JavaScript was introduced in 1995 and its standards are actively being maintained by ECMA International - hence the name ECMAScript for JavaScript. Since 2015, major versions of the JavaScript language are being introduced every year in June. So JavaScript is constantly evolving, and new features are regularly being added to the language. Are you looking to take your JavaScript skills to the next level with all the relevant new features? Whether you're a beginner or an experienced developer, there are always ways to improve and learn new techniques. In this blog post, we'll explore six essential tips that can help you level up your JavaScript skills. For each tip, we'll also point out which ECMAScript version is required to be able to use it.
1. Optional Chaining
Have you tried accessing nested properties inside an object in which the intermediate properties might be undefined? To avoid your code from throwing errors like Cannot read properties of undefined
, we write very convoluted statements wrapped with && operators like this:
if (request.body && request.body.product && request.body.product.price) {
console.log(request.body.product.price);
}
JavaScript has a feature called optional chaining which is a way to access or modify properties of objects without worrying if an intermediate property is undefined. The statement will simply return undefined without throwing an error and ending your code execution prematurely. This optional chaining allows you to write cleaner, more concise code. By using optional chaining, you can avoid having to write multiple checks to verify the existence of an object before attempting to access it.
Rewriting the same if statement with optional chaining will look like this:
if (request?.body?.product?.price) {
console.log(request.body.product.price);
}
This feature was introduced in ES2020.
2. Nullish coalescing operator
When you want to assign a value to a variable, you might be placing null and undefined checks to make sure that you’re retrieving the intended value. If that’s not the case, we might want to provide a fallback value in that case. Such a code sample could look like this:
const userName = (user?.name !== null && user?.name !== undefined) ?
user.name :
'Anonymous';
In JavaScript, there is a nullish coalescing operator (??) that allows developers to provide a fallback value when working with null or undefined values. This operator can be used to check for null or undefined values and provide an alternative value when the initial value is null or undefined.
For example, you can use the nullish coalescing operator to simplify the code sample from above like this:
const userName = user?.name ?? 'Anonymous';
This feature was introduced in ES2020.
3. Maps Instead of For Loop
When you start a JavaScript programming course, one of the first topics typically taught is for loops. For loops are a type of loop that repeats a set of instructions a certain number of times. They are used to iterate over data structures like arrays or objects and can be used to perform a wide variety of tasks.
If you want to iterate through an array and store the value of each number squared in a new array, it would look like this with a for loop:
const numbers = [1, 2, 3, 4, 5];
const squares = [];
for (let i = 0; i < numbers.length; i++) {
squares.push(numbers[i]**2);
}
With the introduction of newer, more modern features in JavaScript, for loops are not always the best option for some tasks. Maps are a powerful data structure that can often be used instead of for loops to improve performance. The map() method takes a callback function as its argument and returns an array with the results of calling the callback on each element in the array. This allows you to quickly and easily iterate over an array without having to write a loop.
The benefit of using a Map is that it doesn’t cause side effects in your code and always allows you to create a new array. The resulting code with a Map would look like this:
const numbers = [1, 2, 3, 4, 5];
const squares = numbers.map(number => number ** 2);
This feature was introduced in ES2015 or ES6.
4. Arrow Functions
Arrow functions are a concise way to define functions in JavaScript, and they have several benefits over traditional function declarations. Arrow functions have a shorter syntax than regular functions, which can make your code more readable. Additionally, arrow functions are lexically scoped, meaning that the value of the this keyword inside an arrow function is the same as the value outside of it. This can be very helpful when working with objects and methods.
For example, consider the following regular function:
function add(a, b) {
return a + b;
}
This can be written as an arrow function like this:
const add = (a, b) => a + b;
This feature was introduced in ES2015 or ES6.
5. Object Destructuring
Object destructuring is a feature of JavaScript that allows you to quickly and easily extract values from an object. It allows you to create variables that contain the values of specific properties in an object, which can be useful when you need to access the same properties repeatedly.
For example, consider the following code:
const product = {
name: "iPhone",
color: "purple",
price: 1000
};
const name = product.name;
const color = product.color;
const price = product.price;
Using object destructuring, you can extract the values from the user object and assign them to variables like this:
const product = {
name: "iPhone",
color: "purple",
price: 1000
};
const { name, color, price } = product;
This feature was introduced in ES2015 or ES6.
6. Object literal enhancement
Now that you know what Object destructuring is, it’s time to take a look at object literal enhancement. This is a feature of JavaScript that is the exact opposite of object destructuring. It allows you to grab variables and create objects with fewer lines of code. For example, consider the following code:
const name = "iPhone";
const color = "purple";
const price = 1000;
const product = {
name: name,
color: color,
price: price
};
Using object literal enhancement, you can simplify this code to:
const name = "iPhone";
const color = "purple";
const price = 1000;
const product = { name, color, price };
This feature was introduced in ES2015 or ES6.
Conclusion
JavaScript is constantly getting enriched by new features every year. By following these up-to-date tips and continuing to learn and grow, you can level up your JavaScript skills and become a more proficient and successful developer. Don't be afraid to challenge yourself and try new things – it's the best way to improve and keep up with the ever-changing world of technology. Happing coding! 😎
If the content was helpful, feel free to support me here:
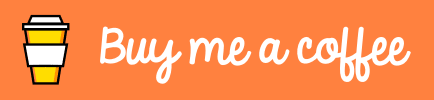
Top comments (0)