TL;DR
These days, any dev can build powerful things with AI.
No need to be a ML expert.
Here are the 7 best libraries you can use to supercharge your development and to impress users with state-of-the-art AI features.
These can give your project magical powers, so DON'T FORGET TO STAR & SUPPORT THEM π
1. CopilotTextarea - AI-powered Writing in React Apps
A drop-in replacement for any react <textarea>
with the features of Github CopilotX.
Autocompletes, insertions, edits.
Can be fed any context in real time or by the developer ahead of time.
import { CopilotTextarea } from "@copilotkit/react-textarea";
import { CopilotProvider } from "@copilotkit/react-core";
// Provide context...
useMakeCopilotReadable(...)
// in your component...
<CopilotProvider>
<CopilotTextarea/>
</CopilotProvider>`
```
Star CopilotTextarea βοΈ
---
## 2. Tavily GPT Researcher - Get an LLM to Search the Web & Databases
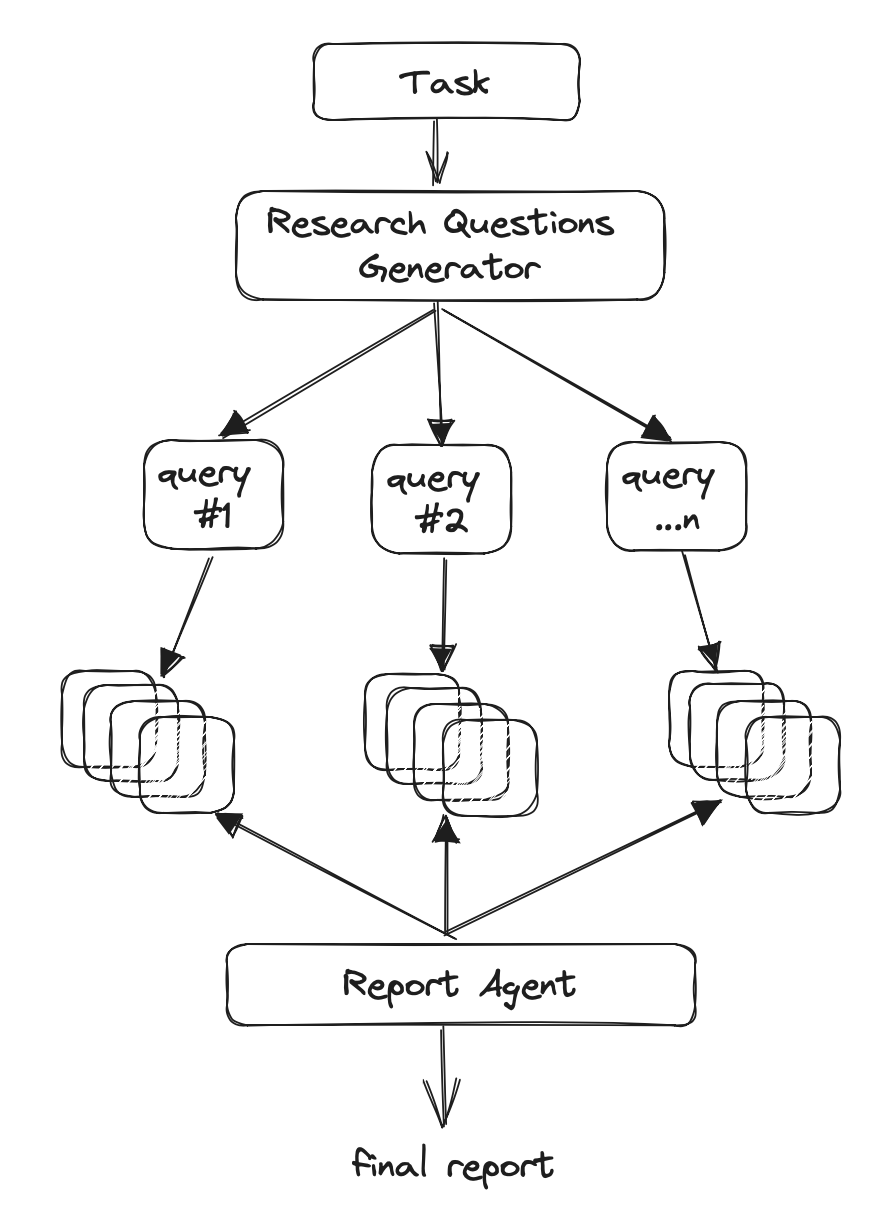
Tavily enables you to add GPT-powered research and content generation tools to your React applications, enhancing their data processing and content creation capabilities.
```tsx
# Create an assistant
assistant = client.beta.assistants.create(
instructions=assistant_prompt_instruction,
model="gpt-4-1106-preview",
tools=[{
"type": "function",
"function": {
"name": "tavily_search",
"description": "Get information on recent events from the web.",
"parameters": {
"type": "object",
"properties": {
"query": {"type": "string", "description": "The search query to use. For example: 'Latest news on Nvidia stock performance'"},
},
"required": ["query"]
}
}
}]
)
```
Star Tavily βοΈ
---
## 3. Pezzo.ai - Observability, Cost & Prompt Engineering Platform
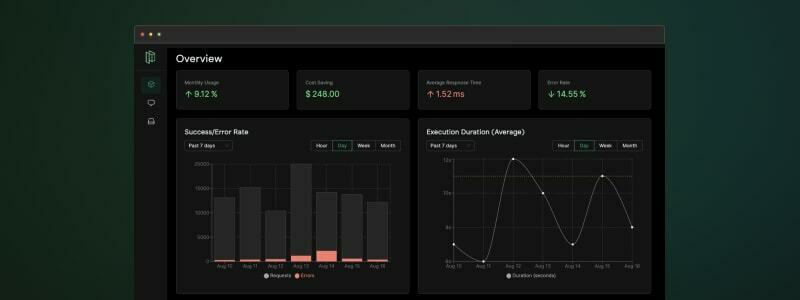
Centralized platform for managing your OpenAI calls.
Optimize your prompts & token use. Keep track of your AI use.
Free & easy to integrate.
```tsx
const prompt = await pezzo.getPrompt("AnalyzeSentiment");
const response = await openai.chat.completions.create(prompt);
```
Star Pezzo βοΈ
---
## 4. [CopilotPortal](https://github.com/RecursivelyAI/CopilotKit): Embed an actionable LLM chatbot inside your app.
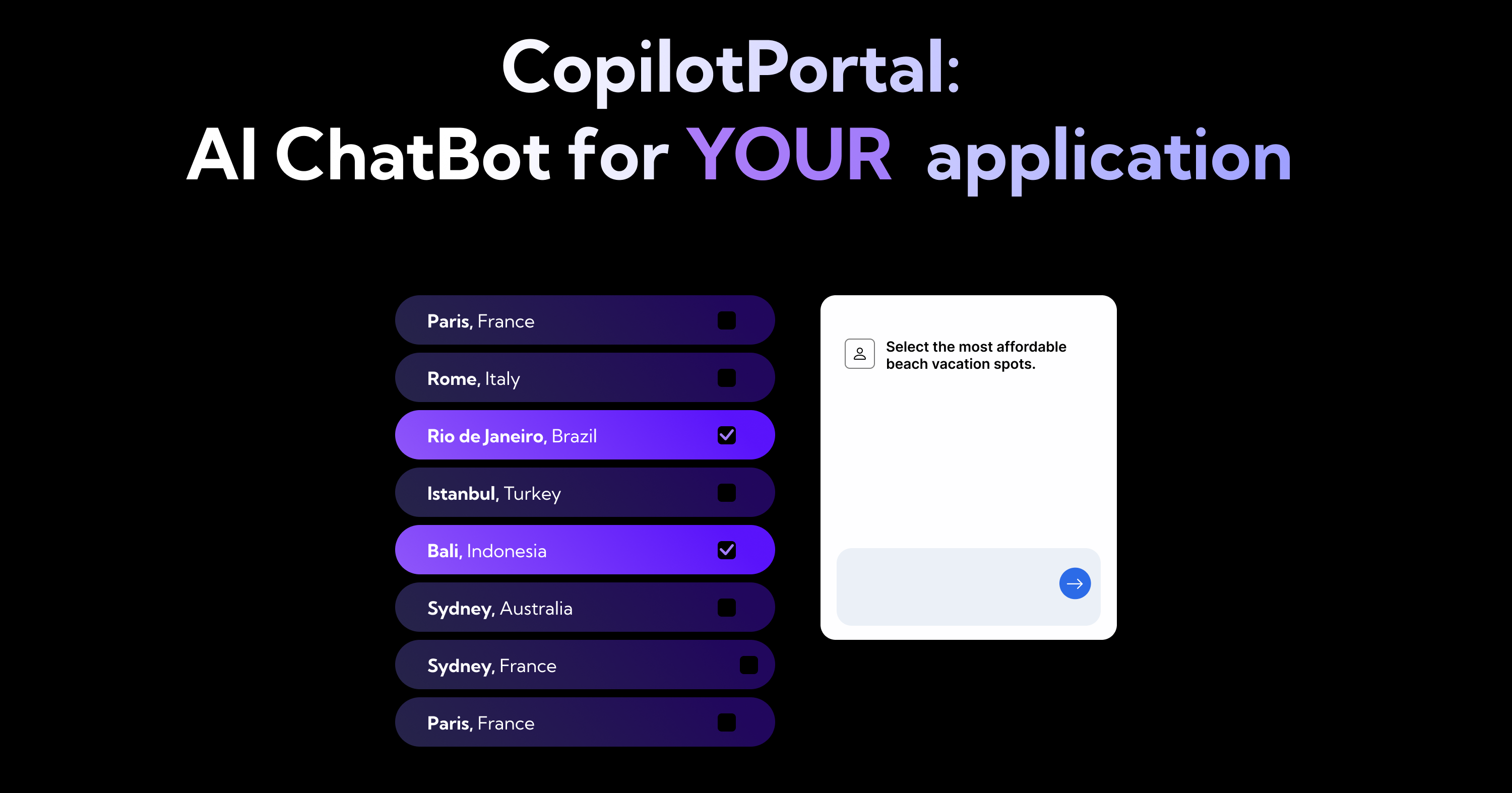
A context-aware LLM chatbot inside your application that answers questions and takes actions.
Get a working chatBot with a few lines of code, then customize and embed as deeply as you need to.
```tsx
import "@copilotkit/react-ui/styles.css";
import { CopilotProvider } from "@copilotkit/react-core";
import { CopilotSidebarUIProvider } from "@copilotkit/react-ui";
export default function App(): JSX.Element {
return (
<CopilotProvider chatApiEndpoint="/api/copilotkit/chat">
<CopilotSidebarUIProvider>
<YourContent />
</CopilotSidebarUIProvider>
</CopilotProvider>
);
}
```
Star CopilotPortal βοΈ
---
## 5. LangChain - Pull together AIs into action chains.
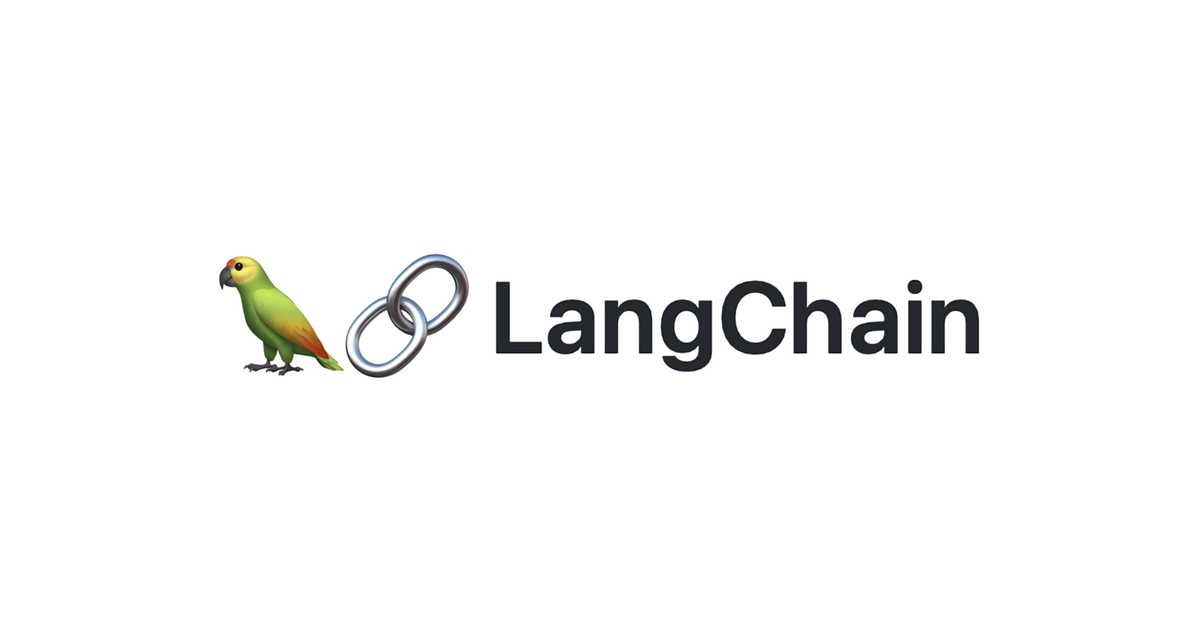
Easy-to-use API and library for adding LLMs into apps.
Link together different AI components and models.
Easily embed context and semantic data for powerful integrations.
```tsx
from langchain.llms import OpenAI
from langchain import PromptTemplate
llm = OpenAI(model_name="text-davinci-003", openai_api_key="YourAPIKey") # Notice "food" below, that is a placeholder for another value later
template = """ I really want to eat {food}. How much should I eat? Respond in one short sentence """
prompt = PromptTemplate(
input_variables=["food"],
template=template,
)
final_prompt = prompt.format(food="Chicken")
print(f"Final Prompt: {final_prompt}")
print("-----------")
print(f"LLM Output: {llm(final_prompt)}")
```
Star LangChain βοΈ
---
## 6. [Weaviate](https://github.com/weaviate/weaviate) - Vector Database for AI-Enhanced Projects
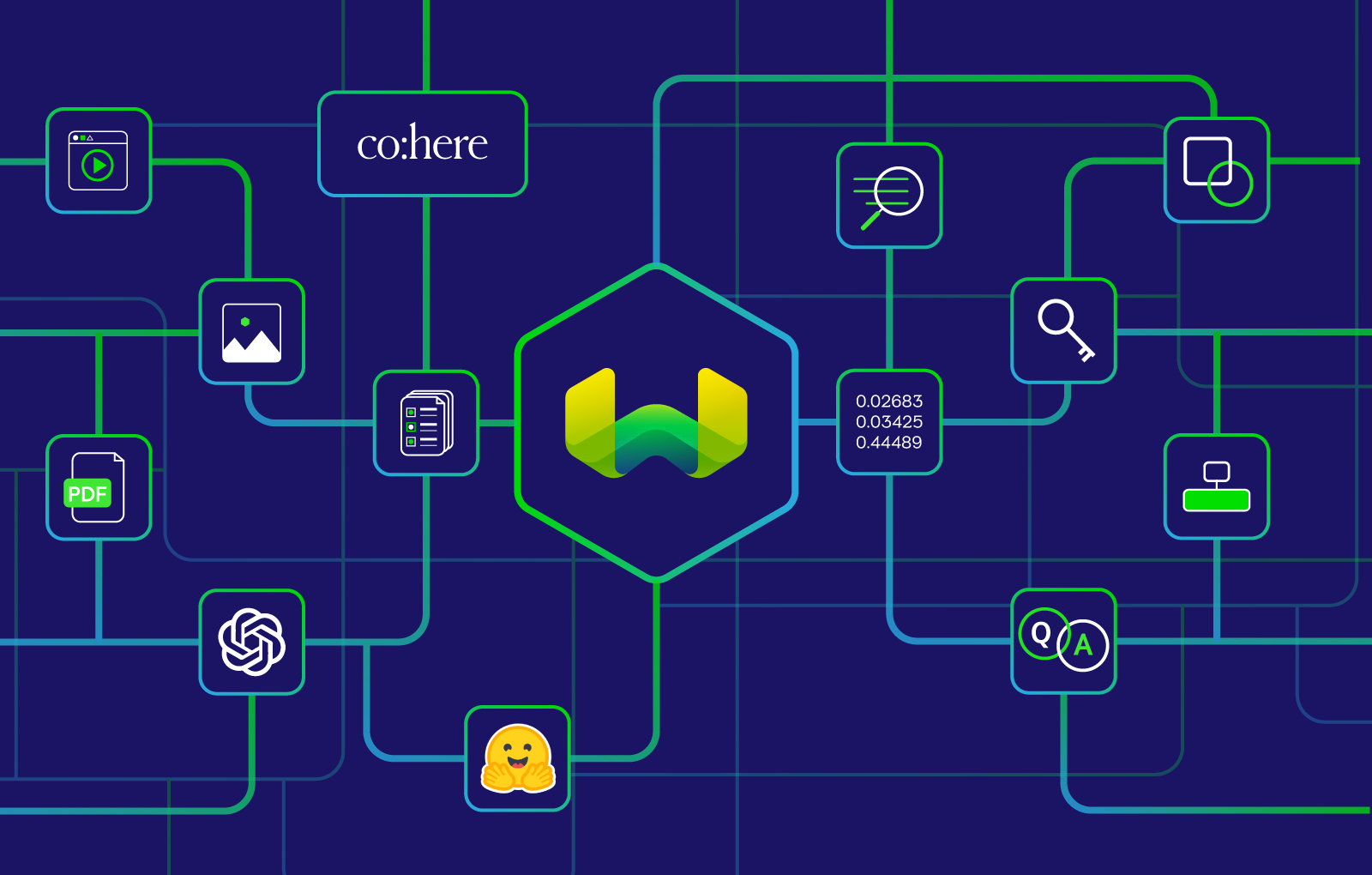
Weaviate is a vector database optimized for fast, efficient searches across large datasets.
It supports integration with AI models and services from providers like OpenAI and Hugging Face, enabling advanced tasks such as data classification and natural language processing.
It's a cloud-native solution and highly scalable to meet evolving data demands.
```tsx
import weaviate
import json
client = weaviate.Client(
embedded_options=weaviate.embedded.EmbeddedOptions(),
)
uuid = client.data_object.create({
})
obj = client.data_object.get_by_id(uuid, class_name='MyClass')
print(json.dumps(obj, indent=2))
```
Star Weaviate βοΈ
---
## 7. [PrivateGPT](https://github.com/imartinez/privateGPT) - Chat with your Docs, 100% privately π‘
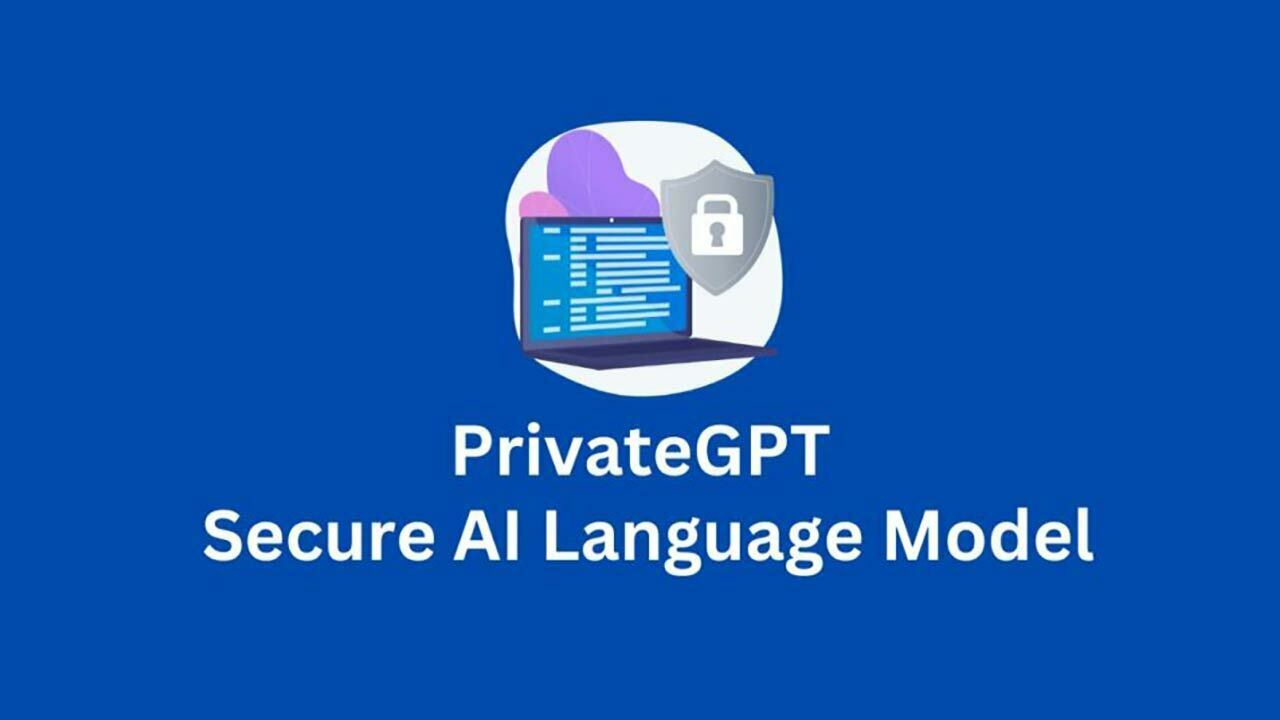
PrivateGPT allows for secure, GPT-driven document interaction within your applications, ensuring data privacy and enhancing context-aware processing capabilities.
PrivateGPT ensures privacy by locally processing and storing documents and context, without sending data to external servers.
```tsx
from privategpt import PrivateGPT, DocumentIngestion, ChatCompletion
client = PrivateGPT(api_key='your_api_key')
def process_documents_and_chat(query, documents):
ingestion_result = DocumentIngestion(client, documents)
chat_result = ChatCompletion(client, query, context=ingestion_result.context)
return chat_result
documents = ['doc1.txt', 'doc2.txt']
query = "What is the summary of the documents?"
result = process_documents_and_chat(query, documents)
print(result)
```
Star PrivateGPT βοΈ
---
## [Bonus Library]. SwirlSearch - AI powered search.
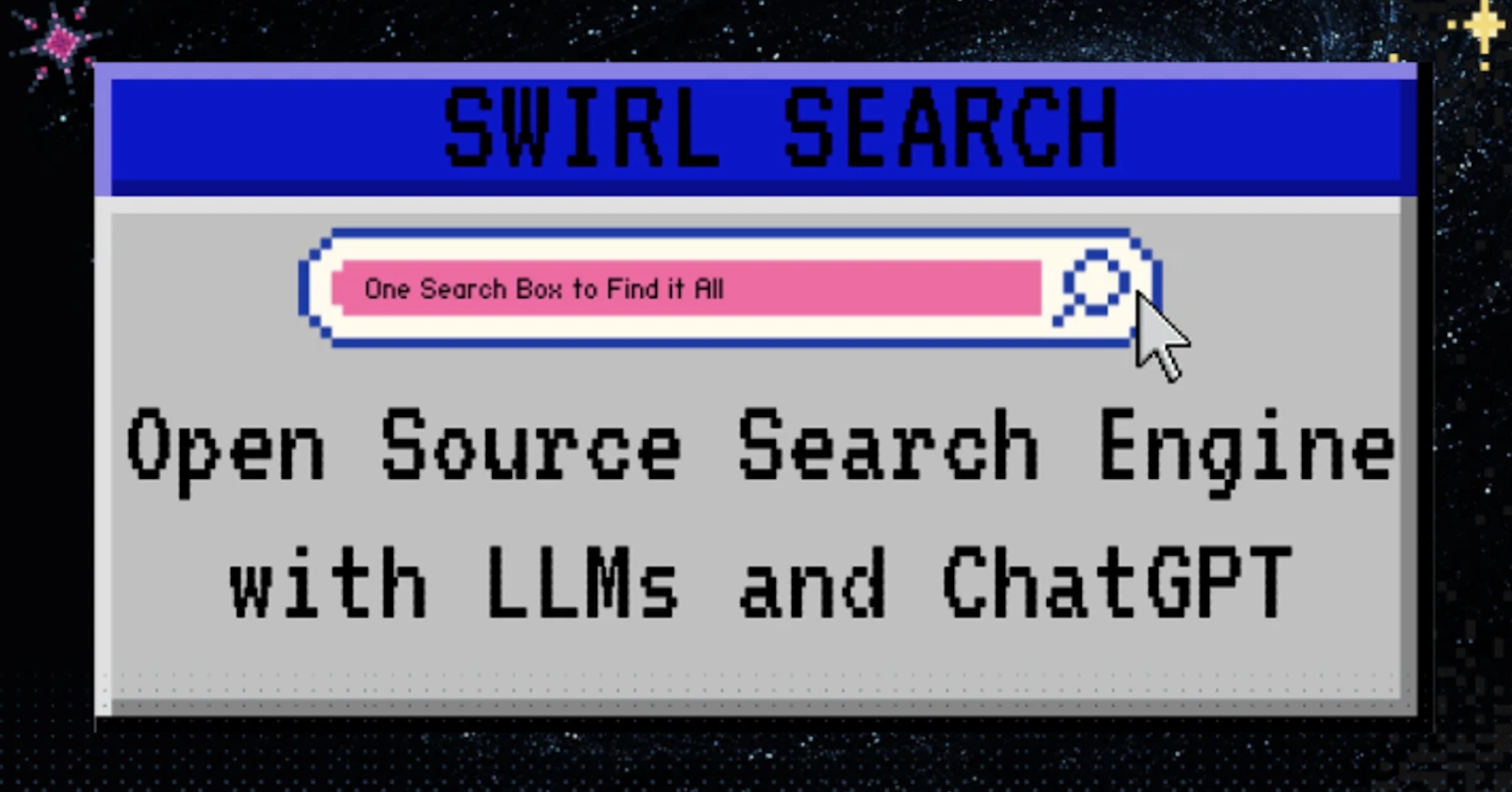
LLM-powered search, summaries and output.
Searches multiple content sources simultaneously into integrated output.
Powerful for tailored in-app integrations of various data sources.
Star SwirlSearch βοΈ
---
Thanks for reading!
I hope these help you build some awesome stuff with AI.
Please like if you enjoyed & comment any other libraries or topics you would like to see.
Top comments (24)
Hey people π
Hope you enjoyed the article! Don't forget to give it some reaction for support.
Let me know if there are any libraries you wish I included & what you'd like to see written about next!
Cheers πΎπ«‘
Hmm⦠I think this is a case of a good listicle! I gave it a like and bookmark!
That is an excellent list. I love all the projects. π
Awesome Open Source π₯
Yes! These projects are all incredible and Open Source is the way!
CopilotKit is interesting!
What can I do with it?
Thanks for the question,
It's a library for integrating AI into react apps.
You can:
Easily insert a GPT chatBot that takes actions & answers questions
Turn any into an AI-powered
Lmk if you have any more Q's about it π©βπΎ
github.com/RecursivelyAI/CopilotKit
Great list!
Thank you for sharing!
Thanks for checking it out π¬
Cool list. Thanks for sharing. Personally, I prefer Quivr over PrivateGPT. Otherwise great list.
I think a lot of these can work together well π
Very cool list!
Yup! Understated point.
The kinds of projects that even junior devs can build by mixing these libraries is hard to grasp.
Nice repos! Thanks for sharing :D
Thanks for reading!
Great compilation, thanks for sharing!
Thanks for checking it out :)
CopilotKit looks pretty nice!
She is very nice π
Nice to see Swirl in the list :)
It's a great project π¬