Welcome to Part 6 of the COVIDiary project! If you’re just joining us or missed a post, here’s what we’ve done so far:
- Part 1: Project Introduction
- Part 2: Initial Setup
- Part 3: Building the Database
- Part 4: Frontend Setup
- Part 4.5: Database Fixes
- Part 5: Backend Routing
This week, we’re working on the back end. By the end of today, we will:
- Add some important gems
- Generate serializers to properly format our data
Are you ready? Let’s do this.
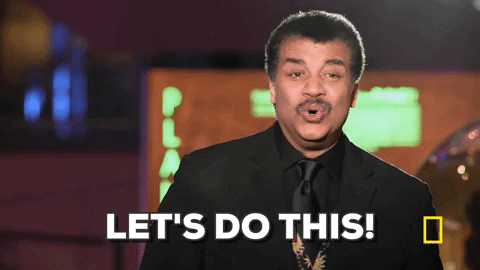
Open your CD-API
repository.
1. Add Gems
We need to add two more gems to our Gemfile
to help the front and back ends communicate properly.
Rack CORS Middleware
The first is Rack CORS Middleware, which will let our fetch requests work. Rack CORS is used so frequently in Rails applications that it’s automatically included in your Gemfile
. To use it, simply uncomment the line in your Gemfile
.
# Use Rack CORS for handling Cross-Origin Resource Sharing (CORS), making cross-origin AJAX possible
gem 'rack-cors'
We also need to uncomment the following section in config/initializers/cors.rb
:
Rails.application.config.middleware.insert_before 0, Rack::Cors do
allow do
origins 'example.com'
resource '*',
headers: :any,
methods: [:get, :post, :put, :patch, :delete, :options, :head]
end
end
Finally, change example.com
to *
for now.
Fast JSON API
The second gem we need to add is Fast JSON API. This will allow us to generate serializers and properly format our data before sending it to the front end. You can read more about serializers here. In your Gemfile
, add the following:
gem ‘fast_jsonapi’
That’s it! Now that we’ve added both gems, let’s install them by running bundle install
in your terminal. You can also start up your backend server now using rails s
.
2. Generate Serializers
We need a serializer for both our Entries
and our Users
.
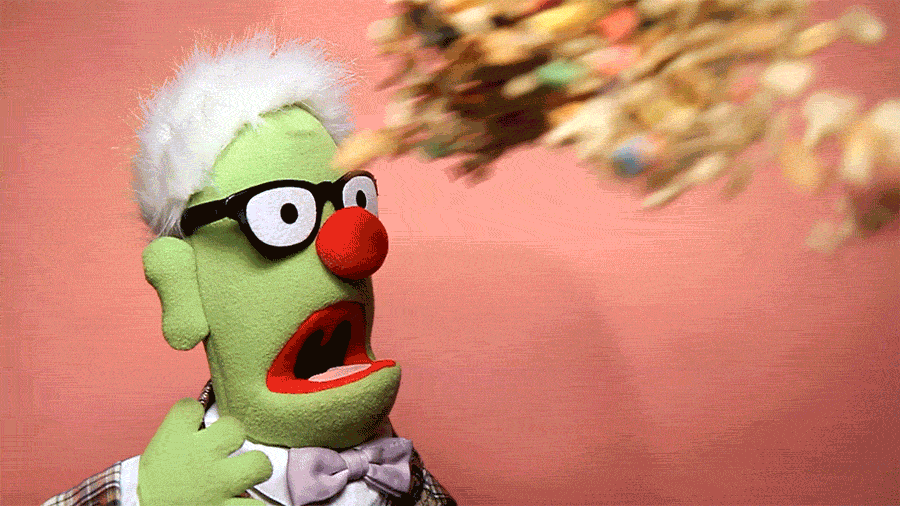
With Fast JSON API, these are easy to create with the rails g serializer
command. Let’s do that now. In your terminal, enter the following:
rails g serializer Entry
rails g serializer User
You will now have a serializers
directory in your app
directory, and it will contain your two new serializer files. They should look something like this:
class EntrySerializer
include FastJsonapi::ObjectSerializer
attributes
end
Let’s configure these to present the data the way we want. First, we’ll add our relationships. A user has many entries, so in your UserSerializer
class, add:
has_many :entries
We’ll set up the opposite side of the relationship in your EntrySerializer
class:
Belongs_to :user
Now that we have the relationships under control, we need to specify which data attributes we want to serialize. In your EntrySerializer
class, change the attributes
line to look like the following:
attributes :id,
:health_rating,
:is_symptomatic,
:health_comments,
:mental_health_rating,
:mental_health_comments,
:diary_entry,
:is_public,
:created_at
Now do the same with the UserSerializer
class:
attributes :id,
:first_name,
:last_name,
:email,
:birth_date,
:occupation,
:is_essential,
:isolation_start,
:isolation_end,
:about
Coming Up
We now have serializers to properly format our data for future fetch()
requests!! Next week, we’ll create our controller actions so we can finally connect to the front end!
Top comments (0)