Introduction
In today's globalized world, it's crucial for businesses to reach a diverse audience. This is where localization comes into play. Localization involves adapting a product or application to a specific market or region, including translating the content, adapting design elements, and considering cultural nuances. For developers working with React and Next.js, there are various techniques and tools available to simplify the process of localizing their applications. In this ultimate guide, we will explore everything you need to know about localizing your React/Next.js app.
Understanding Next.js Internationalization (i18n)
Next.js is a popular open-source framework built on top of React that offers an out-of-the-box solution for server-side rendering (SSR) and static site generation (SSG). It also provides a solid foundation for internationalization (i18n) that complements well with existing i18n libraries. Internationalization is the process of designing and developing a software application to be adapted and translated into multiple languages and cultures. Next.js simplifies the implementation of i18n features such as translations and routing by offering built-in support.
Getting Started: Creating a New Next.js Project
To begin localizing your React/Next.js app, you need to create a new Next.js project. The process is straightforward using the create-next-app CLI tool. Open your terminal and run the following command:
npx create-next-app nextjs-i18n-example
This command will create a new Next.js project called nextjs-i18n-example in a folder with the same name.
Adding React Intl Dependency
Next.js works seamlessly with various i18n libraries such as react-intl, lingui, and next-intl. In this guide, we will focus on using the react-intl library. React-intl is a popular i18n library that supports ICU syntax and provides formatting options. To add react-intl to your Next.js project, navigate to the project folder using your terminal and run the following commands:
cd nextjs-i18n-example
npm i react-intl
These commands will change the current working directory to nextjs-i18n-example and install the react-intl dependency.
Configuring Internationalized Routing
Translations and routing are two essential aspects of internationalization. Next.js offers built-in support for internationalized routing, which simplifies the process of handling different locales in your application. Next.js supports two types of internationalized routing: sub-path routing and domain routing.
Sub-Path Routing
Sub-path routing involves adding the locale to the URL path. For example, /blog represents the default locale, /fr/blog represents the French locale, and /nl-NL/blog represents the Dutch (Netherlands) locale.
Domain Routing
Domain routing uses different domains for serving content in different locales. For example, example.com/blog serves content in the default locale, example.fr/blog serves content in the French locale, and example.nl/blog serves content in the Dutch (Netherlands) locale.
In our case, we will use sub-path routing as it is less complex and more commonly used for average websites. To configure sub-path routing in your Next.js app, you need to update the next.config.js file with the i18n config. Open the next.config.js file and add the following code:
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
i18n: {
locales: ["ar", "en", "fr", "nl-NL"],
defaultLocale: "en",
},
};
module.exports = nextConfig;
In this code, locales represents the list of locales supported in your app, and defaultLocale represents the default locale. This means that all pages for Arabic, French, and Dutch (Netherlands) will be prefixed with ar, fr, and nl-NL in the URL path.
Automatic Locale Detection
Next.js offers automatic locale detection, which determines the user's preferred locale based on the Accept-Language header. This feature simplifies the process of handling user preferences for language and ensures that the app is displayed in the appropriate language by default.
Leveraging the Power of Next-i18next
Next-i18next is a powerful library that complements Next.js by providing translation management and functionality. While Next.js handles the localization of URLs and locales, Next-i18next takes care of translating content, managing translation files, and providing translation components/hooks for React components.
Next-i18next utilizes i18next and react-i18next under the hood, offering a simplified approach to internationalization in Next.js apps. Developers only need to include their translation content as JSON files and let Next-i18next handle the rest.
To use Next-i18next in your React/Next.js app, follow the steps below:
Step 1: Installation
Before you can start using Next-i18next, you need to install it along with the required dependencies. Open your terminal and run the following command:
yarn add next-i18next react-i18next i18next
Ensure that you have react and next installed as well.
Step 2: Configuration
Next-i18next requires a configuration file to define its behavior. Create a file named next-i18next.config.js in the root of your project and add the following code:
module.exports = {
i18n: {
defaultLocale: "en",
locales: ["en", "fr", "de"],
},
};
In this configuration file, you can set the default locale and list all the locales supported by your app.
Step 3: Wrapping Your App
To enable translation support in your Next.js app, you need to wrap your app with the appWithTranslation higher-order component provided by Next-i18next. Open the pages/_app.js file and modify it as follows:
tsx```
import { appWithTranslation } from "next-i18next";
function MyApp({ Component, pageProps }) {
// Your app component code here
}
export default appWithTranslation(MyApp);
Now your app is ready to be translated using Next-i18next.
### Step 4: Translating Your Content
Next-i18next provides hooks and components that make it easy to translate your React components. The main hook you'll use is the useTranslation hook, which gives you access to the t function for translating your content. Here's an example of how to use the useTranslation hook:
import { useTranslation } from "next-i18next";
function MyComponent() {
const { t } = useTranslation();
return
{t("hello")};}
In this example, the t function is used to translate the string "hello". The translation is sourced from the JSON files you provide.
With Next-i18next, you can easily translate your React components and manage your translation content.
## Best Practices for Localizing Your React/Next.js App
When localizing your React/Next.js app, there are several best practices you should follow to ensure a smooth and effective localization process. Let's explore some of these best practices:
### 1. Plan Ahead
Before starting the localization process, it's essential to plan ahead and identify the target languages and regions for your app. This will help you determine the scope of the localization effort and allocate resources accordingly.
### 2. Separate Content from Code
To facilitate the translation process, it's important to separate content from code. Keep all translatable text in separate JSON or CSV files, making it easier for translators to work with the content.
### 3. Use Contextual English
When writing content that will be translated, use contextual English that can be easily understood by translators. Avoid ambiguous or idiomatic language that may be difficult to translate accurately.
### 4. Use Translation Keys
To maintain consistency and facilitate translation updates, use translation keys instead of hardcoding translated text directly into your code. Translation keys act as placeholders for the actual translated content and can be easily updated without modifying the code.
### 5. Test and Validate Translations
Once the translation process is complete, thoroughly test and validate the translated content in your app. Check for any text truncation, layout issues, or missing translations. This step is crucial to ensure a high-quality localized experience for your users.
## Automating Localization with DevTranslate.app
Localizing a React/Next.js app can be a time-consuming and complex task. However, with the help of automated translation tools like DevTranslate.app, you can streamline the localization process significantly. [DevTranslate.app](https://devtranslate.app/) is an automatic translation tool that offers seamless integration with React apps. It simplifies the process of translating your app's content by automatically detecting translatable strings and providing translations in multiple languages. Integrating DevTranslate.app into your localization workflow can save you valuable time and resources.
## Go global
Localizing your React/Next.js app is essential to reach a global audience and provide a personalized experience to users in different languages and regions. By following the best practices and utilizing the right tools, you can simplify the localization process and create a successful internationalized application. Next.js, combined with libraries like Next-i18next, provides a solid foundation for implementing localization features. With the help of automated translation tools like DevTranslate.app, you can further streamline the localization process and enhance the efficiency of your development workflow. Embrace localization and unlock the full potential of your React/Next.js app in the global market.
---
## Speed up your apps & websites translation
**DevTranslate** online JSON, XML, STRINGS & ARB files translator app for developers, product managers, content creators. Use automated multi language translation instead of wasting time copy-pasting all your text into online translators. **[Try DevTranslate for free!](https://devtranslate.app)**
[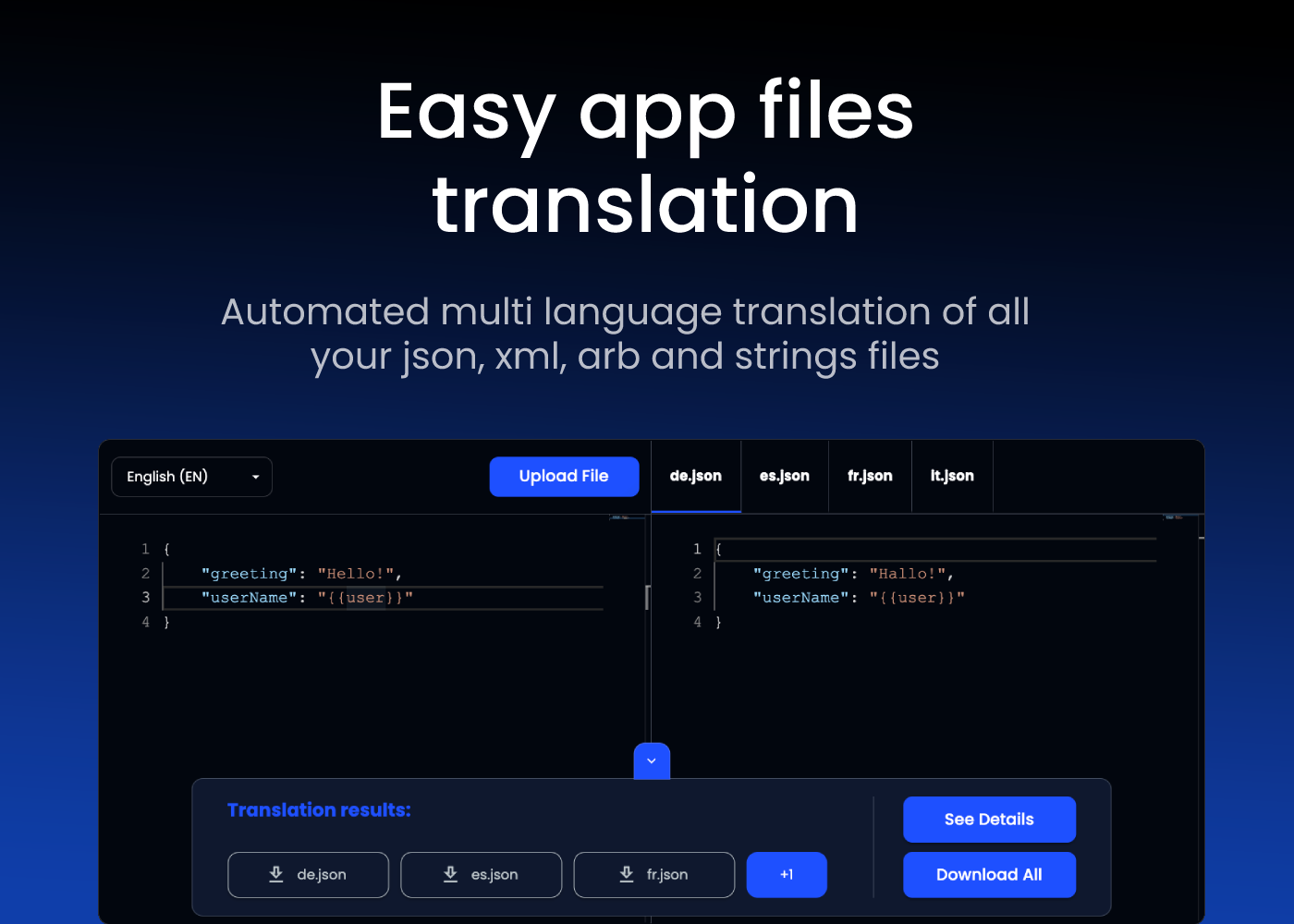](https://devtranslate.app)
Top comments (1)
Be mindful that's even if it's true for the Pages Router architecture of nextjs, it's now obsolete for the App Router architecture.