This post originally appeared on Arjun Rajkumar's blog. Arjun is a web developer based in Bangalore, India.
--
I'm going to spend 1-2 hours/day solving different algorithms. More about this here.
Day 1: Question 1
Write an efficient method that takes stock_prices and returns the best profit you could have made from one purchase and one sale of one share of Apple stock yesterday.
For example:
stock_prices = [10, 7, 5, 8, 11, 9]
get_max_profit(stock_prices)
# returns 6 (buying for $5 and selling for $11)
No "shorting"—you need to buy before you can sell. Also, you can't buy and sell in the same time step—at least 1 minute has to pass.
If you want to follow along, feel free to post your answers below.
Top comments (2)
This was my logic for doing this.
Logic:
It works with these few example cases:
Examples: [10, 7, 5, 8, 11, 9] -> 6 ; [10, 7, 100, 8, 11, 9] -> 92 ; [10] -> 0 ; [10, 2] -> 0.
Code:
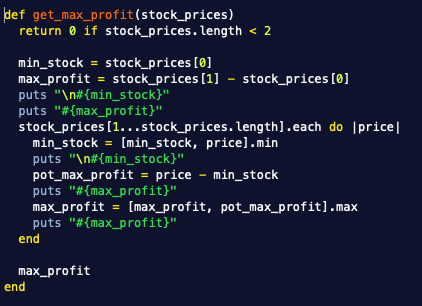
Github
In ruby