In this post, you’ll learn how to implement Google login in your Capacitor apps, based on Ionic React framework. We will also retrieve user name and profile photo to show after login. We will test the authentication on Web, Android and iOS.
Since this post is a mix of three new frameworks, it is possible you landed here by mistake. If you are looking for Google login in
- Ionic Angular (Cordova) apps — Please check this post
- React Native — Please check this for Android and iOS
- Ionic React Capacitor Apps — Continue reading 😄
As you can see from above, there are several options available for Hybrid app development these days, and it is easy to get confused between them. This post is focussed on Ionic framework with React as the front-end framework, and Capacitor as runtime and build environment.
Code for this tutorial is available on Github repo ionic-react-capacitor-google-login
Let’s see a brief intro to each of the included frameworks:
- Ionic
- Capacitor
- Ionic-React
What is Ionic ?
In short — If you create Native apps in Android, you code in Java. If you create Native apps in iOS, you code in Obj-C or Swift. Both of these are powerful but complex languages. With Ionic and Cordova/Capacitor you can write a single piece of code for your app that can run on both iOS and Android (and windows!), that too with the simplicity of HTML, CSS, and JS.
It is important to note the contribution of Cordova/Capacitor in this. Ionic is only a UI wrapper made up of HTML, CSS and JS. So, by default, Ionic cannot run as an app in an iOS or Android device. Cordova/Capacitor is the build environment that containerizes (sort of) this Ionic web app and converts it into a device installable app, along with providing this app access to native APIs like Camera etc.
Capacitor — How is it different from Cordova ?
Capacitor is very similar to Cordova, but with some key differences in the app workflow
Cordova helps build Ionic web app into a device installable app. But there are some limitations of Cordova, which Capacitor tries to overcome with a new App workflow.
Capacitor is a cross-platform app runtime that makes it easy to build web apps that run natively on iOS, Android, Electron, and the web. Ionic people call these apps “Native Progressive Web Apps” and they represent the next evolution beyond Hybrid apps.
Here are the differences between Cordova and Capacitor
- Capacitor considers each platform project a source asset instead of a build time asset. That means, Capacitor wants you to keep the platform source code in the repository, unlike Cordova which always assumes that you will generate the platform code on build time
- Capacitor does not “run on device” or emulate through the command line. Instead, such operations occur through the platform-specific IDE. So you cannot run an Ionic-capacitor app using a command like
ionic run ios
. You will have to run iOS apps using Xcode, and Android apps using Android studio - Because of the above, Capacitor does not use
config.xml
or a similar custom configuration for platform settings. Instead, configuration changes are made by editingAndroidManifest.xml
for Android andInfo.plist
for Xcode - Since platform code is a source asset, you can directly change the native code using Xcode or Android Studio. This give more flexibility to developers. We will do some similar changes for Google login as well.
Plugins
Cordova and Ionic Native plugins can be used in Capacitor environment. However, there are certain Cordova plugins which are known to be incompatible with Capacitor. For Google login functionality, we’ll use the Capacitor Google Auth plugin
Other than that, Capacitor also doesn’t support plugin installation with variables. Those changes have to be done manually in the native code. We will do something similar in this post.
Why Ionic React ?
Since Ionic 4, Ionic has become framework agnostic. Now you can create Ionic apps in Angular, React, Vue or even in plain JS. This gives Ionic great flexibility to be used by all kinds of developers.
Ionic Angular apps are supported by both Cordova and Capacitor build environments.
Same is not true for Ionic React apps — Ionic React apps are only supported by Capacitor build environment. Hence, if you want to build apps in Ionic React, you need to use Capacitor to build the app on device.
I know if can get confusing as three frameworks are crossing paths here. Bottom line for this post — Ionic + React + Capacitor + Capacitor Google Login plugin
A word on Google authentication
We will use the Capacitor Google Auth Plugin to authenticate the user. Once the login is done, we receive user profile information in auth response itself. Hence, there is no need to fetch user’s profile information separately.
This Google Auth plugin supports 2 functions, across web, Android and iOS
- Login
- Logout
Structure of post
I always go step-by-step for readers of all experience level. If you know certain steps, feel free to skip them
Step 1: Create a basic Ionic React app
Step 2: Connect Capacitor with your app
Step 3: Create a Google project for authentication
Step 4: Setup Google Login Plugin and functions
Step 5: Prepare and Test on Web
Step 6: Build and Test your app on Android
Step 7: Build and Test your app on iOS
Let’s get started with Ionic React Capacitor Google login !
Step 1 — Create a basic Ionic-React app
First you need to make sure you have the latest Ionic CLI. This will ensure you are using everything latest. Ensure latest Ionic CLI installation using
$ npm install -g ionic@latest
Creating a basic Ionic-React app is not much different or difficult from creating a basic Ionic-Angular app. Start a basic blank
starter using
$ ionic start IonCapReactGoogleLogin blank --type=react
The --type=react
told the CLI to create a React app, not an Angular app !!
Run the app in browser using
$ ionic serve
You won’t see much in the homepage created in the blank starter. Let’s modify this page to include a button, icon and a title for login. Also, I have created a Homepage, where the user is redirected after successful login. The user profile information in this page comes after login and single API call.
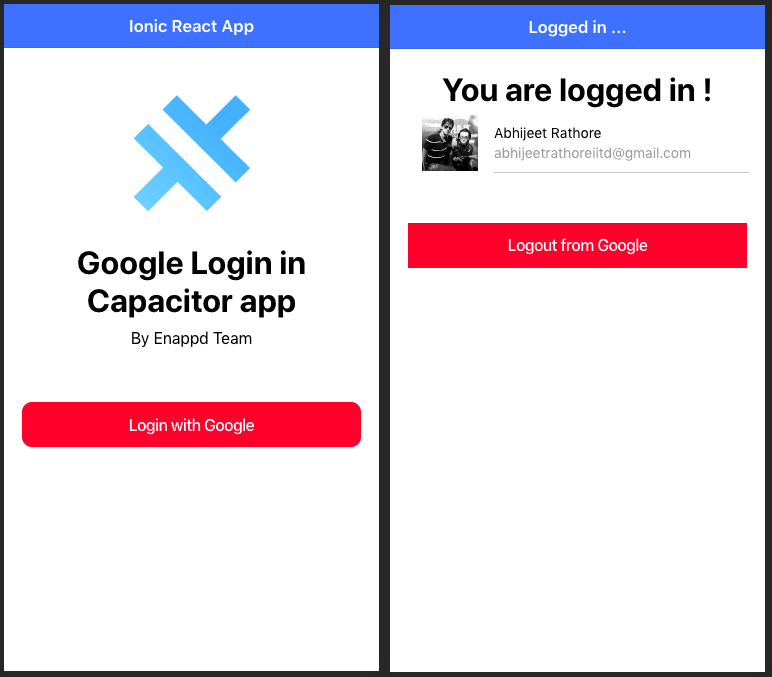
The code for this can be found in the attached Github repository.
Step 2 — Attach Capacitor to your Ionic-React app
Capacitor can be attached to an existing Ionic app as well. To attach Capacitor to your existing Ionic app, run
$ ionic integrations enable capacitor
This will attach Capacitor to your Ionic app. After this, you have to init
the Capacitor app with
$ npx cap init
It will ask you the app name and ID. Give app name whatever you want. App ID is the domain identifier of your app (ex: com.example.app
). Note this ID as this will be required later when you create app in Google developer console. In my case, the ID is com.enappd.IonCapReactGoogleLogin
( too long, I know)
Step 3 — Create a Google app in Developer console
To implement a Google login, you will need a Google app/project in Google developer account.
Create new project
Create a project in Google Developer console or create it in Firebase Console (recommended). Both consoles are essentially integrated with each other.
More details about creating a Firebase project can be found here.

Get project keys and IDs
Now, to implement Google Login in Web, Android and iOS, we need two things
- A web-client ID
-
google-services.plist
file from the project containing project configuration
google-services.plist
In your Firebase console, create a new iOS app. During creation process it will ask you the app bundle ID. Use the bundle ID you used to initialize Capacitor in section 2 (com.enappd.IonCapReactGoogleLogin
). In next step, download the google-services.plist
file.
If you already have an existing project, download the google-services.plist
from the Project Settings page.

google-services.plist from your Firebase iOS project
web-client ID
I will create the Android part of ID in Google developer console just to show that it can be done in either places — Firebase or Google Dev Console.
Open you Google Developer console. Select the project you are working on, and go to Credentials page from the left side menu. In this page, you’ll see several keys and IDs created for your project.
If you have haven’t added an Android app in this Project (in Firebase) you will not see an Android client-ID here. Create a new credential for Android using Create Credential → OAuth client ID option, and select Android

Fill the required options, and make sure you enter the correct package name

Now, this Android Client-ID is created BUT you will not need this. This is only required to be generated to authenticate an Android app in the project. You will only need the web-client ID . Note it down as well.
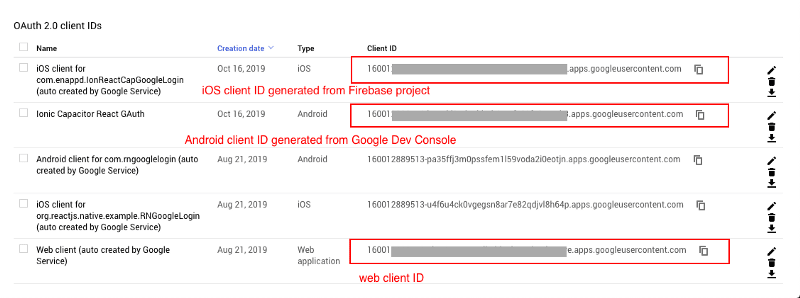
If you create Android app in Firebase, you can get web-client ID by downloading google-services.json
file
Step 4 — Setup Google Login Plugin and functions
To setup Google login in the app, we’ll do following things
4.1 Install Capacitor Google Auth plugin
4.2 Setup plugin functions for login
4.3 Enable routing between two pages of the app
4.4 Control navigation via login / logout from Google Auth
4.5 User’s profile information
4.1 Install Capacitor Google Auth plugin
Install the plugin using
$ npm install --save @codetrix-studio/capacitor-google-auth
Add the following info in your capacitor.config.json
...
"plugins": {
"GoogleAuth": {
"scopes": ["profile","email"],
"serverClientId": "160012889513-qkxxxxxxxxxxxxxxxxxxxxxxxxkgubrp5ve.apps.googleusercontent.com"
}
}
...
serverClientId
is basically the web-client ID itself. Import the plugin in app’s pages using
import "@codetrix-studio/capacitor-google-auth";
import { Plugins } from '@capacitor/core';
After this, build your app using following commands
// Build web assets
$ ionic build
// Run the app in browser
$ ionic serve
4.2 Setup plugin functions for login
We have to setup only two major functions for Google Auth functionality
- Login — Plugins.GoogleAuth.signIn()
- Logout — Plugins.GoogleAuth.signOut()
Login Page
The complete code for Login Page looks like this
Some basic things
history.push({
pathname: '/home',
state: { name: result.name || result.displayName, image: result.imageUrl, email: result.email
}
});
Here, state
field contains navigation params (props). These will be accessed in next page from the location
object of props
. The auth response returns name
field in web and iOS, while it return displayName
in Android.
Home Page
The complete code for Home Page looks like this
The name
, image
and email
sent from first page is accessed via this.props.location.state.name
and so on. This will be used further to display user’s profile information. The Auth response is given in Section 4.5
4.3 Enable routing between two pages of the app
The routes are defined in App.tsx
file like this
<IonApp>
<IonReactRouter>
<IonRouterOutlet>
<Route path="/login" component={Login} exact={true} />
<Route path="/home" component={Home} exact={true} />
<Route exact path="/" render={() => <Redirect to="/login" />} />
</IonRouterOutlet>
</IonReactRouter>
</IonApp>
4.4 Control navigation via login / logout from Google
Once user is successfully logged in, we want to redirect to Home
page. And when user logs out, we want to come back to Login
page.
On Login
page, history.push
redirects user to next page after successful login
On Login
page,history.goBack()
takes you back to previous page after logout is successful.
4.5 User’s profile information
User’s profile info is returned in auth response in this format
{"authentication": {
"accessToken": "xxxxxxxxxx",
"idToken": "xxxxxxxxxxxx"},
"email": "abhijeetrathoreiitd@gmail.com",
"familyName": "Rathore",
"givenName": "Abhijeet",
"id": "104xxxxxxxxx2852",
"imageUrl": "https://lh3.googleusercontent.com/a-/AAuE7XXXXXXXXXXXXEq-pnteIcLe-XGib4kn7eZsQ=s96-c",
"name": "Abhijeet Rathore",
"serverAuthCode": "XXXXXXXX"
}
Step 5: Prepare and Test on Web
To implement Google Auth in web app with Capacitor, add clientId
meta tag to head in public/index.html
file.
<meta name="google-signin-client_id" content="{your client id here}">
With all the above code in place, web Google login can be tested in ionic serve
itself. Here’s how it will work on browser
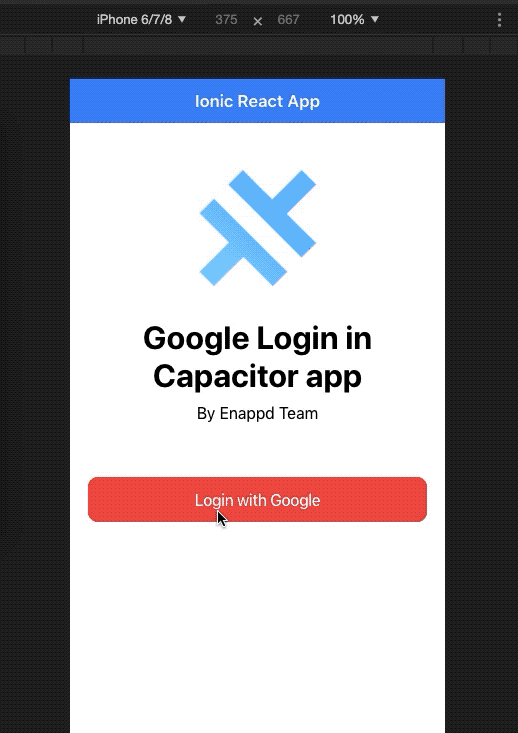
Note: At the time of writing this blog, V1.2.1 of Google Auth plugin, signOut function is missing for Web. If you want to fix this, go to node_modules/@codetrix-studio/capacitor-google-auth/dist/esm/definitions.d.ts
and add a signOut declaration as
signOut(): Promise<{value: string;}>;
Step 6 — Build and Test your app on Android
To build the app on Android, run these commands
// Add android platform
$ npx cap add android
// Copy all changes to Android platform
$ npx cap sync
// Open the project in Android studio
$ npx cap open android
In Android studio, locate file android/app/src/main/java///MainActivity.java
, and add the plugin to the initialization list:
import com.codetrixstudio.capacitor.GoogleAuth.GoogleAuth
this.init(savedInstanceState, new ArrayList<Class<? extends Plugin>>(){{<br> ...<br> add(GoogleAuth.class);<br> ...<br>}}
);

Also, add the following in your strings.xml
file in Android Project
<resources>
<string name="server_client_id">Your Web Client ID</string>
</resources>
Build the app on Android device using Android studio. You should be able to login via Google, and reach the inner page where you see the user information. Following GIF shows the login flow in my OnePlus device.

Step 7— Build and Test your app on iOS
To build the app on iOS, run these commands
// Add android platform
$ npx cap add ios
// Copy all changes to iOS platform
$ npx cap sync
// Open the project in XCode
$ npx cap open ios
- Place your downloaded
GoogleService.plist
inios/App
folder - Find
REVERSED_CLIENT_ID
from theGoogleService.plist
and Add it as a URL scheme ininfo.plist
of Xcode
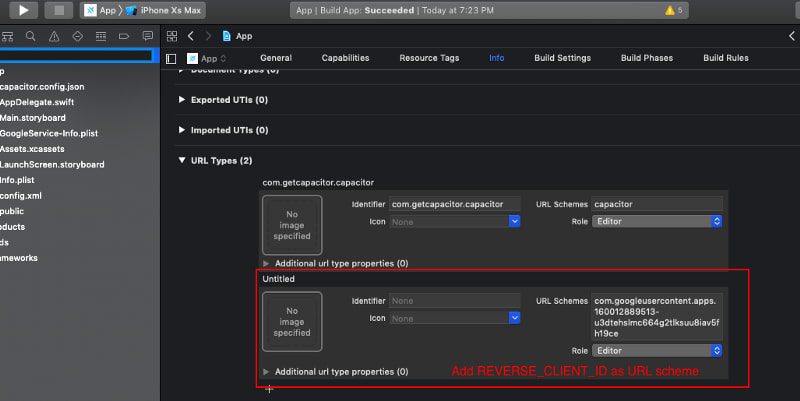
Build the app on iOS device or Simulator using Xcode. You should be able to login via Google, and reach the inner page where you see the user information. Following GIF shows the login flow in my OnePlus device.
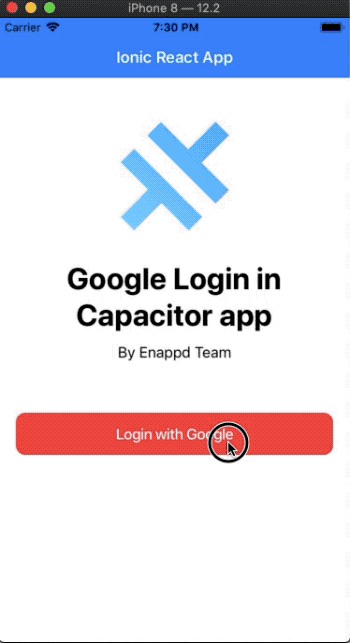
Conclusion
In this post you learnt how to implement Google login in your Ionic React Capacitor app. Social logins are very important part of your apps, as they make users trust your apps more. It is also easy to use, and users don’t have to remember any passwords. You can always link the Social logins with your server as well.
We also tested the Authentication on all three devices — Android, iOS and Web. This creates a complete set of devices a user can use with your app.
Code for this tutorial is available on Github repo ionic-react-capacitor-google-login
Next Steps
Now that you have learned the implementation of Google Login in Ionic React Capacitor app, you can also try following blogs for other Ionic apps
Ionic React Capacitor
- Facebook Login in Ionic React Capacitor Apps
- Twitter Login in Ionic React Capacitor Apps
- How to make basic apps in ionic-react-capacitor
- Camera and Image gallery in Ionic-React-Capacitor
- Push notification in Ionic-React-Capacitor apps
- Playing Music in Ionic Capacitor apps
- Adding Icon and Splash in Ionic React Capacitor apps
- Create HTML5 games in Ionic Capacitor apps using Phaser
If you need a base to start your next Ionic 4 React app, you can make your next awesome app using Ionic React Full App

Ionic Angular
- Ionic 4 Payment Gateways — Stripe | PayPal | Apple Pay | RazorPay
- Ionic 4 Charts with — Google Charts | HighCharts | d3.js | Chart.js
- Ionic 4 Social Logins — Facebook | Google | Twitter
- Ionic 4 Authentications — Via Email | Anonymous
- Ionic 4 Features — Geolocation | QR Code reader | Pedometer
- Media in Ionic 4 — Audio | Video | Image Picker | Image Cropper
- Ionic 4 Essentials — Native Storage | Translations | RTL
- Ionic 4 messaging — Firebase Push | Reading SMS
- Ionic 4 with Firebase — Basics | Hosting and DB | Cloud functions
If you need a base to start your next Ionic 4 Angular app, you can make your next awesome app using Ionic 4 Full App

Top comments (0)