Building an NFT marketplace with Polygon, Next.js, Tailwind, Solidity, Hardhat, Ethers.js, and IPFS
To view the video course for this tutorial, click here. To view Version 1 of this tutorial, click here
In my last end to end Ethereum tutorial, The Complete Guide to Full Stack Ethereum Development I introduced how to build a basic app on Ethereum using modern tooling like Hardhat and Ethers.js.
In this guide you'll learn how to build, deploy, and test out a full stack NFT marketplace on Ethereum. We'll also look at how to deploy to Polygon.
One thing that has become apparent is how quickly Ethereum scaling solutions like Polygon, Arbitrum, and Optimism are gaining momentum and adoption. These technologies enable developers to build the same applications they would directly on Ethereum with the added benefits of lower gas costs and faster transaction speeds among other things.
Because of the value proposition that these solutions offer combined with the general lack of existing content, I will be building out various example projects and tutorials for full stack applications using these various Ethereum scaling solutions, starting with this one on Polygon.
To view the final source code for this project, visit this repo
Prerequisites
To be successful in this guide, you must have the following:
- Node.js version
16.14.0
or greater installed on your machine. I recommend installing Node using either nvm or fnm. - Metamask wallet extension installed as a browser extension
The stack
In this guide, we will build out a full stack application using:
Web application framework - Next.js
Solidity development environment - Hardhat
File Storage - IPFS
Ethereum Web Client Library - Ethers.js
Though it will not be part of this guide (coming in a separate post), we will look at how to build a more robust API layer using The Graph Protocol to get around limitations in the data access patterns provided by the native blockchain layer.
About the project
The project that we will be building will be Metaverse Marketplace - an NFT marketplace.
When a user puts an NFT for sale, the ownership of the item will be transferred from the creator to the marketplace contract.
When a user purchases an NFT, the purchase price will be transferred from the buyer to the seller and the item will be transferred from the marketplace to the buyer.
The marketplace owner will be able to set a listing fee. This fee will be taken from the seller and transferred to the contract owner upon completion of any sale, enabling the owner of the marketplace to earn recurring revenue from any sale transacted in the marketplace.
The marketplace logic will consist of just one smart contract:
NFT Marketplace Contract - this contract allows users to mint NFTs and list them in a marketplace.
I believe this is a good project because the tools, techniques, and ideas we will be working with lay the foundation for many other types of applications on this stack – dealing with things like payments, commissions, and transfers of ownership on the contract level as well as how a client-side application would use this smart contract to build a performant and nice-looking user interface.
In addition to the smart contract, I'll also show you how to build a subgraph to make the querying of data from the smart contract more flexible and efficient. As you will see, creating views on data sets and enabling various and performant data access patterns is hard to do directly from a smart contract. The Graph makes this much easier.
About Polygon
From the docs:
"Polygon is a protocol and a framework for building and connecting Ethereum-compatible blockchain networks. Aggregating scalable solutions on Ethereum supporting a multi-chain Ethereum ecosystem."
Polygon is about 10x faster than Ethereum & yet transactions are more than 10x cheaper.
Ok cool, but what does all that mean?
To me it means that I can use the same knowledge, tools, and technologies I have been using to build apps on Ethereum to build apps that are faster and cheaper for users, providing not only a better user experience but also opening the door for many types of applications that just would not be feasible to be built directly on Ethereum.
As mentioned before, there are many other Ethereum scaling solutions such as Arbitrumand Optimism that are also in a similar space. Most of these scaling solutions have technical differences and fall into various categories like sidechains , layer 2s, and state channels.
Polygon recently rebranded from Matic so you will also see the word Matic used interchangeably when referring to various parts of their ecosystem because the name still is being used in various places, like their token and network names.
To learn more about Polygon, check out this post as well as their documentation here.
Now that we have an overview of the project and related technologies, let's start building!
Project setup
To get started, we'll create a new Next.js app. To do so, open your terminal. Create or change into a new empty directory and run the following command:
npx create-next-app nft-marketplace
Next, change into the new directory and install the dependencies using a package manager like npm
, yarn
, or pnpm
:
cd nft-marketplace
npm install ethers hardhat @nomiclabs/hardhat-waffle \
ethereum-waffle chai @nomiclabs/hardhat-ethers \
web3modal @openzeppelin/contracts ipfs-http-client \
axios
Setting up Tailwind CSS
We'll be using Tailwind CSS for styling, we we will set that up in this step.
Tailwind is a utility-first CSS framework that makes it easy to add styling and create good looking websites without a lot of work.
Next, install the Tailwind dependencies:
npm install -D tailwindcss@latest postcss@latest autoprefixer@latest
Next, we will create the configuration files needed for Tailwind to work with Next.js (tailwind.config.js
and postcss.config.js
) by running the following command:
npx tailwindcss init -p
Next, configure your template content
paths in tailwind.config.js:
/* tailwind.config.js */
module.exports = {
content: [
"./pages/**/*.{js,ts,jsx,tsx}",
"./components/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Finally, delete the code in styles/globals.css and update it with the following:
@tailwind base;
@tailwind components;
@tailwind utilities;
Configuring Hardhat
Next, initialize a new Hardhat development environment from the root of your project:
npx hardhat
? What do you want to do? Create a basic sample project
? Hardhat project root: <Choose default path>
If you get an error referencing your README.md, delete README.md and run
npx hardhat
again.
Now you should see the following files and folders created for you in your root directory:
hardhat.config.js - The entirety of your Hardhat setup (i.e. your config, plugins, and custom tasks) is contained in this file.
scripts - A folder containing a script named sample-script.js that will deploy your smart contract when executed
test - A folder containing an example testing script
contracts - A folder holding an example Solidity smart contract
Next, update the configuration at hardhat.config.js with the following:
View the gist here
/* hardhat.config.js */
require("@nomiclabs/hardhat-waffle")
module.exports = {
defaultNetwork: "hardhat",
networks: {
hardhat: {
chainId: 1337
},
// unused configuration commented out for now
// mumbai: {
// url: "https://rpc-mumbai.maticvigil.com",
// accounts: [process.env.privateKey]
// }
},
solidity: {
version: "0.8.4",
settings: {
optimizer: {
enabled: true,
runs: 200
}
}
}
}
In this configuration, we've configured the local Hardhat development environment as well as the Mumbai testnet (commented out for now).
You can read more about both Matic networks here.
Smart Contract
Next, we'll create our smart contract!
In this file I'll do my best to comment within the code everything that is going on.
Create a new file in the contracts directory named NFTMarketplace.sol. Here, add the following code:
View the gist here
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.4;
import "@openzeppelin/contracts/utils/Counters.sol";
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol";
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "hardhat/console.sol";
contract NFTMarketplace is ERC721URIStorage {
using Counters for Counters.Counter;
Counters.Counter private _tokenIds;
Counters.Counter private _itemsSold;
uint256 listingPrice = 0.025 ether;
address payable owner;
mapping(uint256 => MarketItem) private idToMarketItem;
struct MarketItem {
uint256 tokenId;
address payable seller;
address payable owner;
uint256 price;
bool sold;
}
event MarketItemCreated (
uint256 indexed tokenId,
address seller,
address owner,
uint256 price,
bool sold
);
constructor() ERC721("Metaverse Tokens", "METT") {
owner = payable(msg.sender);
}
/* Updates the listing price of the contract */
function updateListingPrice(uint _listingPrice) public payable {
require(owner == msg.sender, "Only marketplace owner can update listing price.");
listingPrice = _listingPrice;
}
/* Returns the listing price of the contract */
function getListingPrice() public view returns (uint256) {
return listingPrice;
}
/* Mints a token and lists it in the marketplace */
function createToken(string memory tokenURI, uint256 price) public payable returns (uint) {
_tokenIds.increment();
uint256 newTokenId = _tokenIds.current();
_mint(msg.sender, newTokenId);
_setTokenURI(newTokenId, tokenURI);
createMarketItem(newTokenId, price);
return newTokenId;
}
function createMarketItem(
uint256 tokenId,
uint256 price
) private {
require(price > 0, "Price must be at least 1 wei");
require(msg.value == listingPrice, "Price must be equal to listing price");
idToMarketItem[tokenId] = MarketItem(
tokenId,
payable(msg.sender),
payable(address(this)),
price,
false
);
_transfer(msg.sender, address(this), tokenId);
emit MarketItemCreated(
tokenId,
msg.sender,
address(this),
price,
false
);
}
/* allows someone to resell a token they have purchased */
function resellToken(uint256 tokenId, uint256 price) public payable {
require(idToMarketItem[tokenId].owner == msg.sender, "Only item owner can perform this operation");
require(msg.value == listingPrice, "Price must be equal to listing price");
idToMarketItem[tokenId].sold = false;
idToMarketItem[tokenId].price = price;
idToMarketItem[tokenId].seller = payable(msg.sender);
idToMarketItem[tokenId].owner = payable(address(this));
_itemsSold.decrement();
_transfer(msg.sender, address(this), tokenId);
}
/* Creates the sale of a marketplace item */
/* Transfers ownership of the item, as well as funds between parties */
function createMarketSale(
uint256 tokenId
) public payable {
uint price = idToMarketItem[tokenId].price;
address seller = idToMarketItem[tokenId].seller;
require(msg.value == price, "Please submit the asking price in order to complete the purchase");
idToMarketItem[tokenId].owner = payable(msg.sender);
idToMarketItem[tokenId].sold = true;
idToMarketItem[tokenId].seller = payable(address(0));
_itemsSold.increment();
_transfer(address(this), msg.sender, tokenId);
payable(owner).transfer(listingPrice);
payable(seller).transfer(msg.value);
}
/* Returns all unsold market items */
function fetchMarketItems() public view returns (MarketItem[] memory) {
uint itemCount = _tokenIds.current();
uint unsoldItemCount = _tokenIds.current() - _itemsSold.current();
uint currentIndex = 0;
MarketItem[] memory items = new MarketItem[](unsoldItemCount);
for (uint i = 0; i < itemCount; i++) {
if (idToMarketItem[i + 1].owner == address(this)) {
uint currentId = i + 1;
MarketItem storage currentItem = idToMarketItem[currentId];
items[currentIndex] = currentItem;
currentIndex += 1;
}
}
return items;
}
/* Returns only items that a user has purchased */
function fetchMyNFTs() public view returns (MarketItem[] memory) {
uint totalItemCount = _tokenIds.current();
uint itemCount = 0;
uint currentIndex = 0;
for (uint i = 0; i < totalItemCount; i++) {
if (idToMarketItem[i + 1].owner == msg.sender) {
itemCount += 1;
}
}
MarketItem[] memory items = new MarketItem[](itemCount);
for (uint i = 0; i < totalItemCount; i++) {
if (idToMarketItem[i + 1].owner == msg.sender) {
uint currentId = i + 1;
MarketItem storage currentItem = idToMarketItem[currentId];
items[currentIndex] = currentItem;
currentIndex += 1;
}
}
return items;
}
/* Returns only items a user has listed */
function fetchItemsListed() public view returns (MarketItem[] memory) {
uint totalItemCount = _tokenIds.current();
uint itemCount = 0;
uint currentIndex = 0;
for (uint i = 0; i < totalItemCount; i++) {
if (idToMarketItem[i + 1].seller == msg.sender) {
itemCount += 1;
}
}
MarketItem[] memory items = new MarketItem[](itemCount);
for (uint i = 0; i < totalItemCount; i++) {
if (idToMarketItem[i + 1].seller == msg.sender) {
uint currentId = i + 1;
MarketItem storage currentItem = idToMarketItem[currentId];
items[currentIndex] = currentItem;
currentIndex += 1;
}
}
return items;
}
}
In this contract we are inheriting from the ERC721 standard implemented by OpenZepplin
Now the smart contract code and environment is complete and we can try testing it out.
To do so, we can create a local test to run through much of the functionality, like minting a token, putting it up for sale, selling it to a user, and querying for tokens.
To create the test, open test/sample-test.js and update it with the following code:
View the gist here
/* test/sample-test.js */
describe("NFTMarket", function() {
it("Should create and execute market sales", async function() {
/* deploy the marketplace */
const NFTMarketplace = await ethers.getContractFactory("NFTMarketplace")
const nftMarketplace = await NFTMarketplace.deploy()
await nftMarketplace.deployed()
let listingPrice = await nftMarketplace.getListingPrice()
listingPrice = listingPrice.toString()
const auctionPrice = ethers.utils.parseUnits('1', 'ether')
/* create two tokens */
await nftMarketplace.createToken("https://www.mytokenlocation.com", auctionPrice, { value: listingPrice })
await nftMarketplace.createToken("https://www.mytokenlocation2.com", auctionPrice, { value: listingPrice })
const [_, buyerAddress] = await ethers.getSigners()
/* execute sale of token to another user */
await nftMarketplace.connect(buyerAddress).createMarketSale(1, { value: auctionPrice })
/* resell a token */
await nftMarketplace.connect(buyerAddress).resellToken(1, auctionPrice, { value: listingPrice })
/* query for and return the unsold items */
items = await nftMarketplace.fetchMarketItems()
items = await Promise.all(items.map(async i => {
const tokenUri = await nftMarketplace.tokenURI(i.tokenId)
let item = {
price: i.price.toString(),
tokenId: i.tokenId.toString(),
seller: i.seller,
owner: i.owner,
tokenUri
}
return item
}))
console.log('items: ', items)
})
})
Next, run the test from your command line:
npx hardhat test
If the test runs successfully, it should log out an array containing the two marketplace items.
Building the front end
Now that the smart contract is working and ready to go, we can start building out the UI.
The first thing we might think about is setting up a layout so that we can enable some navigation that will persist across all pages.
To set this up, open pages/_app.js and update it with the following code:
View the gist here
/* pages/_app.js */
import '../styles/globals.css'
import Link from 'next/link'
function MyApp({ Component, pageProps }) {
return (
<div>
<nav className="border-b p-6">
<p className="text-4xl font-bold">Metaverse Marketplace</p>
<div className="flex mt-4">
<Link href="/">
<a className="mr-4 text-pink-500">
Home
</a>
</Link>
<Link href="/create-nft">
<a className="mr-6 text-pink-500">
Sell NFT
</a>
</Link>
<Link href="/my-nfts">
<a className="mr-6 text-pink-500">
My NFTs
</a>
</Link>
<Link href="/dashboard">
<a className="mr-6 text-pink-500">
Dashboard
</a>
</Link>
</div>
</nav>
<Component {...pageProps} />
</div>
)
}
export default MyApp
The navigation has links for the home route as well as a page to sell an NFT, view the NFTs you have purchased, and a dashboard to see the NFTs you've listed.
Querying the contract for marketplace items
The next page we'll update is pages/index.js. This is the main entry-point of the app, and will be the view where we query for the NFTs for sale and render them to the screen.
View the gist here
/* pages/index.js */
import { ethers } from 'ethers'
import { useEffect, useState } from 'react'
import axios from 'axios'
import Web3Modal from 'web3modal'
import {
marketplaceAddress
} from '../config'
import NFTMarketplace from '../artifacts/contracts/NFTMarketplace.sol/NFTMarketplace.json'
export default function Home() {
const [nfts, setNfts] = useState([])
const [loadingState, setLoadingState] = useState('not-loaded')
useEffect(() => {
loadNFTs()
}, [])
async function loadNFTs() {
/* create a generic provider and query for unsold market items */
const provider = new ethers.providers.JsonRpcProvider()
const contract = new ethers.Contract(marketplaceAddress, NFTMarketplace.abi, provider)
const data = await contract.fetchMarketItems()
/*
* map over items returned from smart contract and format
* them as well as fetch their token metadata
*/
const items = await Promise.all(data.map(async i => {
const tokenUri = await contract.tokenURI(i.tokenId)
const meta = await axios.get(tokenUri)
let price = ethers.utils.formatUnits(i.price.toString(), 'ether')
let item = {
price,
tokenId: i.tokenId.toNumber(),
seller: i.seller,
owner: i.owner,
image: meta.data.image,
name: meta.data.name,
description: meta.data.description,
}
return item
}))
setNfts(items)
setLoadingState('loaded')
}
async function buyNft(nft) {
/* needs the user to sign the transaction, so will use Web3Provider and sign it */
const web3Modal = new Web3Modal()
const connection = await web3Modal.connect()
const provider = new ethers.providers.Web3Provider(connection)
const signer = provider.getSigner()
const contract = new ethers.Contract(marketplaceAddress, NFTMarketplace.abi, signer)
/* user will be prompted to pay the asking proces to complete the transaction */
const price = ethers.utils.parseUnits(nft.price.toString(), 'ether')
const transaction = await contract.createMarketSale(nft.tokenId, {
value: price
})
await transaction.wait()
loadNFTs()
}
if (loadingState === 'loaded' && !nfts.length) return (<h1 className="px-20 py-10 text-3xl">No items in marketplace</h1>)
return (
<div className="flex justify-center">
<div className="px-4" style={{ maxWidth: '1600px' }}>
<div className="grid grid-cols-1 sm:grid-cols-2 lg:grid-cols-4 gap-4 pt-4">
{
nfts.map((nft, i) => (
<div key={i} className="border shadow rounded-xl overflow-hidden">
<img src={nft.image} />
<div className="p-4">
<p style={{ height: '64px' }} className="text-2xl font-semibold">{nft.name}</p>
<div style={{ height: '70px', overflow: 'hidden' }}>
<p className="text-gray-400">{nft.description}</p>
</div>
</div>
<div className="p-4 bg-black">
<p className="text-2xl font-bold text-white">{nft.price} ETH</p>
<button className="mt-4 w-full bg-pink-500 text-white font-bold py-2 px-12 rounded" onClick={() => buyNft(nft)}>Buy</button>
</div>
</div>
))
}
</div>
</div>
</div>
)
}
When the page loads, we query the smart contract for any NFTs that are still for sale and render them to the screen along with metadata about the items and a button for purchasing them.
Creating and listing NFTs
Next, let's create the page that allows users to create and list NFTs.
There are a few things happening in this page:
- The user is able to upload and save files to IPFS
- The user is able to create a new NFT
- The user is able to set metadata and price of item and list it for sale on the marketplace
After the user creates and lists an item, they are re-routed to the main page to view all of the items for sale.
View the gist here
/* pages/create-nft.js */
import { useState } from 'react'
import { ethers } from 'ethers'
import { create as ipfsHttpClient } from 'ipfs-http-client'
import { useRouter } from 'next/router'
import Web3Modal from 'web3modal'
const client = ipfsHttpClient('https://ipfs.infura.io:5001/api/v0')
import {
marketplaceAddress
} from '../config'
import NFTMarketplace from '../artifacts/contracts/NFTMarketplace.sol/NFTMarketplace.json'
export default function CreateItem() {
const [fileUrl, setFileUrl] = useState(null)
const [formInput, updateFormInput] = useState({ price: '', name: '', description: '' })
const router = useRouter()
async function onChange(e) {
/* upload image to IPFS */
const file = e.target.files[0]
try {
const added = await client.add(
file,
{
progress: (prog) => console.log(`received: ${prog}`)
}
)
const url = `https://ipfs.infura.io/ipfs/${added.path}`
setFileUrl(url)
} catch (error) {
console.log('Error uploading file: ', error)
}
}
async function uploadToIPFS() {
const { name, description, price } = formInput
if (!name || !description || !price || !fileUrl) return
/* first, upload metadata to IPFS */
const data = JSON.stringify({
name, description, image: fileUrl
})
try {
const added = await client.add(data)
const url = `https://ipfs.infura.io/ipfs/${added.path}`
/* after metadata is uploaded to IPFS, return the URL to use it in the transaction */
return url
} catch (error) {
console.log('Error uploading file: ', error)
}
}
async function listNFTForSale() {
const url = await uploadToIPFS()
const web3Modal = new Web3Modal()
const connection = await web3Modal.connect()
const provider = new ethers.providers.Web3Provider(connection)
const signer = provider.getSigner()
/* create the NFT */
const price = ethers.utils.parseUnits(formInput.price, 'ether')
let contract = new ethers.Contract(marketplaceAddress, NFTMarketplace.abi, signer)
let listingPrice = await contract.getListingPrice()
listingPrice = listingPrice.toString()
let transaction = await contract.createToken(url, price, { value: listingPrice })
await transaction.wait()
router.push('/')
}
return (
<div className="flex justify-center">
<div className="w-1/2 flex flex-col pb-12">
<input
placeholder="Asset Name"
className="mt-8 border rounded p-4"
onChange={e => updateFormInput({ ...formInput, name: e.target.value })}
/>
<textarea
placeholder="Asset Description"
className="mt-2 border rounded p-4"
onChange={e => updateFormInput({ ...formInput, description: e.target.value })}
/>
<input
placeholder="Asset Price in Eth"
className="mt-2 border rounded p-4"
onChange={e => updateFormInput({ ...formInput, price: e.target.value })}
/>
<input
type="file"
name="Asset"
className="my-4"
onChange={onChange}
/>
{
fileUrl && (
<img className="rounded mt-4" width="350" src={fileUrl} />
)
}
<button onClick={listNFTForSale} className="font-bold mt-4 bg-pink-500 text-white rounded p-4 shadow-lg">
Create NFT
</button>
</div>
</div>
)
}
Viewing only the NFTs purchased by the user
In the NFTMarketplace.sol smart contract, we created a function named fetchMyNFTs
that only returns the NFTs owned by the user.
In pages/my-nfts.js, we will use that function to fetch and render them.
This functionality is different than the query main pages/index.js page because we need to ask the user for their address and use it in the contract, so the user will have to sign the transaction for it to be able to fetch them properly.
View the gist here
/* pages/my-nfts.js */
import { ethers } from 'ethers'
import { useEffect, useState } from 'react'
import axios from 'axios'
import Web3Modal from 'web3modal'
import { useRouter } from 'next/router'
import {
marketplaceAddress
} from '../config'
import NFTMarketplace from '../artifacts/contracts/NFTMarketplace.sol/NFTMarketplace.json'
export default function MyAssets() {
const [nfts, setNfts] = useState([])
const [loadingState, setLoadingState] = useState('not-loaded')
const router = useRouter()
useEffect(() => {
loadNFTs()
}, [])
async function loadNFTs() {
const web3Modal = new Web3Modal({
network: "mainnet",
cacheProvider: true,
})
const connection = await web3Modal.connect()
const provider = new ethers.providers.Web3Provider(connection)
const signer = provider.getSigner()
const marketplaceContract = new ethers.Contract(marketplaceAddress, NFTMarketplace.abi, signer)
const data = await marketplaceContract.fetchMyNFTs()
const items = await Promise.all(data.map(async i => {
const tokenURI = await marketplaceContract.tokenURI(i.tokenId)
const meta = await axios.get(tokenURI)
let price = ethers.utils.formatUnits(i.price.toString(), 'ether')
let item = {
price,
tokenId: i.tokenId.toNumber(),
seller: i.seller,
owner: i.owner,
image: meta.data.image,
tokenURI
}
return item
}))
setNfts(items)
setLoadingState('loaded')
}
function listNFT(nft) {
router.push(`/resell-nft?id=${nft.tokenId}&tokenURI=${nft.tokenURI}`)
}
if (loadingState === 'loaded' && !nfts.length) return (<h1 className="py-10 px-20 text-3xl">No NFTs owned</h1>)
return (
<div className="flex justify-center">
<div className="p-4">
<div className="grid grid-cols-1 sm:grid-cols-2 lg:grid-cols-4 gap-4 pt-4">
{
nfts.map((nft, i) => (
<div key={i} className="border shadow rounded-xl overflow-hidden">
<img src={nft.image} className="rounded" />
<div className="p-4 bg-black">
<p className="text-2xl font-bold text-white">Price - {nft.price} Eth</p>
<button className="mt-4 w-full bg-pink-500 text-white font-bold py-2 px-12 rounded" onClick={() => listNFT(nft)}>List</button>
</div>
</div>
))
}
</div>
</div>
</div>
)
}
Dashboard
The next page we will be creating is the dashboard that will allow users to view all of the items they have listed.
This page will be using the fetchItemsListed
function from the NFTMarketplace.sol smart contract which returns only the items that match the address of the user making the function call.
Create a new file called dashboard.js in the pages directory with the following code:
View the gist here
/* pages/dashboard.js */
import { ethers } from 'ethers'
import { useEffect, useState } from 'react'
import axios from 'axios'
import Web3Modal from 'web3modal'
import {
marketplaceAddress
} from '../config'
import NFTMarketplace from '../artifacts/contracts/NFTMarketplace.sol/NFTMarketplace.json'
export default function CreatorDashboard() {
const [nfts, setNfts] = useState([])
const [loadingState, setLoadingState] = useState('not-loaded')
useEffect(() => {
loadNFTs()
}, [])
async function loadNFTs() {
const web3Modal = new Web3Modal({
network: 'mainnet',
cacheProvider: true,
})
const connection = await web3Modal.connect()
const provider = new ethers.providers.Web3Provider(connection)
const signer = provider.getSigner()
const contract = new ethers.Contract(marketplaceAddress, NFTMarketplace.abi, signer)
const data = await contract.fetchItemsListed()
const items = await Promise.all(data.map(async i => {
const tokenUri = await contract.tokenURI(i.tokenId)
const meta = await axios.get(tokenUri)
let price = ethers.utils.formatUnits(i.price.toString(), 'ether')
let item = {
price,
tokenId: i.tokenId.toNumber(),
seller: i.seller,
owner: i.owner,
image: meta.data.image,
}
return item
}))
setNfts(items)
setLoadingState('loaded')
}
if (loadingState === 'loaded' && !nfts.length) return (<h1 className="py-10 px-20 text-3xl">No NFTs listed</h1>)
return (
<div>
<div className="p-4">
<h2 className="text-2xl py-2">Items Listed</h2>
<div className="grid grid-cols-1 sm:grid-cols-2 lg:grid-cols-4 gap-4 pt-4">
{
nfts.map((nft, i) => (
<div key={i} className="border shadow rounded-xl overflow-hidden">
<img src={nft.image} className="rounded" />
<div className="p-4 bg-black">
<p className="text-2xl font-bold text-white">Price - {nft.price} Eth</p>
</div>
</div>
))
}
</div>
</div>
</div>
)
}
Reselling a token
The final page we will be creating will allow users to resell an NFT they've purchased from someone else.
This page will be using the resellToken
function from the NFTMarketplace.sol smart contract.
View the gist here
/* pages/resell-nft.js */
import { useEffect, useState } from 'react'
import { ethers } from 'ethers'
import { useRouter } from 'next/router'
import axios from 'axios'
import Web3Modal from 'web3modal'
import {
marketplaceAddress
} from '../config'
import NFTMarketplace from '../artifacts/contracts/NFTMarketplace.sol/NFTMarketplace.json'
export default function ResellNFT() {
const [formInput, updateFormInput] = useState({ price: '', image: '' })
const router = useRouter()
const { id, tokenURI } = router.query
const { image, price } = formInput
useEffect(() => {
fetchNFT()
}, [id])
async function fetchNFT() {
if (!tokenURI) return
const meta = await axios.get(tokenURI)
updateFormInput(state => ({ ...state, image: meta.data.image }))
}
async function listNFTForSale() {
if (!price) return
const web3Modal = new Web3Modal()
const connection = await web3Modal.connect()
const provider = new ethers.providers.Web3Provider(connection)
const signer = provider.getSigner()
const priceFormatted = ethers.utils.parseUnits(formInput.price, 'ether')
let contract = new ethers.Contract(marketplaceAddress, NFTMarketplace.abi, signer)
let listingPrice = await contract.getListingPrice()
listingPrice = listingPrice.toString()
let transaction = await contract.resellToken(id, priceFormatted, { value: listingPrice })
await transaction.wait()
router.push('/')
}
return (
<div className="flex justify-center">
<div className="w-1/2 flex flex-col pb-12">
<input
placeholder="Asset Price in Eth"
className="mt-2 border rounded p-4"
onChange={e => updateFormInput({ ...formInput, price: e.target.value })}
/>
{
image && (
<img className="rounded mt-4" width="350" src={image} />
)
}
<button onClick={listNFTForSale} className="font-bold mt-4 bg-pink-500 text-white rounded p-4 shadow-lg">
List NFT
</button>
</div>
</div>
)
}
Running the project
To run the project, we will need to have a deploy script to deploy the smart contracts to the blockchain network.
Deploying the contracts to a local network
When we created the project, Hardhat created an example deployment script at scripts/sample-script.js.
To make the purpose of this script more clear, update the name of scripts/sample-script.js to scripts/deploy.js.
Next, update scripts/deploy.js with the following code:
const hre = require("hardhat");
const fs = require('fs');
async function main() {
const NFTMarketplace = await hre.ethers.getContractFactory("NFTMarketplace");
const nftMarketplace = await NFTMarketplace.deploy();
await nftMarketplace.deployed();
console.log("nftMarketplace deployed to:", nftMarketplace.address);
fs.writeFileSync('./config.js', `
export const marketplaceAddress = "${nftMarketplace.address}"
`)
}
main()
.then(() => process.exit(0))
.catch((error) => {
console.error(error);
process.exit(1);
});
This script will deploy the contract to the blockchain network and create a file named config.js that will hold the address of the smart contract after it's been deployed.
We will first test this on a local network, then deploy it to the Mumbai testnet.
To spin up a local network, open your terminal and run the following command:
npx hardhat node
This should create a local network with 20 accounts.
Next, keep the node running and open a separate terminal window to deploy the contract.
In a separate window, run the following command:
npx hardhat run scripts/deploy.js --network localhost
When the deployment is complete, the CLI should print out the address of the contract that was deployed:
You should also see the config.js file populated with this smart contract address.
Importing accounts into MetaMask
You can import the accounts created by the node into your Metamask wallet to try out in the app.
Each of these accounts is seeded with 10000 ETH.
To import one of these accounts, first switch your MetaMask wallet network to Localhost 8545.
Next, in MetaMask click on Import Account from the accounts menu:
Copy then paste one of the Private Keys logged out by the CLI and click Import. Once the account is imported, you should see some the Eth in the account:
I'd suggest doing this with 2 or 3 accounts so that you have the ability to test out the various functionality between users.
Running the app
Now we can test out the app!
To start the app, run the following command in your CLI:
npm run dev
To test everything out, try listing an item for sale, then switching to another account and purchasing it.
Deploying to Polygon
Now that we have the project up and running and tested locally, let's deploy to Polygon. We'll start by deploying to Mumbai, the Polygon test network.
The first thing we will need to do is save one of our private keys from our wallet as an environment variable.
To get the private key, you can use one of the private keys given to you by Hardhat or you can export them directly from MetaMask.
If you are on a Mac, you can set an environment variable from the command line like so (be sure to run the deploy script from this same terminal and session):
export privateKey="your-private-key"
Private keys are never meant to be shared publicly under any circumstance. It is advised never to hardcode a private key in a file. If you do choose to do so, be sure to use a testing wallet and to never under any circumstances push a file containing a private key to source control or expose it publicly.
Configuring the network
Next, we need to switch from the local test network to the Mumbai Testnet.
To do so, we need to create and set the network configuration.
First, open MetaMask and click on Settings.
Next, click on Networks and then Add Network:
Here, we will add the following configurations for the Mumbai test network as listed here:
Network Name: Mumbai TestNet
New RPC URL: https://rpc-mumbai.maticvigil.com
Chain ID: 80001
Currency Symbol: Matic
Save this, then you should be able to switch to and use the new network!
Finally, you will need some testnet Matic tokens in order to interact with the applications.
To get these, you can visit the Matic Faucet, inputting the address of the wallets that you would like to request the tokens.
Deploying to the Matic / Polygon network
Now that you have some Matic tokens, you can deploy to the Polygon network!
To do so, be sure that the address associated with the private key you are deploying your contract with has received some Matic tokens in order to pay the gas fees for the transaction.
Also, be sure to uncomment the mumbai
configuration in hardhat.config.js:
mumbai: {
url: "https://rpc-mumbai.maticvigil.com",
accounts: [process.env.privateKey]
}
To deploy to Matic, run the following command:
npx hardhat run scripts/deploy.js --network mumbai
If you run a deployment error, the public RPC may be congested. In production, it's recommended to use an RPC provider like Infura, Alchemy, Quicknode, or Figment DataHub.
Once the contracts have been deployed, update the loadNFTs
function call in pages/index.js to include the new RPC endpoint:
/* pages/index.js */
/* old provider */
const provider = new ethers.providers.JsonRpcProvider()
/* new provider */
const provider = new ethers.providers.JsonRpcProvider("https://rpc-mumbai.maticvigil.com")
You should now be able to update the contract addresses in your project and test on the new network 🎉!
npm run dev
If you run into an error, the contract address printed out to the console by hardhat could be incorrect due to a bug I've run into recently. You can get the correct contract addresses by visiting https://mumbai.polygonscan.com/ and pasting in the address from which the contracts were deployed to see the most recent transactions and getting the contract addresses from the transaction data.
Deploying to Mainnet
To deploy to the main Matic / Polygon network, you can use the same steps we set up for the Mumbai test network.
The main difference is that you'll need to use an endpoint for Matic as well as import the network into your MetaMask wallet as listed here.
An example update in your project to make this happen might look like this:
/* hardhat.config.js */
/* adding Matic main network config to existing config */
...
matic: {
url: "https://rpc-mainnet.maticvigil.com",
accounts: [privateKey]
}
...
Public RPCs like the one listed above may have traffic or rate-limits depending on usage. You can sign up for a dedicated free RPC URL using services like Infura, MaticVigil, QuickNode, Alchemy, Chainstack, or Ankr.
For example, using something like Infura:
url: `https://polygon-mainnet.infura.io/v3/${infuraId}`
To view the final source code for this project, visit this repo
Next steps
Congratulations! You've deployed a non-trivial app to Polygon.
The coolest thing about working with solutions like Polygon is how little extra work or learning I had to do compared to building directly on Ethereum. Almost all of the APIs and tooling in these layer 2's and sidechains remain the same, making any skills transferable across various platforms like Polygon.
For the next steps, I'd suggest porting over the queries implemented in this app using The Graph. The Graph will open up many more data access patterns including things like pagination, filtering, and sorting which are necessary for any real-world application.
I will also be publishing a tutorial showing how to use Polygon with The Graph in the coming weeks.
Latest comments (359)
Hello World
I am facing some npm dependency issues. Has anyone else encountered the following errors? If so, could you please tell me how to resolve them?
ERR! code ERESOLVE
npm ERR! ERESOLVE could not resolve
npm ERR!
npm ERR! While resolving: react-photo-gallery@8.0.0
npm ERR! Found: react@17.0.2
npm ERR! node_modules/react
npm ERR! react@"^17.0.2" from the root project
npm ERR! peer react@">=16.8.0" from @emotion/react@11.10.5
npm ERR! node_modules/@emotion/react
npm ERR! @emotion/react@"^11.4.0" from react-awesome-reveal@3.8.1
npm ERR! node_modules/react-awesome-reveal
npm ERR! react-awesome-reveal@"^3.8.1" from the root project
npm ERR! @emotion/react@"^11.1.1" from react-select@4.3.1
npm ERR! node_modules/react-select
npm ERR! react-select@"^4.3.1" from the root project
npm ERR! 31 more (@emotion/use-insertion-effect-with-fallbacks, ...)
npm ERR!
npm ERR! Could not resolve dependency:
npm ERR! peer react@"^16.8.0" from react-photo-gallery@8.0.0
npm ERR! node_modules/react-photo-gallery
npm ERR! react-photo-gallery@"^8.0.0" from the root project
npm ERR!
npm ERR! Conflicting peer dependency: react@16.14.0
npm ERR! node_modules/react
npm ERR! peer react@"^16.8.0" from react-photo-gallery@8.0.0
npm ERR! node_modules/react-photo-gallery
npm ERR! react-photo-gallery@"^8.0.0" from the root project
npm ERR!
npm ERR! Fix the upstream dependency conflict, or retry
npm ERR! this command with --force, or --legacy-peer-deps
npm ERR! to accept an incorrect (and potentially broken) dependency resolution.
Has anyone received the following error? Ran into it while trying out the UI locally after running the deploy script for the first time
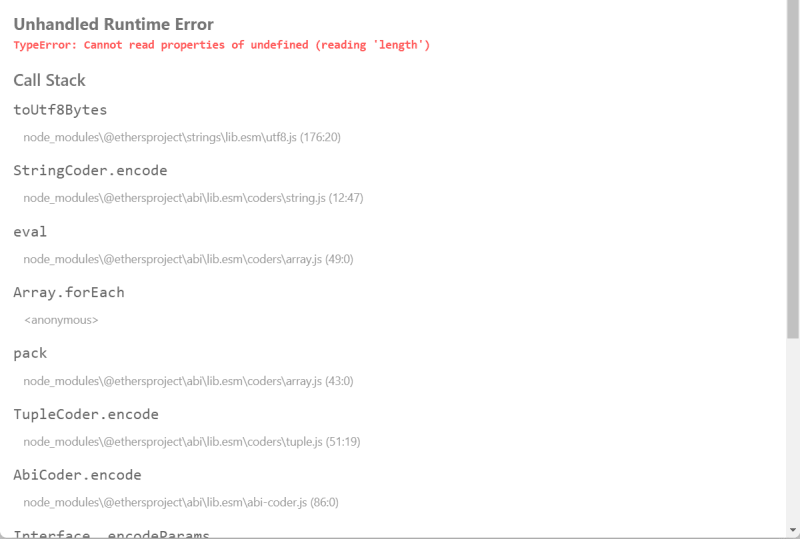
hello guys have been stuck for days trying to fix this
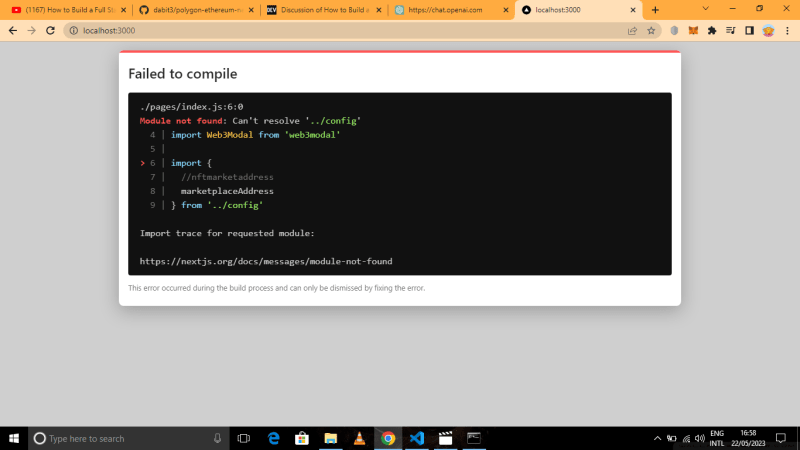
hello guys i was trying to deploy this NFTMarketplace.sol contract to sepolia test network but got this error ready - started server on 0.0.0.0:3000, url: localhost:3000
info - Loaded env from C:\Users\MOKUA\Downloads\my project\polygon-ethereum-nextjs-marketplace.env
info - Using webpack 5. Reason: Enabled by default nextjs.org/docs/messages/webpack5
event - compiled successfully
event - build page: /
wait - compiling...
error - ./pages/index.js:6:0
Module not found: Can't resolve '../config'
4 | import Web3Modal from 'web3modal'
5 |
Import trace for requested module:
nextjs.org/docs/messages/module-no...
event - build page: /next/dist/pages/_error
wait - compiling...
error - ./pages/index.js:6:0
Module not found: Can't resolve '../config'
4 | import Web3Modal from 'web3modal'
5 |
Import trace for requested module:
nextjs.org/docs/messages/module-no... .kindly help
Has anyone when sell nft received the following error?
Can anyone help me fast, im getting this error
Hey,
the following method is clear to me:
const data = await contract.fetchMarketItems();
But how do I find only one item for Id, e.g.:
const data = await contract.fetchMarketItem(identId);
404 This page could not be found.
when clicking on SellNFT button I am receiving the above error. Please let me know if anyone has idea why I'm getting this error!!
Any ideas why this is happening? I am trying to create the nft, but seems like all the pages throw a 404 (home, create, etc)
Hi there! When using the input to upload the image I keep getting this from the server: basic auth failure: invalid project id or project secret
any ideas?
Error: call revert exception (method="getListingPrice()", errorArgs=null, errorName=null, errorSignature=null, reason=null, code=CALL_EXCEPTION, version=abi/5.5.0)
How to fix error above? Thanks a lot
Hey Guys,
I have been getting this issue after running my localhost once i've deployed the nft to mumbai
Error: missing revert data in call exception; Transaction reverted without a reason string See: links.ethers.org/v5-errors-CALL_EXCEPTION
Any possible solutions for this?
Is this marketplace something like developed digital twin of a real one?
Unhandled Runtime Error
AxiosError: Network Error
Call Stack
XMLHttpRequest.handleError
node_modules/axios/lib/adapters/xhr.js (154:0)
Unhandled Runtime Error
Error: Invariant: attempted to hard navigate to the same URL /create-nft.js http://localhost:3000/create-nft.js
getting this error