Note: This is a rough note on my journey in learning Vim, Vi and NeoVim. Note that they are not in particular order
Install neovim: https://neovim.io/
Install vim-plug: https://github.com/junegunn/vim-plug
Vim 101
Types of Mode
- Normal mode
- Insert mode
- Visual mode
- Command mode
Motions
- [x]
k
- Upward - [x]
j
- Downward - [x]
l
- Right - [x]
h
- Left - [x]
w
- Hop forward between words - [x]
b
- Hop backward between words - [x]
e
- Move to the end of line - [x]
~
- swap to upper or lower case - [x]
g~w
- apply Uppercase to a world - [x]
g~it
- it is inner tag, applies uppercase to everything inside a tag - [x]
%
- jump between matching curly braces, square brackets or () - [x]
gx
- open up the link to a browse - [x]
gf
- open the file path i.e if you have a path ./index.js, put your cursor on it and type gf -[x]gx
- open a link under a cursor -[x]*
search for words under cursor and use Shift N or n to toggle between words
Additional Modes and Concepts:
- Replace mode (R): Replace characters as you type.
- Visual Block mode (Ctrl+v): Select text as a block (useful for editing columns).
- Insert mode with Auto-Completion (Ctrl+n/Ctrl+p): Auto-complete words in the document when in insert mode.
Additional Motions:
- 0 (zero): Move to the beginning of the line.
- $: Move to the end of the line.
- gg: Move to the beginning of the file.
- G: Move to the end of the file.
- H: Move to the top of the screen.
- M: Move to the middle of the screen.
- L: Move to the bottom of the screen. Editing:
- J: Join the current line with the next one. r + : Replace the current character with .
- yw: Yank (copy) a word.
- Y: Yank (copy) a whole line.
- p: Paste after the cursor.
- P: Paste before the cursor.
Buffers & Tabs:
: Close the current buffer.
: List all open buffers.
(:bn) /
(:bp): Switch to the next/previous buffer.
- gt/gT: Switch between open tabs.
Visual Mode Enhancements:
- vip: Select the paragraph around the cursor.
- vi{: Select everything inside {}.
- **vi[orvi]`: Select everything inside brackets.
Search and Replace:
- /old/new/g: Substitute all occurrences of "old" with "new" in the current line.
- :%s/old/new/g: Substitute all occurrences of "old" with "new" in the entire file.
- :%s/old/new/gc: Same as above but with confirmation before replacing.
- : Turn off search highlighting.
```
Macros:
```vim
- q{register}: Start recording a macro in the specified register.
- @{register}: Replay the recorded macro in that register.
Registers:
- "0p: Paste the most recent yanked text.
- "1p, "2p, ...: Access numbered registers (for older yanks/deletes).
- : View the contents of registers.
```
Working with Indentation:
=: Automatically indent lines (use gg=G for the entire file).
Other Useful Commands:
```
- :!{command}: Run an external command.
- Ctrl+o: Go back to the previous position (useful when navigating between different areas).
- Ctrl+i: Go forward to the next position.
- Ctrl+^: Switch between the current and previous file.
```
#### Command + Count + Motion
- 8k - Jump up 8 lines
- 16j - Jump down 16 lines
- d$ - delete to the end of the line
- de - delete to the end of the word
- dG - delete to the end of the file
---
#### Explorer
- :Ex or :Explore: Opens the file explorer in the current directory.
- :Sex: Opens the file explorer in a horizontal split.
- :Vex: Opens the file explorer in a vertical split.
- SHIFT + % - Create a new file in :Ex mode
#### Navigation within Netrw:
- Enter: Opens a file or navigates into a directory.
- - (hyphen): Goes up one directory level.
- r: Refreshes the directory view.
- i: Toggles between different views (tree view, detailed view, etc.).
- q: Closes the explorer.
---
#### Search
- :set hlsearch - Enable search highlighting
- :noh - disable search highlighting
---
#### Skip code block
- } - skip code block
- { - skip code block
- ctrl+e / ctrl+y - Scroll down/up one line
- Ctrl+d - Move down half a page
- Ctrl+u - Move up half a page
---
##### Delete and Change text in command mode
- dd - delete a single line
- x - delete while in command mode
- r - change a text while in command mode
- u - undo
- Ctrl + r - Redo
- d3j - delete 2 words down
- d2w - delete 2 words forwars
- db - delete backward
- d2j - delete 2 words doward
- c - Delete, then start insert mode
- cc - Delete line, then start insert mode
- cw - Change words
---
##### INDENT
- `>>` Indent forward
- `<<` Indent backward
---
#### Quit and Save
- :qa - Quit all open buffers
- :wa - Write all open buffers
- :wqa - Write and quit all open buffers
- :q - Quit current buffer
- :w - Write current buffer
- :wq - Write and quit current buffer
- :ZZ - quit vim (quit with saving)
- :ZQ - quit without saving
##### Select content inside () and {}
- :vib - select content inside ()
- :viB - select content inside {}
- :cib - change everything inside ()
- :ciB - change everything inside {}
---
#### Combinations
```bash
vaw - Selects a word under the cursor.
v enters Visual mode, and aw selects a word (including trailing whitespace).
va) - Selects text within parentheses, including the parentheses themselves.
v enters Visual mode, a selects "around" (inclusive), and ) specifies the target.
ciw - Changes the entire word under the cursor.
c enters Insert mode after deleting the selected text, and iw selects the inner word.
diw - Delete inner words
caw - Change around words
di( - Deletes text within parentheses, excluding the parentheses.
d deletes the selected text, i specifies the "inner" target, and ( selects the parentheses.
ci" - Changes text within double quotes, excluding the quotes.
c enters Insert mode after deleting the selected text, i specifies the "inner" target, and " selects the quotes.
```
---
#### Marks
Setting a Mark: Press "m" with any letter or character
e.g
ma to set a mark for location a
mb to set a mark for location b
To go location a, press 'a
To go location b, press 'b
#### File Tabs
- :e filename - Find and open a file in a new buffer
- :tabe - Make a new tab
- :vsp - Vertically split windows and open a file
- ctrl+ws - Split windows horizontally
- ctrl+wv - Split windows vertically
- ctrl+ww - Switch between windows in normal mode
---
#### Insert mode
- I - insert to the beginning of the line
- i - insert to the left of the word
- a - insert to the right after the word
- A - insert to the end of the line
- esc - Leave insert mode
- Ctrl + c - leave insert mode method 2
- a - insert to the other side
- o - insert a new line and enter into insert mode
- O - insert a new line above
- c - change mode
- . - repeat an action multiple times
- / - search for something in the file
e.g /console.log
Then use `n` to jump to the desired match, Shift N to jump back
- : - run a command
- n - Repeat search in same direction
- N - Repeat search in opposite direction
---
##### Visual mode
- v - visual mode
- vw - highlight the word forward
- y - yank (copy)
- p - paste below
- P - paste above a line
- shift + p - Paste the copied line(s) above the current line.
- shift + v - Visual line mode
- y5j - copy 5 lines down from where you are
- d5j - delete 5 lines downward
- viw - copy single word
---
###### Set number
> :set number
---
#### Run command from vim
> :!ls
> :!node app.js
---
##### Quit and saving
- :q - quit without saving changes
- :q! - quit and save changes
- :wq - write and quit with changes changed
- :qa - Quit all tabs and terminal
---
##### Jump to line
- :2 - go to line 2
- :3 - go to line 3
---
#### Resize terminal
- :resize {number_of_lines} for vertical resize
- :vertical resize 50
- :vertical resize {number_of_columns}
- :resize 10
#### Neovim
Install neovim:
- [x] Open the power-shell in administrator mode
- [X] Choco install neovim and also choco install vim-plug
- [X] Check if neovim is installed, run: `nvim` in your terminal
- [X] open your run command and type `%appdata%`in /AppData/Local/nvim or ~/.config/nvim/init.vim
- [X] Press cmd on top and navigate to command mode
- [X] cd .. away from the Roaming folder
- [X] cd Local
- [X] create a new folder called `nvim`
- [X] Create a file called `init.vim`
- [X] paste the following config there
---
#### My Neovim config: init.vim
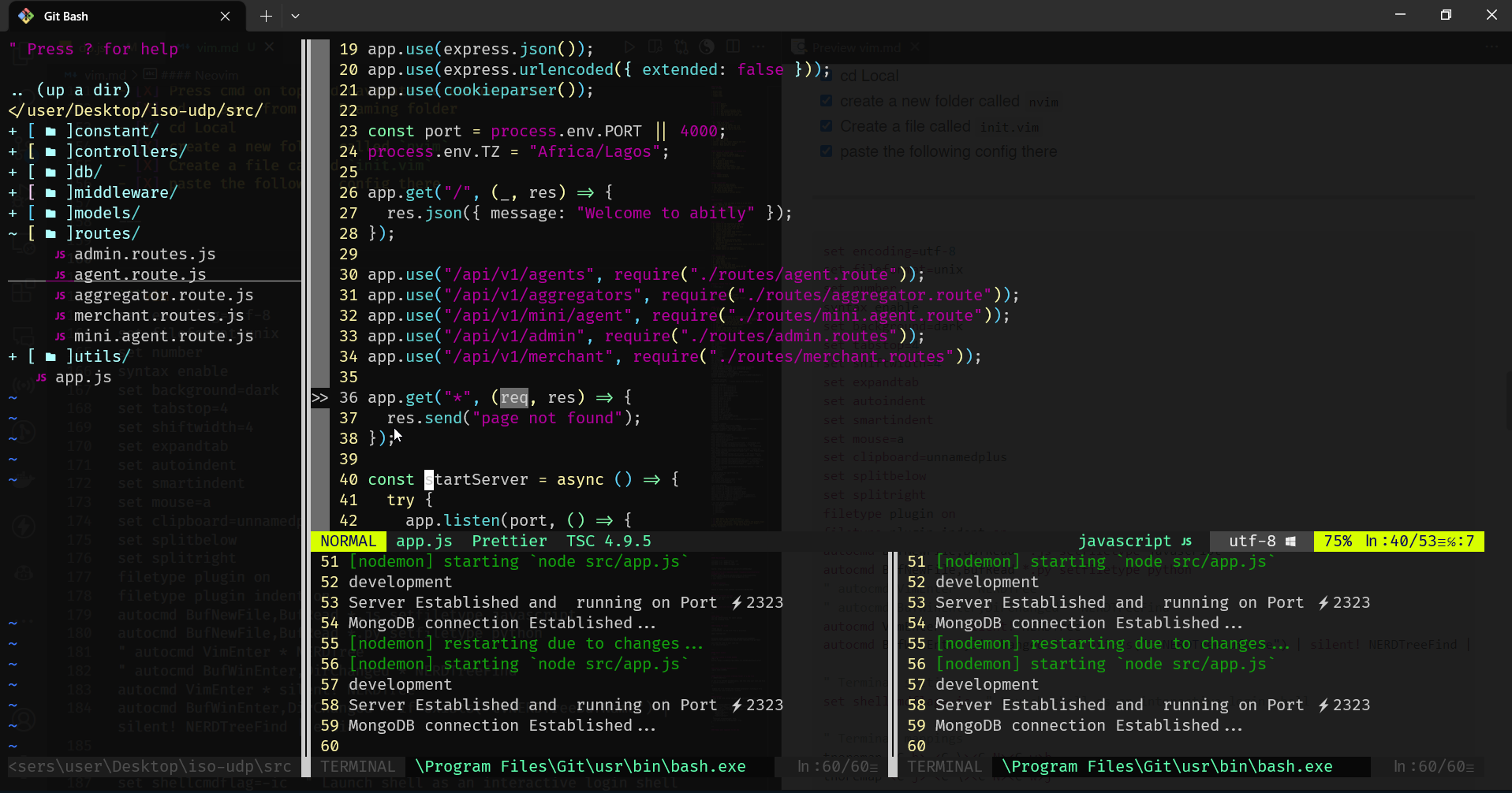
```vim
set t_Co=256
set foldmethod=indent
set foldlevel=99
set encoding=utf-8
set fileformat=unix
set modifiable
set number
syntax enable
set background=dark
set tabstop=4
set shiftwidth=4
set expandtab
set autoindent
set smartindent
set mouse=a
set clipboard=unnamedplus
set splitbelow
set splitright
set termguicolors
set incsearch
set wrap
set scrolloff=8
set sidescrolloff=8
set sidescroll=1
set nomodeline
set wildmenu
set complete-=i
set autoread
set viminfo^=!
set listchars=tab:,nbsp:_,trail:,extends:>,precedes:<
setlocal spell spelllang=en "Set spell check language to en
set list " Highlight non whitespace characters
set showmatch
set laststatus=2 " Always show status line
set cursorline
" set exrc " Use vimrc from local dir
" set hidden " Enable switching with modified buffers
" set undofile " Enable persistent undos across file open and closeasa
" setlocal spell spelllang=en "Set spell check language to en
" setlocal spell! " Disable spellchecking by default
" set nrformats-=octal " 007 != 010
" set sessionoptions-=options
" set viewoptions-=option
filetype plugin on
filetype indent plugin on
autocmd BufNewFile,BufRead *.js setfiletype javascript
autocmd BufNewFile,BufRead *.py setfiletype python
autocmd VimEnter * silent! NERDTree
autocmd BufWinEnter,DirChanged * if exists("t:NERDTreeBufName") | silent! NERDTreeFind | endif
autocmd TermOpen * tnoremap <buffer> <Esc> <C-\><C-n>
" Terminal settings
" set shell=set shellcmdflag=-ic
set shellcmdflag=-ic " Launch shell as an interactive login shell
" Save and source the init.vim file
command! Reload source $MYVIMRC
" Terminal mappings
tnoremap <C-h> <C-\><C-N><C-w>h
tnoremap <C-j> <C-\><C-N><C-w>j
tnoremap <C-k> <C-\><C-N><C-w>k
tnoremap <C-l> <C-\><C-N><C-w>l
nnoremap <C-f> :NERDTreeFocus<CR>
nnoremap <C-n> :NERDTree<CR>
nnoremap <C-t> :NERDTreeToggle<CR>
nnoremap <leader>w :w<CR>
nnoremap <leader>q :q<CR>
inoremap <Esc> <C-\><C-n>
nnoremap <M-h> <C-w>h
nnoremap <M-j> <C-w>j
nnoremap <M-k> <C-w>k
nnoremap <M-l> <C-w>l
nnoremap <C-Left> <C-w>h
nnoremap <C-Down> <C-w>j
nnoremap <C-Up> <C-w>k
nnoremap <C-Right> <C-w>l
nnoremap <C-v> <C-\><C-N>pi
nnoremap <F5> :NERDTreeRefresh<CR>
inoremap <CR> <C-g>u<CR>
inoremap <silent><expr> <CR> coc#pum#visible() ? coc#pum#confirm() : "\<CR>"
nmap <leader>i :AutoImportToggle<CR>
noremap <CapsLock> <Esc>
nnoremap <leader>f :FZF<CR>
" Terminal commands
command! Terminal split term://$SHELL
command! VTerminal vsplit term://$SHELL
" nnoremap <leader>t :split term://$SHELL<CR>
" Toggle terminal split
nnoremap <leader>t :call ToggleTerminal()<CR>
function! ToggleTerminal()
if exists('t:term_id') && !term_getjob(t:term_id)
" Terminal exists but the job has finished, close the terminal buffer
call term_close(t:term_id)
return
endif
if &buftype ==# 'terminal'
" Current buffer is a terminal, close it
quit
return
endif
" Open a new terminal split
split term://$SHELL
endfunction
" Key mappings for coc.nvim for go to definition
nmap <silent> gd <Plug>(coc-definition)
nmap <silent> gy <Plug>(coc-type-definition)
nmap <silent> gr <Plug>(coc-references)
nmap <silent> [g <Plug>(coc-diagnostic-prev)
nmap <silent> ]g <Plug>(coc-diagnostic-next)
nnoremap <silent> <space>s :<C-u>CocList -I symbols<cr>
nnoremap <silent> <space>d :<C-u>CocList diagnostics<cr>
nmap <leader>do <Plug>(coc-codeaction)
nmap <leader>rn <Plug>(coc-rename)
" Move current line up
nnoremap <leader>k :m-2<CR>==
vnoremap <leader>k :m-2<CR>gv=gv
" Move current line down
nnoremap <leader>j :m+<CR>==
vnoremap <leader>j :m+<CR>gv=gv
call plug#begin()
Plug 'folke/tokyonight.nvim'
Plug 'windwp/nvim-autopairs', {'do': ':UpdateRemotePlugins'}
Plug 'luochen1990/rainbow'
Plug 'sheerun/vim-polyglot'
Plug 'vimlab/split-term.vim'
Plug 'neoclide/coc.nvim', {'branch': 'release'}
Plug 'neovim/nvim-lspconfig'
Plug 'kassio/neoterm'
Plug 'http://github.com/tpope/vim-surround' " Surrounding ysw)
Plug 'https://github.com/preservim/nerdtree' " NerdTree
Plug 'https://github.com/tpope/vim-commentary' " For Commenting gcc & gc
Plug 'https://github.com/vim-airline/vim-airline' " Status bar
Plug 'vim-airline/vim-airline-themes'
Plug 'https://github.com/lifepillar/pgsql.vim' " PSQL Pluging needs :SQLSetType pgsql.vim
Plug 'https://github.com/ap/vim-css-color' " CSS Color Preview
Plug 'https://github.com/rafi/awesome-vim-colorschemes' " Retro Scheme
Plug 'https://github.com/neoclide/coc.nvim' " Auto Completion
Plug 'https://github.com/github/copilot.vim.git'
Plug 'https://github.com/ryanoasis/vim-devicons' " Developer Icons
Plug 'https://github.com/tc50cal/vim-terminal' " Vim Terminal
Plug 'https://github.com/preservim/tagbar' " Tagbar for code navigation
Plug 'https://github.com/terryma/vim-multiple-cursors' " CTRL + N for multiple cursors
Plug 'airblade/vim-gitgutter'
Plug 'neovim/nvim-lspconfig'
Plug 'hrsh7th/nvim-compe' "Plugin for auto completion
Plug 'https://github.com/mbbill/undotree'
Plug 'https://github.com/tpope/vim-fugitive'
Plug 'https://github.com/junegunn/fzf.vim' " Fuzzy Finder, Needs Silversearcher-ag for :Ag
Plug 'https://github.com/junegunn/fzf' "To use :FZF
Plug 'https://github.com/glepnir/dashboard-nvim'
Plug 'https://github.com/preservim/tagbar', {'on': 'TagbarToggle'} " Tagbar for code navigation
Plug 'https://github.com/dkarter/bullets.vim'
Plug 'jiangmiao/auto-pairs'
Plug 'alvan/vim-closetag'
Plug 'junegunn/rainbow_parentheses.vim'
Plug 'junegunn/limelight.vim'
Plug 'junegunn/vim-journal'
Plug 'joshdick/onedark.vim'
call plug#end()
colorscheme onedark
" Configuration for split-term.vim
" Plugin settings
" set splitright
" set splitbelow
let g:split_term_default_shell = "bash"
let g:split_term_vertical = 0
let g:disable_key_mappings = 0
let g:autoimport#mapping_key = '<C-space>'
let g:autoimport#enable_default_mappings = 1
let g:rainbow_active = 1 "set to 0 if you want to enable it later via :RainbowToggle
" airline setup
let g:airline_left_sep = ''
let g:airline_left_alt_sep = ''
let g:airline_right_sep = ''
let g:airline_right_alt_sep = ''
let g:airline_theme = 'dark'
let g:airline#extensions#ale#enabled = 1
let g:NERDTreeShowHidden = 1
" Coc.nvim mappings
nmap <silent> gd <Plug>(coc-definition)
nmap <silent> gy <Plug>(coc-type-definition)
nmap <silent> gr <Plug>(coc-references)
nmap <silent> [g <Plug>(coc-diagnostic-prev)
nmap <silent> ]g <Plug>(coc-diagnostic-next)
nnoremap <silent> <space>s :<C-u>CocList -I symbols<cr>
nnoremap <silent> <space>d :<C-u>CocList diagnostics<cr>
nmap <leader>do <Plug>(coc-codeaction)
nmap <leader>rn <Plug>(coc-rename)
nmap <leader>i :AutoImportToggle<CR>
" traverse in insert mode
inoremap <C-h> <Left>
inoremap <C-j> <Down>
inoremap <C-k> <Up>
inoremap <C-l> <Right>
" map the CapsLock key to Esc
imap jk <Esc>
imap kj <Esc>
" Coc.nvim global extensions
let g:coc_global_extensions = [
\ 'coc-snippets',
\ 'coc-pyright',
\ 'coc-html',
\ 'coc-markdownlint',
\ 'coc-yaml',
\ 'coc-tsserver',
\ 'coc-json',
\ 'coc-css',
\ 'coc-prettier'
\ ]
function! SemshiBufWipeout()
" Save the buffer to a file
let filename = '/tmp/buffer-' . bufnr('%') . '.txt'
writefile getline(1,"$") filename
endfunction
" auto save when leaving insert mode
nnoremap <silent> <Esc> :update<CR>
inoremap <silent> jk <Esc>:update<CR>
let g:onedark_color_overrides = {
\ "comment_grey": {"gui": "#69747C","cterm": "245", "cterm16": "8"},
\ "gutter_fg_grey": { "gui": "#69747C", "cterm": "245", "cterm16": "8"}
\}
```
---
##### Key binding
```
nnoremap <c-f> :NERDTrueFocus <CR>
nnoremap <c-n> :NERDTree <CR>
nnoremap <c-n> :NERDTreeToggle <CR>
```
Source: https://www.youtube.com/watch?v=XBlKG2LW6p4
---
##### Fix the auto complete issue: ~/Appdata/Local/nvim-data/plugged/coc.nvim
Source: https://github.com/neoclide/coc.nvim/issues/3258
> cd ~/Appdata/Local/nvim-data/plugged/coc.nvim
> yarn install
##### Bash Aliases
> nvim ~/.bash_profile
Source 2: https://www.youtube.com/watch?v=XBlKG2LW6p4
---
#### Close terminal or pane
Click on the panel and run :close
or quit vim: :qa
---
##### Practice with vim game
> vimtutor
---
#### Fixing the issue
```
:!node app.js
/usr/bin/bash: /s: No such file or directory
shell returned 127
```
Solution: https://stackoverflow.com/questions/74389900/unable-to-run-commands-with-neovim-when-launched-from-git-bash
Just add the line to your init.vim
> set shellcmdflag=-c
---
##### Split window
> :sp
---
#### Split terminal horizontally
:split term://$SHELL
---
##### Refresh tree after creating folder or a new file
> :NERDTreeRefresh
or set it to refresh automatically, add this into your init.vim file
> autocmd VimEnter * NERDTree
or
simply hit r to refresh the current directory's listing or R to refresh the root directory's listing .
---
#### Prettier configuration
Create a new file called coc-settings.json and paste the following files
```
{
"eslint.autoFixOnSave": true,
"eslint.filetypes": ["javascript", "javascriptreact", "typescript", "typescriptreact"],
"coc.preferences.formatOnSaveFiletypes": [
"javascript",
"javascriptreact",
"typescript",
"typescriptreact"
],
"tsserver.formatOnType": true,
"coc.preferences.formatOnType": true,
"eslint.exclude": [
"**/readme.md"
],
"snippets.ultisnips.pythonPrompt": false
}
" auto Save
" Enable auto-save
autocmd InsertLeave,FocusLost * silent! write
" Disable auto-save for specific file types
autocmd FileType gitcommit autocmd! InsertLeave,FocusLost
" Visual feedback for auto-save
autocmd BufWritePost * if &ft !~# 'commit' | echo "File saved" | endif
```
---
#### Remap your CapsLock to esc key
```
setxkbmap -option caps:escape
```
---
#### go to definition
```
gd: Go to definition
gy: Go to type definition
gr: Find references
[g: Go to the previous diagnostic (error, warning, etc.)
]g: Go to the next diagnostic
<space>s: Open the symbol list
<space>d: Open the diagnostics list
<leader>do: Perform code actions
<leader>rn: Rename symbol
```
```
:CocInstall coc-python
:CocInstall coc-typescript
:CocInstall coc-golang
```
locate your airline and remove this line: C:\Users\user\AppData\Local\nvim-data\plugged\vim-airline\plugin
call airline#extensions#load()
Windows:
:split or :vsp: Split the window horizontally.
:vsplit or :sp: Split the window vertically.
<Ctrl-w> <arrow key>: Switch between windows.
<Ctrl-w> +/-: Increase or decrease the window size.
Tackling: E21: Cannot make changes, 'modifiable' is off
`:set noreadonly`
or
autocmd BufReadPost * if &readonly | set noreadonly | endif
##### Navigation
Search and Find:
- Press / to enter search mode and type a pattern to search forward.
- Use ? to search backward.
- Press n to move to the next search match and N to move to the previous match.
##### Buffer Navigation:
- Use :ls to list all open buffers.
- Press :b followed by a buffer number or part of a buffer name to switch to a specific buffer.
- Use :bnext or :bn to move to the next buffer and :bprevious or :bp to move to the previous buffer.
##### Nerdtree preview
```bash
?
```
Top comments (0)