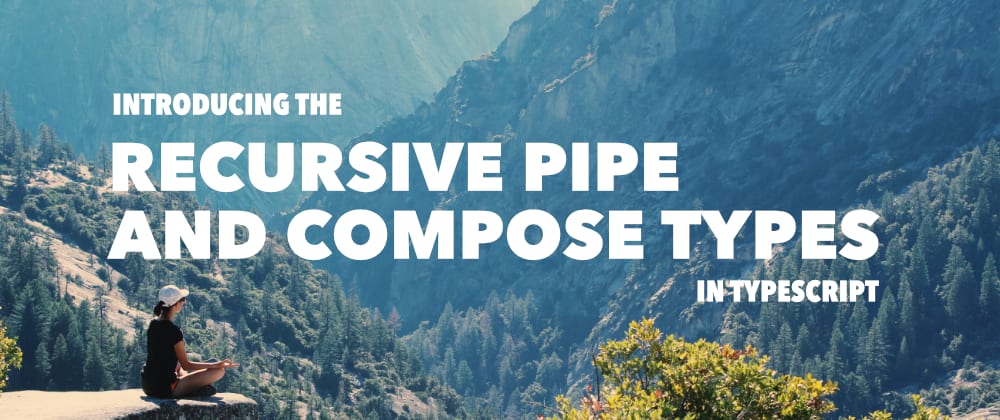
It turns out, the recursive Pipe (and Compose) types offer key advantages over the traditional method of using parameter overloading. The key advan...
For further actions, you may consider blocking this person and/or reporting abuse
Hi, at first I would like to say that I like your approach the most of all I've seen.
My question, coming from React, is:
Would it be possible to compose functions in a way, that any extra incoming arguments are passed along with current's hoc outgoing arguments to next hoc, and that any extra arguments of the wrapped function that are not satisfied by any hoc are also exposed in the outer interface?
In React it makes sense as higher order components/functions usually don't represent a chain of actions on a single value, but instead add some functionality and add additional props to the underlying consumer component. Though, it's all in a single object called props.
It's somewhat difficult to express clearly, so here's the idea:
A real life usage could be
Where the
MyComponent
doesn't really care about whatwithSettings
returns, butwithApolloQuery
needs it.MyComponent
then cares about the result, theme and the consumer should provide the additional required prop:<MyComponent giveMeThisProp={true} />
Great article and wow, what a complex and powerful type! Is there a way to get the actual argument (and not its type) recursively? I'm currently trying to create such a type for Solid.js' setStore function, which has basically the following interface:
A selector can be a
keyof Item
,(keyof Item)[]
, a range{ from: number, to: number }
or a filter function. A setter can either be a DeepPartial value of the selected type, undefined or a function that receives the current Item and returns aforesaid values.¹: each subsequent selector should not receive the Store, but the selection within it.
²: the setter should receive the selection of the last selector.
However, a selector could also be a string or number if the selected item inside the store was an object or array. Unfortunately, if I use
I get the error that my Selectors are matching
undefined
. Any ideas or pointers on what I'm doing wrong?