing-library/jest-dom"],` to _tsconfig.json_ in the `compilerOptions`.
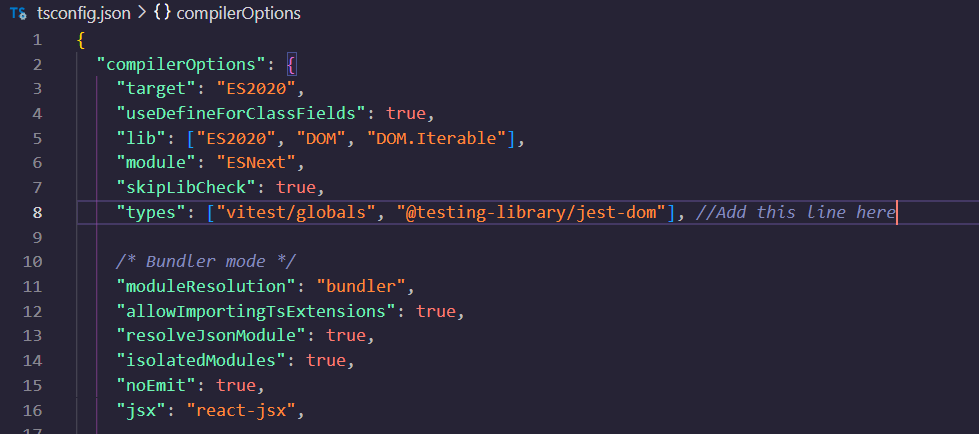
Voilà! If you enjoy this content, please consider supporting my efforts. Your generosity fuels more of what you love! 🩷
<a href="https://www.buymeacoffee.com/janoskocs" target="_blank"><img src="https://cdn.buymeacoffee.com/buttons/default-orange.png" alt="Buy Me A Coffee" height="41" width="174"></a>
----------
I'm János, I write about Software, coding, and technology.
Checkout my [portfolio](https://janoskocs.com). 💾
Top comments (6)
Hello Janos, Thank you posting this.
I am having an issue building for production when i deploy due to the line below under tsconfig.app.json compiler option:
"types": ["vitest/globals", "@testing-library/jest-dom"]
Is there a way I can resolve this, I have tried my best, and searched but still hanging, will really appreciate your assistance, thanks.
Hi Janos, I have tried that but it is still failing, the error is different though.
I have attached an image below.
I will be very glad, if we can get on call to fix this once and for all. Thank you Janos.
Hi AbdulAfeez, let's connect on LinkedIn and we can catch up on a call, I'm not 100% sure what's going on here. Did you try other solutions?
Hi Janos,
I have tried other options, but, I felt they are not a good practice.
Please can I get your linkedin handle, for me to connect with you, and probably schedule a call?
Thank you.
Hey AbdulAfeez, sure thing, you can find it on my GitHub or Portfolio!