In the ever-evolving landscape of software development, the significance of testing cannot be overstated. It plays a pivotal role in guaranteeing the dependability and functionality of applications. Uncovering and resolving bugs in the early stages of development not only conserves time and resources but also elevates the overall quality of the software.
Enter Vitest, a robust testing framework that stands out as a compelling alternative to well-known tools like Jest, particularly for Vue.js projects. Fueled by Vite, Vitest distinguishes itself with its exceptional speed and simplicity, making it an invaluable resource for developers seeking to streamline their testing processes.
In this article, we will walk you through the basics Vitest and testing in your projects using Vitest framework.
Getting Started
Table of contents
- What is Vite
- What is Vitest
- Why Vitest
- Vitest vs other frameworks
- Create your first vitest app
- Create a React project with Vitest
- Installing the dependencies
- Writing your first test script
- Running the test
What is Vite
Vite stands out as a cutting-edge, high-speed tool designed for scaffolding and constructing web projects. It is crafted by Evan You, the mind behind Vue.js. Vite supports a range of frameworks, including Vue, React, Preact, Lit, Svelte, and Solid. Its key strength lies in leveraging native ES modules, resulting in superior speed compared to conventional tools like webpack or Parcel.
Vite employs a server that dynamically compiles and serves necessary dependencies through ES modules. This strategy enables Vite to process and deliver only the code essential at any given moment. Consequently, Vite deals with considerably less code during server startup and updates. Another factor contributing to Vite’s speed is its utilization of esbuild for pre-bundling dependencies in development. Esbuild, a remarkably fast JavaScript bundler implemented in the Go language, enhances the overall performance of Vite.
What is Vitest
Vitest, a testing framework, is constructed atop Vite, a tool dedicated to overseeing and constructing JavaScript-centric web applications. It stands out as a swift and minimalist testing solution, demanding minimal configuration. Vitest seamlessly aligns with Jest, a widely adopted JavaScript testing framework, and seamlessly integrates into Vue applications. While it is purpose-built for use with Vite, Vitest can also operate independently, offering flexibility in its application.
Why Vitest
Vitest stands out as a powerful testing framework that offering unique advantages over other frameworks like Jest. It provides the following features which makes it attractive towards the testing frameworks.
Simplified Setup and Configuration:
ViTest streamlines the setup and configuration process, enabling developers to dedicate more time to writing tests and less to intricate configuration. Its minimalistic approach makes it an ideal choice for small to medium-sized projects.
Concise and Legible Syntax:
ViTest offers a concise and easily understandable syntax, simplifying the task of writing and comprehending test cases. With its clean and intuitive API, you can articulate your test expectations in a natural, human-readable manner.
Outstanding Performance and Test Execution:
ViTest is renowned for its exceptional performance and rapid test execution speed. It optimizes test execution, ensuring the efficiency of your test suite, even as your codebase expands. This advantage proves especially valuable when tackling extensive test suites or large-scale projects.
Seamless TypeScript Integration:
ViTest seamlessly integrates with TypeScript, harnessing its type system to detect errors and furnish insightful feedback during testing. You have the ability to define and enforce type validations within your test cases, guaranteeing type correctness throughout your testing journey.
Vitest vs other frameworks
When it comes to testing your JavaScript code, the landscape offers a plethora of options. Two standout choices among the most popular ones are Jest and Vitest.
Jest
Jest is a JavaScript testing framework that is designed to ensure the correctness of any JavaScript codebase. It has gained popularity among developers for its simplicity and user-friendly nature. Jest works with projects using Babel, TypeScript, Node, React, Angular, Vue, and more. It is a zero-config framework that aims to work out of the box on most JavaScript projects. Jest provides a wide range of features such as snapshots, isolated tests, great API, fast and safe, code coverage, and easy mocking. Jest is well-documented, requires minimal configuration, and can be extended to meet specific project needs. Widely utilized by companies and individuals globally, it stands as a trusted and extensively adopted tool in the realm of JavaScript development.
Vitest vs Jest
Whether you opt for Jest or Vitest for JavaScript testing, you’ll find a contemporary, straightforward, and speedy testing experience. These frameworks are well-established, work seamlessly.
Speed
The choice between Jest and Vitest for faster tests depends on the specific circumstances. Vitest generally offers a safer bet for faster test execution, but the significance of this advantage varies based on factors such as the number of tests and the available resources. If you have a substantial number of tests and are running them on a resource-constrained local development environment, test speed becomes a more crucial concern. However, if you have only a few tests or are testing on a well-resourced infrastructure, the speed difference may be less critical.
Module management
Jest aligns with CommonJS for module management, offering simplicity in testing for projects using this traditional approach. In contrast, Vitest is tailored for ECMAScript Modules (ESM), a more contemporary module management system. Choosing between Jest and Vitest may hinge on your project’s module strategy; Jest seamlessly integrates with CommonJS, while Vitest is the preferred option for ESM users. The module management system compatibility becomes a pivotal factor in deciding which testing framework aligns with your JavaScript project.
Documentation and community support
Jest having been around for a decade, boasts a larger and more established community than the relatively newer Vitest. This results in better documentation and support for Jest, making it a more accessible choice. While Vitest is gaining popularity, it may take time to match Jest’s community strength. Jest currently enjoys the advantage of a more established ecosystem.
Image credits Vitest: Blazing Fast Unit Test Framework (lo-victoria.com)
Creating your first vitest app
In this section, we will try to create our first vitest application.
- Open command prompt / terminal in your machine.
- Create a folder named
first-vitest-app
and switch to the directory.
mkdir first-vitest-app
- Initialize the project using the following command and provide the necessary details (test command: vitest) when it required. ```
npm init
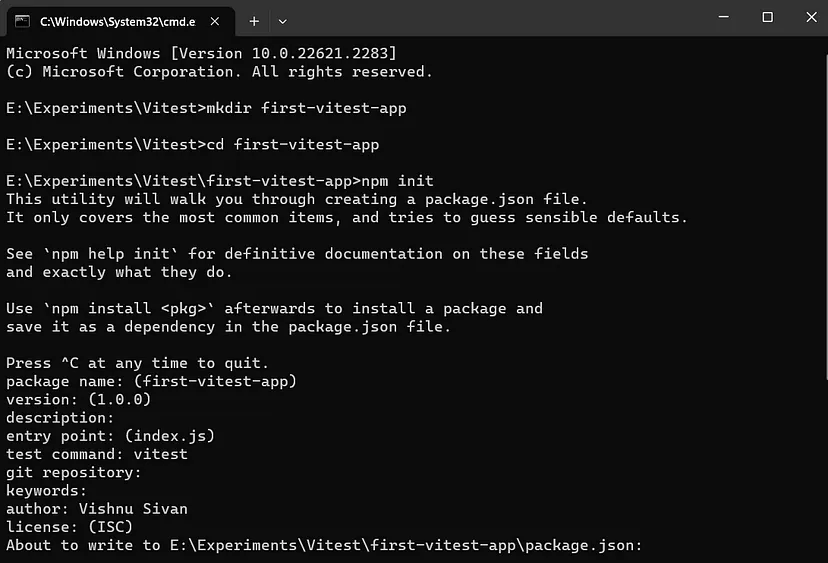
- Install the libraries `vite`, `vitest`, `@vitest/ui` using the following command.
npm install vite vitest @vitest/ui
Vite is the default development dependency for vitest. The vitest/ui provides a user interface for testing the application.
- Add the scripts attribute with the following contents in the `package.json` file.
"scripts": {
"test": "vitest",
"test:ui": "vitest --ui",
"test:run": "vitest run"
}
- Create a file named vite.config.ts and add the following code to it.
///
// Configure Vitest (https://vitest.dev/config/)
import { defineConfig } from 'vite'
export default defineConfig({
test: {
/* for example, use global to avoid globals imports (describe, test, expect): */
// globals: true,
},
})
- Create a folder named `test` and create files named `basic.test.ts` and `suite.test.ts` inside it. `basic.test.ts` file is used to write the basic test cases using `test()` method and `suite.test.ts` file is used to write test cases using `describe()` method.
- Open `basic.test.ts` file and add the following code to it.
import { assert, expect, test } from 'vitest'
test('Math.sqrt()', () => {
expect(Math.sqrt(4)).toBe(2)
expect(Math.sqrt(144)).toBe(12)
expect(Math.sqrt(2)).toBe(Math.SQRT2)
})
test('JSON', () => {
const input = {
foo: 'hello',
bar: 'world',
}
const output = JSON.stringify(input)
expect(output).eq('{"foo":"hello","bar":"world"}')
assert.deepEqual(JSON.parse(output), input, 'matches original')
})
- Open `suite.test.ts` file and add the following code to it.
import { assert, describe, expect, it } from 'vitest'
describe('suite name', () => {
it('foo', () => {
assert.equal(Math.sqrt(4), 2)
})
it('bar', () => {
expect(1 + 1).eq(2)
})
it('snapshot', () => {
expect({ foo: 'bar' }).toMatchSnapshot()
})
})
### Running the test
Run the test using the following commands.
- Run the test in the normal command line mode.
npm run test
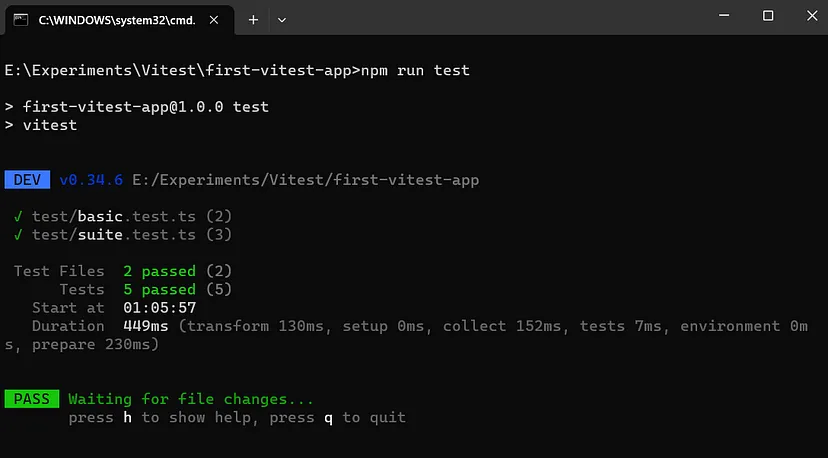
- Run the test with UI.
npm run test:ui
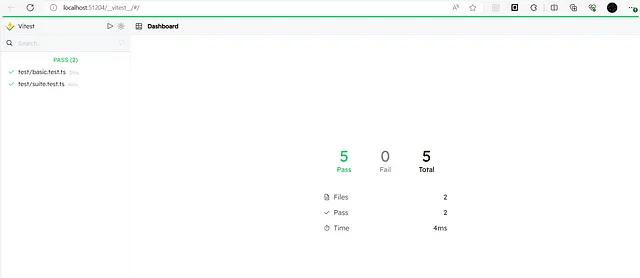
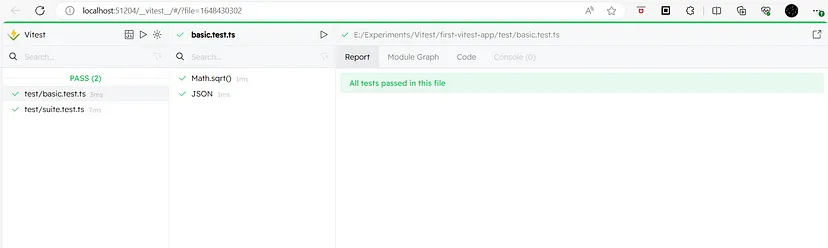
### Creating a React project with Vitest
In this section, we will build a React application utilizing the Vite framework. Additionally, we will develop test cases using the Vitest framework and proceed to execute it.
- Create a react project using vite by executing the following command.
npm create vite@latest
- Switch to the project folder and install the dependencies using the following command.
npm install
#### Installing the dependencies
- Install vitest library using the following command.
npm install -D vitest jsdom @testing-library/react @testing-library/jest-dom @types/testing-library__jest-dom
- Add the scripts attribute with the following contents in the `package.json` file.
"scripts": {
...
"test": "vitest"
}
- Create a folder named `tests` and add a file named `setup.ts` with the following content to it.
///
import { expect, afterEach } from 'vitest';
import { cleanup } from '@testing-library/react';
import * as matchers from '@testing-library/jest-dom/matchers';
expect.extend(matchers);
afterEach(() => {
cleanup();
});
- Open `vite.config.js` file and add the following code to it.
test: {
environment: 'jsdom',
setupFiles: ['./tests/setup.ts'],
testMatch: ['./tests/*/.test.tsx'],
globals: true
}
### Writing your first test script
We have configured vitest for the app. We can create a basic test case to evaluate the App component.
- Create a file named App.test.tsx and add the following code to it.
import { render, screen } from '@testing-library/react';
import { describe, expect, it } from 'vitest'
import App from "../src/App";
import React from 'react';
describe('App', () => {
it('renders headline', () => {
render();
const headline = screen.getByText("Vite + React");
expect(headline).toBeInTheDocument();
});
});
### Running the test
Run the test using the following command.
npm run test
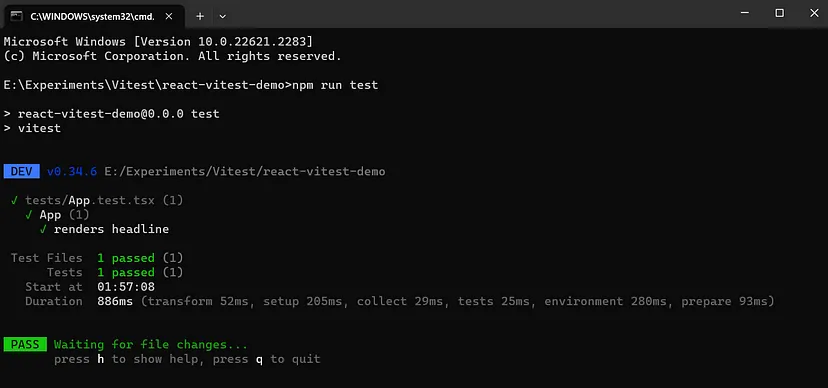
- Change the headline in `App.tsx` file to `“Your first Vitest app”`. Run the test again then you will get the output as follows.
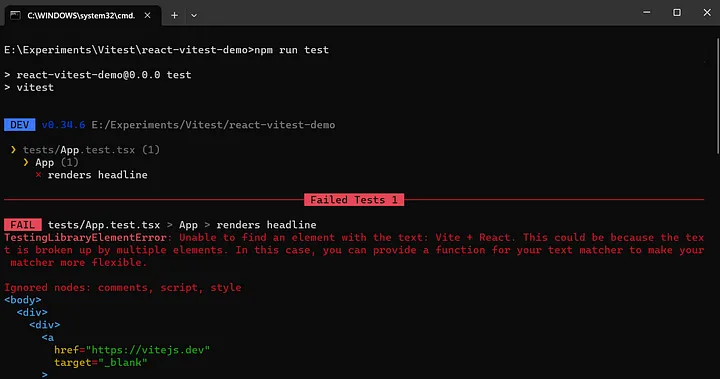
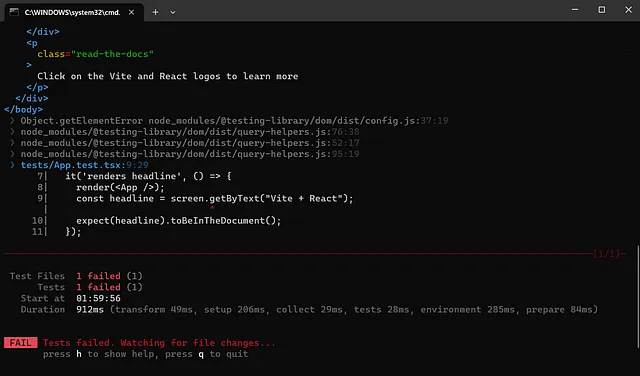
Thanks for reading this article.
Thanks Gowri M Bhatt for reviewing the content.
If you enjoyed this article, please click on the heart button ♥ and share to help others find it!
The full source code for this tutorial can be found here,
[GitHub - codemaker2015/vitest-examples: vitest testing framework examples](https://github.com/codemaker2015/vitest-examples)
The article is also available on [Medium](https://medium.com/@codemaker2016/introducing-vitest-the-super-fast-testing-framework-c4a86b431f8d).
Top comments (0)