In this post, you will learn how to implement anonymous login in Ionic 4 apps using Firebase. We will also learn how to link an existing anonymous login with a new set of email password, so an anonymous user can convert into a normal user. We will implement these in a simple Ionic 4 app and test.
Complete source code of this tutorial is available in the Ionic-4-firebase-anonymous-login
What is Firebase?
If you don’t know much about Firebase … you need to catch up with latest tech news. Firebase is the popular stuff in the market today. One can use Firebase to create quick mobile app back-ends, with a ton of built-in and ready-to-integrate features.
The most used feature of Firebase is as a back-end. But along with back-end, Authentication features of Firebase are a must to use. So, essentially Auth becomes the most used feature by default.
Firebase Authentication Methods
Firebase provides a number of login methods — Email/password, social logins and anonymous login.
Yes! anonymous login. Sounds strange right?
Anonymous login is a special type of login Firebase provides, where the user does not provide any login info from their end. Firebase creates a random ID and logs user in the app. If the user wants to convert to a regular user at a later point, Firebase provides a way to link the anonymous account to a normal credential as well.
That being said, I’m sure you are very much interested in learning all kinds of login with Firebase. You can check out our Firebase social logins with Facebook and Twitter blogs on our site, along with free starters ( 👻 woohoo ! )
What is Ionic 4?
You probably already know about Ionic, but I’m putting it here just for the sake of beginners. Ionic is a complete open-source SDK for hybrid mobile app development. Ionic provides tools and services for developing hybrid mobile apps using Web technologies like CSS, HTML5, and Sass. Apps can be built with these Web technologies and then distributed through native app stores to be installed on devices.
In other words — If you create native apps in Android, you code in Java. If you create native apps in iOS, you code in Obj-C or Swift. Both of these are powerful, but complex languages. With Cordova (and Ionic) you can write a single piece of code for your app that can run on both iOS and Android (and windows!), that too with the simplicity of HTML, CSS, and JS.
Post structure
We will go in a step-by-step manner to explore the anonymous login feature of Firebase. This is my break-down of the blog
STEPS
- Create a Firebase project
- Create a simple Ionic 4 app
- Connect the Ionic app to Firebase
- Implement anonymous login using AngularFire2
- Convert anonymous user to a regular user
We have two major objectives
- Anonymously login a user
- Link an anonymous user to an email/password account, so user can login with those credentials
Since this is an example in Ionic 4, you can avoid Step 6, and complete your testing on the browser itself. Let’s dive right in!

Step1 — Create a Firebase project
If you have ever used Firebase, you can skip to next step. For beginners, you can create a Firebase project by going to Firebase console (sign-in if it asks you to). Create a new project or select an existing one. Your Firebase console dashboard should look like this

Note — It’s really easy to create a Firebase project, but if you still face any issue, follow step 1–4 of this blog
Enable Anonymous login
Once your project is created, go inside the project. Go to Authentication tab, and you need to toggle Anonymous login Enabled

Step 2 — Create a simple Ionic 4 app
I have covered this topic in detail in this blog.
In short, the steps you need to take here are
- Make sure you have node installed in the system (V10.0.0 at the time of this blog post)
- Install ionic cli using npm
- Create an Ionic app using
ionic start
You can create a blank
starter for the sake of this tutorial. On running ionic start blank
, node modules will be installed. Once the installation is done, run your app on browser using
$ ionic serve
With slight modifications, my app’s login page looks like this (fancy icon 🔥, huh)
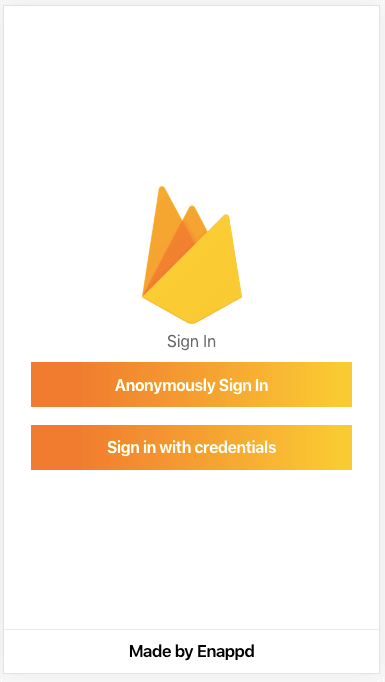
Simple and sweet, just two buttons
This is what we’ll do
- User logs in anonymously → go to Home Page
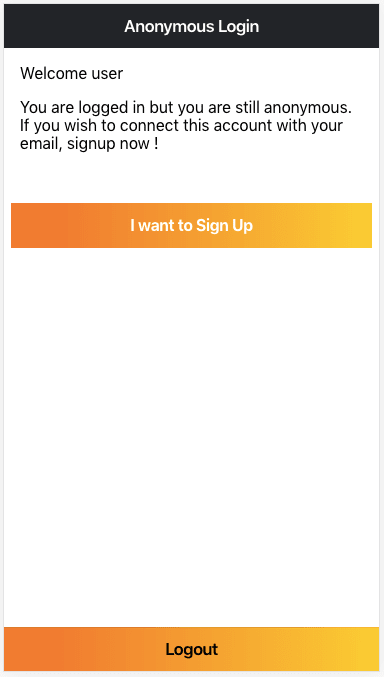
- User clicks “I want to Sign Up” to link his/her credentials with the anonymous account. Email and password fields are provided to the user. User signs up.
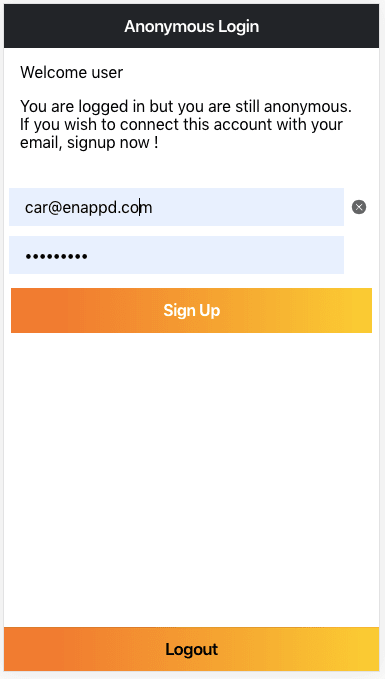
- User then logs out, and logs back in with the credentials he/she connected to the anonymous account
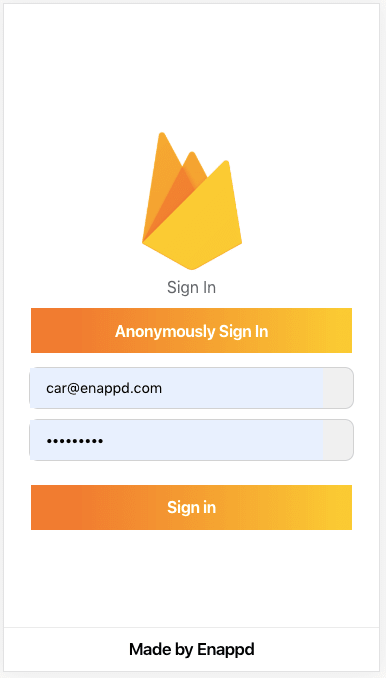
We’ll see the code for this in subsequent steps
Step 3 — Connect the Ionic app to Firebase
Copy Firebase config
Details of this step can be found in our blog How to integrate Firebase in Ionic 4 apps
First, copy your Firebase config information from your Firebase console and paste it in environment
files in your Ionic 4 app. The environment file will look like this
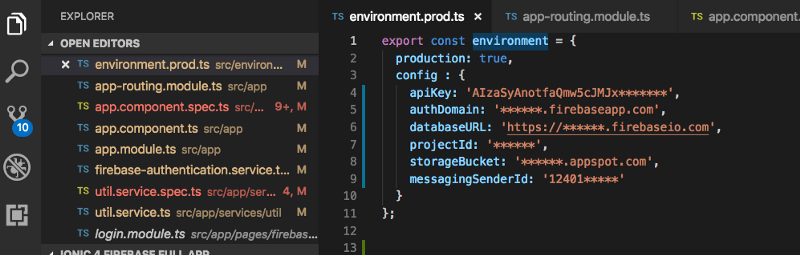
To connect the app to Firebase, and use the Firebase methods, we’ll use AngularFire2 package. Install the package using
$ npm install firebase @angular/fire --save
Import angularFire2 and your environment
in app.module.ts
With this, your app is now connected to Firebase project. Next, we’ll write the functions for anonymous login, logout, linking credentials and signing in with normal credentials.
Step 4 — Implement anonymous login
In login.page.ts
we’ll import angularFire2, and write a method to login anonymously. Our file will look like following
When user clicks “Anonymously Login”, login function is called. It uses auth.signInAnonymously()
method of AngularFire2 auth module. This signs in the user anonymously.
Your user is now logged in, but still anonymous. User can browse features of your app and take a call on becoming a regular user. First objective is achieved 😎 So easy, right?
Step 4 — Convert anonymous user to a regular user
In Homepage, we will provide the user an option to convert to a regular user. User can enter email/password details and signup as a regular user. This will connect all the data of the anonymous profile with the new credentials of the user. So you don’t lose the data and easily convert the user to a regular user.
Following is the function for converting anonymous user to regular user
The email and password above are entered by the user. If the response goes to success, your user is successfully linked to the new credentials! Let’s test that by logging out.
Logout
Test normal login
Go to login page, and allow the user to enter email and password for login. The following function can be used to login a user with already existing credentials
You’ll see that the user is logged in with the linked credentials. Although we don’t have any data associated with the anonymous user to show the linking, but if your app does, the user signed in with Email/password will stay connected to that data as well.
Overall, the process will look something like this

Conclusion
In this blog, we learned how to implement anonymous login in Ionic 4 using Firebase. We also learned how we can connect an anonymous user to a new user with Email/password account. This way you can allow a user to anonymously login to the app, browse the features, and then signup if he/she likes, without losing the anonymous account data!
Complete source code of this tutorial is available in the Ionic-4-firebase-anonymous-login
Next Steps
Now that you have learned the implementation of Firebase anonymous login in Ionic 4, you can also try
- Ionic 4 PayPal payment integration — for Apps and PWA
- Ionic 4 Stripe payment integration — for Apps and PWA
- Ionic 4 Apple Pay integration
- Twitter login in Ionic 4 with Firebase
- Facebook login in Ionic 4 with Firebase
- Geolocation in Ionic 4
- QR Code and scanners in Ionic 4 and
- Translations in Ionic 4
If you need a base to start your next Ionic 4 app, you can make your next awesome app using Ionic 4 Full App

This blog was originally published on enappd.com
Top comments (0)