Grammar is not a joke...
Exactly, in Javascript's grammar there's no else if
statement.
How many times have you used it before? Why It's still working?
We always code like this:
function wow(arg){
if(arg === "dog"){
return "LOVELY";
}
else if(arg === "cat"){
return "CUTE";
}
else return "gimme an animal";
}
wow("cat");
//-> "CUTE"
But what's really happening is this:
function wow(arg){
if(arg === "dog"){
return "LOVELY";
}
else {
if(arg === "cat"){
return "CUTE";
}
else return "gimme an animal";
}
}
wow("cat");
What's happening here?
Literally, we're using some implicit JS behavior about {}
uses.
When we use the else if
statement we're omitting the {}
but Javascript It's still working because It does not requires the parentheses in that case, like in many other cases!
...so what?
I'm not writing this post, just because It's something really curious to know.
I'm writing this to make you think about all the good parts or right ways to code, that forces you to write code in a way, that sometimes It's not really the best way.
There's a lot to discuss about implicit and explicit declaration of stuff like: coercion, parentheses, semicolon...
But the true always stands in the middle!.
If you just follow some specific rules on how to... you're not understanding why those rules were written, and this else if
should make you think about It.
How many time have you written code, because someone told you to do so but you were totally blind about It?
I bet, a lot.
I'm not saying that we should not care about ALL those rules, and that we should know ALL the JS documentation.
I'm just saying that right now, your duty is to write good code that can be understood by someone else and to go that way... some rules are ok, but you should know the why.
Because someone is good at code, It does not mean that you have to follow his golden rules.
What's implicit for him, maybe explicit for you and many other people.
If you don't have the same knowledge, about that specific argument (and It's not possible to have exactly the same level of know about It in every single part of the code) you've two options:
- Do what he's telling you to do... If It works.
- Go out and check the why
Always care about the good parts but first of all, always care about your knowledge and don't code just by rules.
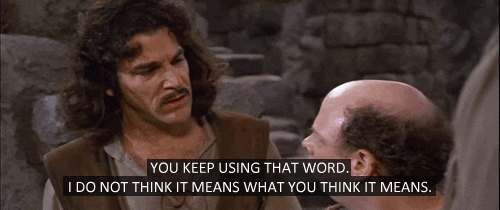
Latest comments (98)
I'm not a compiler guru or have scoured the V8 code, but for languages that support this, the relevant BNF typically goes something like:
if-stmt ::= if <condition> <stmt> [else <stmt>] [;]
stmt ::= <expr> || <expr-block>
I.e. it's not an implicit set of brackets, it's one statement or a statement block.
If you're wondering why we do these things as rote, it's largely fashion. This is the fashionable way to write code at the moment. Many codebases are time capsules for the standards of the time, which is why everyone wants to rewrite them every 5 years! In only the last month or so, suddenly
arg => arg
is out of fashion (now has to be(arg) => arg
)! In fact, seniors would most likely say your example should be aswitch
, but that's literally a dirty word these days (I had go way down the comments to find someone suggest it!), even though it's meant for exactly that situation. The fact you did this example in a small function and did returns is a relatively new phenomena.The reasons for being overly syntactical were quite sane at the time. For example, the reason why we used to explicitly put brackets round every block in an if statement was because:
I don't think it's necessary for everyone to know these sorts of reasons why they're spared the extra syntax. But without going through the pain, we wouldn't know what were the good bits. But.. just maybe... you might have some more sympathy for the senior devs out there! :D
The brackets, if you put them in, do a great job of showing why the conditions up to a success are evaluated, but the ones after aren't.
No, I don't mean leaving brackets in your code is okay, I meant it as an exercise.
Please re-read: w3schools.com/js/js_if_else.asp
I don't understand how this could be true :/
"dont do this.. dont do that.."
THE"if" you gonna need it.. wether your boss,your mentor, your mom tell you not to do so.
I don't think one can design a computer without any logic gate. And, transformation doesn't change how a thing is designed.
I'm intrigued by what you said about
if-statements
. I'm curious to know how you would write the following callback function.I'm still a novice so it's difficult for me to imagine doing some things without
if-statements
. I would appreciate it if you could show me😅🙂.You could use something like promises.
Ooh. That's right. Forgot promises could do that😅. Thanks 😊
function wow(arg)
{
if (arg === "dog") return "LOVELY";
if (arg === "cat") return "CUTE";
return ("gimme an animal");
}
wow("cat");
//-> "CUTE"
// or
function anotherWow()
{
return (arg === “dog”) ? “LOVELY”:((arg === “cat”) ? “CUTE”:”gimme an animal”);
}
anotherWow(“cat”);
Yah... but what? 😅
Here are two real-world pieces of code I wrote yesterday. I describe the problem I'm solving, link to the relevant resources that led me to write that code, and include the
if
statement laden code I wrote. Your absolutism and your certainty has me rolling my eyes, so go ahead and prove me wrong! Rewrite my code without if statements in a way that's better: gist.github.com/JoshCheek/71623a73...I mean,
else if
is syntactic sugar forelse { if ... }
as opposed to being a special language construct (like Python'selif
), but it does exactly the same thing. You could write basically the same article about how C pointers are just integers, or about how aswitch
statement is just syntactic sugar for something nasty-looking withgoto
, or any number of things. A more honest post might talk about how a CPU can't execute JavaScript, so it gets translated into machine code -else if
andelse { if
look the same at that level, since they mean the same thing. Which one is syntactic sugar for the other doesn't really matter, and, as others have mentioned in this thread, I'd argue that actually theelse { if
version adds an additional language construct (which is whyif (true) alert('alert!')
works) and is therefore the more honest version.99% of businesses ????????
If you consider that COBOL has been back ...
If you consider that many banks prefer continue with old COBOL (not the new one) for the ATM services.
I you consider that big manifactures build products IoT with a poorly code and very buggy.
I think that you're developing for excelence, despite I've seen in your code of elixir-iteraptor some IF statement
And the folk thinks that PHP syntax is rubbish? When in JS you can use ; or not at all? Or many coders sometimes use var or not at all. Ignoring the importance of var ... If you speak of JS you cannot say "Grammar is not a joke"
Nope... I don't agree.
If you know grammar, you know where to use ";" and where to use "var".
If you dunno It, you just code by memory.
"there is a golden rule"... Sometimes I sacrifice efficiency for readability, sometimes I do the opposite. To say one size fits all is arrogant, narrow minded and not teaching good practices. There should be a good reason to do everything we do, even if it is not the most appropriate it can still be the correct choice for that instance
Unbelievable...golden rule? Really?
You also realize it when you want to use the shorthand.
On the shorthand syntax there is no "else if". You have to write this instead:
(arg === "dog" ? "LOVELY" : (arg === "cat" ? "CUTE" : "gimme an animal"))